Hello Guys 👋 In this post, We will learn how to make Number Guessing Game JavaScript. In the Number guessing game basically, we have to guess a number between 1 to 100. When we enter any number between 1 to 100. If a guessed number is higher than the actual number then show a message YOUR GUESS IS TOO HIGH and if a number is lower then show a message YOUR GUESS IS TOO LOW. If we number is correct then show a message YOU GUESSED CORRECTLY! and the score is increased and also we have 5 lifeLine in this game. If lifeLine is zero then the game is over. Also, we store high scores in local storage.
In this project, we will use HTML, CSS, and basic Vanilla Javascript. In this project, you will learn new things like local storage, DOM manipulation, etc. This project our main focus is javascript.
You can see the Demo Here Number Guessing Game JavaScript
👉 Create a 📂index.html
Here we are making attractive UI
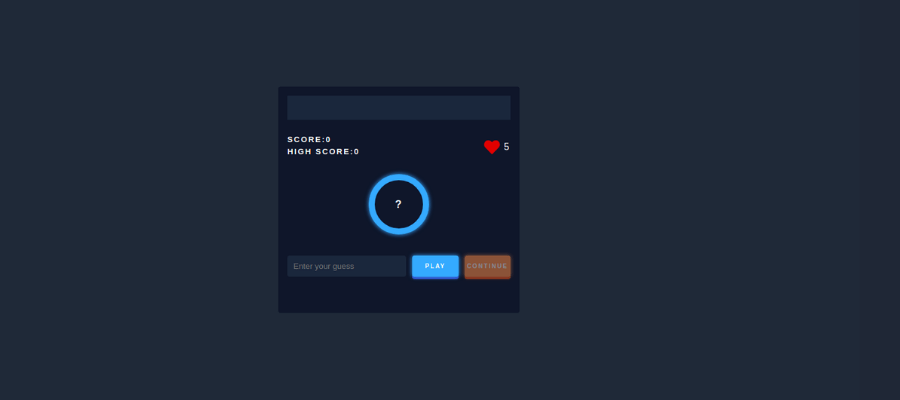
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Number Guessing Game in JavaScript</title>
<link rel="stylesheet" href="style.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.10.0/css/all.min.css" integrity="sha512-PgQMlq+nqFLV4ylk1gwUOgm6CtIIXkKwaIHp/PAIWHzig/lKZSEGKEysh0TCVbHJXCLN7WetD8TFecIky75ZfQ==" crossorigin="anonymous" referrerpolicy="no-referrer" />
</head>
<body>
<div class="game-wrapper">
<div class="game-data">
<h5 class="feedback-label"></h5>
<div class="score-data">
<h5 class="score-label">Score:<span class="score"> 0</span></h5>
<h5 class="score-label">High Score:<span class="high-score"> 0</span></h5>
</div>
<div class="lives-data">
<i class="fas fa-heart heart"></i>
<span class="lives-count"> 5</span>
</div>
<div class="hidden-number-wrapper">
<div class="hidden-number-wrapper-inner">
<h5 class="hidden-number-label">?</h5>
</div>
</div>
</div>
<div class="game-controls">
<input type="text" class="guess" placeholder="Enter your guess">
<button class="btn btn-blue play">Play</button>
<button class="btn btn-orange continue" disabled>Continue</button>
</div>
<div class="numbers-guess">
<h5> Guessed numbers are: <span class="guessed-number"></span></h5>
</div>
</div>
<div class="modal">
<h5 class="game-over">Game over</h5>
<p>Would you like to continue?</p>
<button class="btn btn-blue retry">Retry</button>
</div>
<!-- Scripts -->
<script src="index.js"></script>
</body>
</html>
👉 Now Create a 📂style.css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
html {
font-size: 62.5%;
}
body {
font-family: sans-serif;
background-color: #212a37;
color: #fff;
font-size: 1.6rem;
height: 100vh;
display: grid;
place-content: center;
letter-spacing: 2px;
text-transform: uppercase;
}
span {
display: inline-block;
}
.game-wrapper {
background-color: #101829;
width: 40rem;
border-radius: 4px;
padding: 1.5rem;
}
.game-data {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 2.5rem 1rem;
}
.feedback-label {
background-color: #1d293b;
padding: 2rem 1rem;
grid-column: 1/-1;
text-align: center;
line-height: 0;
}
.score-data {
grid-column: 1/3;
display: flex;
flex-direction: column;
}
.score-label {
margin-bottom: 0.5rem;
}
.lives-data {
grid-column: 3/-1;
display: flex;
align-items: center;
justify-content: flex-end;
}
.heart {
font-size: 2.6rem;
margin-right: 0.5rem;
color: #c92626;
}
.hidden-number-wrapper {
display: flex;
grid-column: 1/-1;
padding: 0 0 2.5rem;
}
.hidden-number-wrapper-inner {
width: 10rem;
height: 10rem;
border: 1rem solid #5bb1fc;
border-radius: 50%;
display: flex;
margin: auto;
box-shadow: 0 0 0.7rem #5bb1fc;
}
.hidden-number-label {
margin: auto;
font-size: 1.8rem;
}
.game-controls {
display: flex;
padding: 1rem 0;
}
.game-controls > *:not(:last-child) {
margin-right: 1rem;
}
.guess {
border-radius: 4px;
border: none;
outline: none;
background-color: #1d293b;
color: #fff;
padding: 1rem;
}
.btn {
border: none;
border-radius: 4px;
color: #fff;
width: 100%;
height: 3.5rem;
cursor: pointer;
text-transform: uppercase;
font-weight: bold;
letter-spacing: 2px;
font-size: 1rem;
}
.btn.btn-blue {
background-color: #5bb1fc;
box-shadow: 0 0 0.7rem #5bb1fc, 0 4px #2d57bb;
}
.btn.btn-orange {
background-color: #f59555;
box-shadow: 0 0 0.7rem #f59555, 0 4px #e25521;
}
.btn:active {
transform: translateY(2px);
}
.btn.btn-blue:active {
box-shadow: 0 0 0.7rem #5bb1fc, 0 2px #2d57bb;
}
.btn.btn-orange:active {
box-shadow: 0 0 0.7rem #f59555, 0 2px #e25521;
}
.btn:disabled {
opacity: 0.5;
cursor: default;
}
.modal {
width: 100%;
height: 100vh;
background-color: #212a37;
position: absolute;
top: 0;
left: 0;
opacity: 0;
transform: scale(0);
z-index: 9999;
transition: opacity 0.5s;
display: grid;
place-content: center;
gap: 1.5rem 0;
text-align: center;
}
.modal.active {
opacity: 1;
transform: scale(1);
}
.game-over {
font-size: 6rem;
}
.numbers-guess{
margin-top: 20px;
opacity: 0;
}
💡 Now It’s time For Javascript Code 😎.
👉 Create 📂index.js File
The first thing I did was to create references to the necessary HTML elements.
const numberLabel = document.querySelector(".hidden-number-label");
const playBtn = document.querySelector(".play");
const continueBtn = document.querySelector(".continue");
const inputGuessNumber = document.querySelector(".guess");
const message = document.querySelector(".feedback-label");
const scoreLabel = document.querySelector(".score");
const highScoreLabel = document.querySelector(".high-score");
const livesCountLabel = document.querySelector(".lives-count");
const gameOver = document.querySelector(".modal");
const game = document.querySelector(".game-wrapper");
const retry = document.querySelector(".retry");
const numbersGuess = document.querySelector(".numbers-guess");
const guessdNumbersLabel = document.querySelector(".guessed-number");
var guessedNumbers = [];
var score = 0;
var lifLine = 5;
Here we take blank array guessedNumbers for storing guessed numbers in an array. A score variable for storing scores and in a lifeline variable store a 5. When the user guesses a wrong number then the lifeline is decreased. And when the lifeline is zero then the game is over.
Random Number Generate
function randomNumberGenerate() {
return Math.floor(Math.random() * 100) + 1;
}
The random() function is used to generate a random number between 0 (inclusive) and 1 (exclusive). This generated number is then multiplied by 100 and added 1 to generate numbers from 1 – 100. The floor() function is used to return the number to the nearest integer (downwards). The value will not be rounded if the passed argument is an integer.
Show Guessed Numbers
function showGuessedNumbers(guessedNumbers) {
numbersGuess.style.opacity = 1;
guessdNumbersLabel.innerHTML = guessedNumbers;
}
In showGuessedNumbers() function, we need to pass an argument guessedNumbers. When the user guesses a wrong number. Then the number is stored in the guessedNumbers array and shown in guessdNumbersLabel. Here if the user guesses the wrong number then we show the number guesses div.
Check Is Game Over
function checkIsGameOver(lifLine) {
if (lifLine === 0) {
gameOver.style.opacity = 1;
gameOver.style.transform = "scale(1)";
game.style.opacity = 0;
}
}
Here checkIsGameOver() function take argument lifeline. Here when the user guesses a wrong number then the lifeline is decreased. If lifeline is zero then the game is over so here lifeline is zero then game over model opacity is 1 and game wrapper opacity is 0.
Save HighScore
function saveHighScore(score) {
localStorage.setItem("highscore", score);
}
saveHighScore() function for save high score in local storage. Here saveHighScore() function take one argument score. The setItem() method sets the value of the specified Storage Object item.
Show HighScore
function showHighScore() {
const highScore = localStorage.getItem("highscore");
console.log("highscore", highScore);
console.log("highscore", typeof highScore);
if (highScore == null) {
highScoreLabel.innerHTML = 0;
} else {
highScoreLabel.innerHTML = highScore;
}
}
showHighScore() function for showing high score. In this function, we first get the high score value from local storage using getItem() method. Here getItem() method returns a value of the specified Storage Object item. Here we check when the high score value is null then showing in highScoreLabel is zero otherwise shows a high score number. Let me explain when we first time we call this function then we haven’t any high score value means the high score value is null. After when the user plays the game and corrects guess a number then we assign score value in high score variable.
Check HighScore
function checkHighScore(score) {
const highScore = JSON.parse(localStorage.getItem("highscore"));
if (score > highScore) {
saveHighScore(score);
showHighScore();
}
}
Here checkHighScore() function check if the score is greater than the high score then we update the high score and show a high score.
👉 Here we defined all functions now. It’s time to add an event listener in the play button.
var answer = randomNumberGenerate();
playBtn.addEventListener("click", () => {
const user_guess = inputGuessNumber.value;
if (user_guess < 1 || user_guess > 100) {
alert("Please Enter A Number Between 1 to 100.");
} else {
if (user_guess < answer) {
message.innerHTML = "Your guess is too low";
lifLine--;
checkIsGameOver(lifLine);
livesCountLabel.innerHTML = lifLine;
guessedNumbers.push(user_guess);
showGuessedNumbers(guessedNumbers);
} else if (user_guess > answer) {
message.innerHTML = "Your guess is too high";
lifLine--;
checkIsGameOver(lifLine);
livesCountLabel.innerHTML = lifLine;
guessedNumbers.push(user_guess);
showGuessedNumbers(guessedNumbers);
} else if (user_guess == answer) {
message.innerHTML = "You Guessed correctly!";
numberLabel.innerHTML = user_guess;
playBtn.disabled = true;
continueBtn.disabled = false;
score++;
scoreLabel.innerHTML = score;
checkHighScore(score);
}
}
inputGuessNumber.value = "";
});
Here we store a random number in the answer variable. Now we add the event listener in playBtn. In the event listener function, we store user guessed number in the user_guess variable. And check condition if the user enters zero and greater than 100 then we show an alert message.
Now we check the condition if user_guess is lower than the answer. Then show message Your guess is too low and also lifeline is decreased. And pass lifeline in checkIsGameOver() function. Where check if lifeline is zero then the game is over. After show guess numbers. Also similar in user_guess is higher than answer.
When the user guesses the right answer then show the message You Guessed correctly. After we disabled the play button and enabled the continue button so the user can play again. Here we also checkHighScore() function for update high score value.
Continue Button
continueBtn.addEventListener("click", () => {
answer = randomNumberGenerate();
console.log("answer contimue", answer);
playBtn.disabled = false;
continueBtn.disabled = true;
numberLabel.innerHTML = "?";
guessedNumbers = [];
numbersGuess.style.opacity = 0;
guessdNumbersLabel.innerHTML = "";
});
Now we add an event listener in the continue button for play again. Here we generate a new random number and store it in the answer variable. After enable the play button and disabled the continue button and also reset guessed numbers.
Retry Button
retry.addEventListener("click", () => {
gameOver.style.opacity = 0;
gameOver.style.transform = "scale(0)";
game.style.opacity = 1;
message.innerHTML = "";
lifLine = 5;
score = 0;
guessedNumbers = [];
livesCountLabel.innerHTML = lifLine;
scoreLabel.innerHTML = score;
numbersGuess.style.opacity = 0;
guessdNumbersLabel.innerHTML = "";
showHighScore();
});
In this function reset all values like a lifeline, score, etc.
Number Guessing Game JavaScript(Source Code)
👉 watch the video Number Guessing Game Javascript