In this article, we will generate a random number in JavaScript. We will use the Math()
library which is provided by JavaScript. Since we know that Math library contains functions who are actually related to mathematics. So we will here use the Math.random()
function to get random number, but random() function only returns float values which ranges between 0 and 1. This is why we need to customize this, so it should any random value.
This program is very basic and for beginners, so let’s just make this and understand with clear and basic language.
Generating Normal Random Value Between 0 and 1
Firstly, we need to add HTML file, in this file we will add normal HTML markup. Now we have added a <script> tag in which we will add our JS code. Here we have added a variable in which we have assigned value from Math.random
a function. So in this variable, we have a random float value between 0 and 1. And we just used the document.write
method to show the result. You can notice here we have added <h1> inside the JS code and used $ sign for our variable, these two things will work when we use ``
(backtick).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script type="text/javascript">
var x = Math.random();
document.write(`<h1>generated value: ${x}</h1>`);
</script>
</body>
</html>
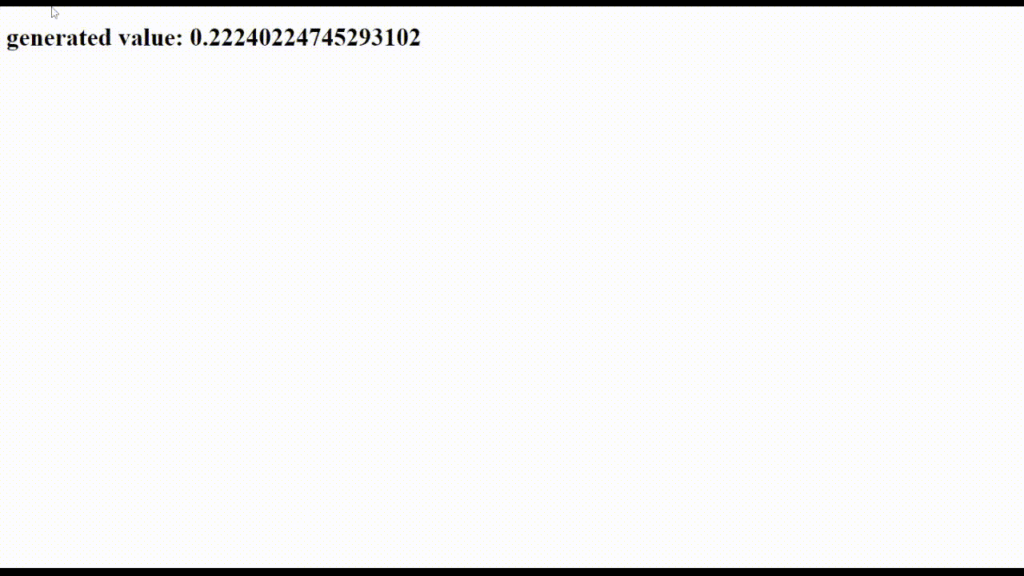
As we can see, we are able to generate random number and if we refresh then we get different and random values.
Generating The Within Range
We have here copied the above code, and we have just added one more line here, we have just multiplied the value with 100. This is because we need a value between 0 and 100, that means you just need to multiply the highest value of range? So yes, for example, if we require a random value between 0 and 1000 then you just need to multiply by 1000. Now if we need a value between 20 and 500, then we need to multiply then value with 500 and add 20. Like x = (x * 500) + 20;
. Remember, all of these value will be in float, means decimal values will be in there.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script type="text/javascript">
var x = Math.random();
document.write(`<h1>generated value: ${x}</h1>`);
x = x * 100;
document.write(`<h1> Ranged generated value: ${x}</h1>`);
</script>
</body>
</html>
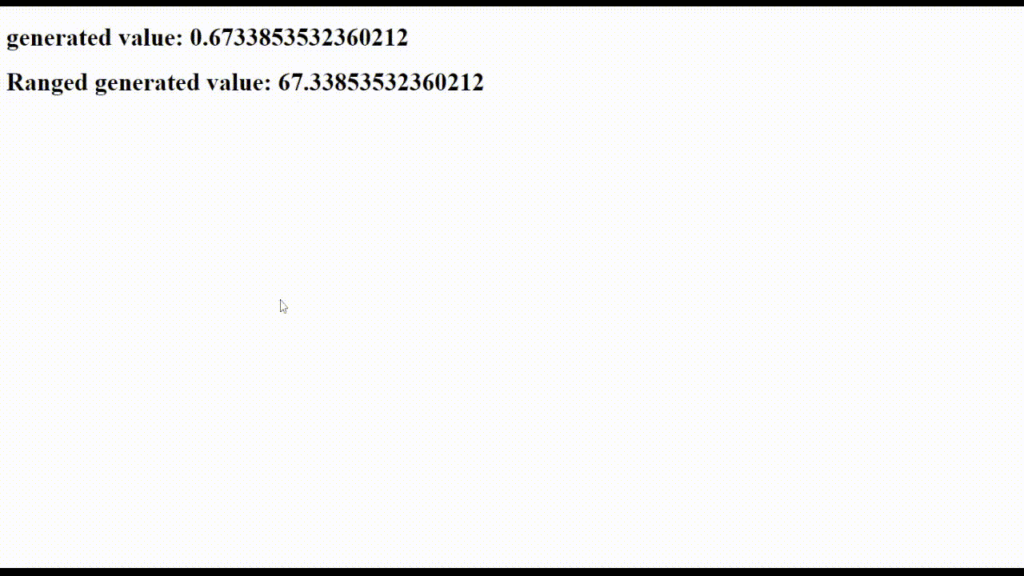
Generating Random Integer Value
Now we have again copied the above code, and again we have added a single line of code. In here, we have applied the Math.floor()
function on our variable to get an integer value. Floor function will remove the decimals and show only integer. Here one more thing which I want to point out is, we can also use parseInt()
to get integer value, but it won’t be good practice, because parseInt()
converts the string to integer number. So it’s better to use floor function.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script type="text/javascript">
var x = Math.random();
document.write(`<h1>generated value: ${x}</h1>`);
x = x * 100;
document.write(`<h1> Ranged generated value: ${x}</h1>`);
x= Math.floor(x);
document.write(`<h1> Integer Ranged generated value: ${x}</h1>`);
</script>
</body>
</html>
Output
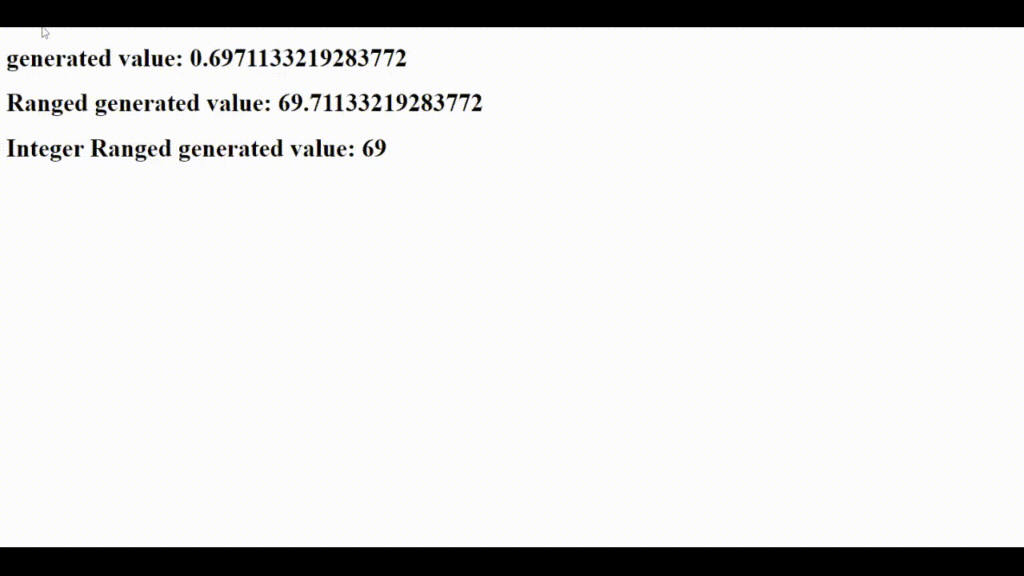