In this article, we are going to learn and see to computing Fibonacci sequence in JavaScript. It is a basic problem, or you can say a program in JavaScript, computing Fibonacci sequence becomes more problematic when you don’t know its main logic. So we are just going to make a Fibonacci sequence with some easier and good way to help out beginners.
What is Fibonacci Sequence?
Fibonacci’s sequence is pretty easy to understand, here we have two input values, one is an initial value and the other one is a number of passes. For example, if we need to find Fibonacci sequence starts from 1 till 5 passes then sequence will be 1,1,2,3,5.
So here we have simple logic in order to create Fibonacci sequence is, we have two initial values, as we have to assign 0,1. Then the 3rd value of this sequence will be 0+1 =1, 4th value will be 1+1 =2, and 5th value will be 1+2 = 3 etc. So basically we are deciding the next number by adding preceding values or two before values.
Program Creation For Fibonacci Sequence
Okay, There are multiple ways to create this sequence, but we will see two basic and easy methods to create this sequence. (1) using loop, (2) using function recursion.
Fibonacci Sequence Using Loop
The first method is to use loop, here we are using while loop, but you can go with for loop as well. Here we have added a variable in which we have added a function with two parameter result and len. result is an array where we will add the result of each loop.
Then we have added two variable num1 and num2 where we have assigned 1st and 2nd value of the array. After that, we have also added a temporary variable “next” and cnt =2, and we are just adding the num1 and num2 and assigning in variable next. Then we assigned value of num2 in num1 and value of next in num2, and we’re just pushing the value of next in to the result array.
var fibo = function(result, len){
var num1 = result[0],
num2 = result[1],
next,
cnt = 2;
while(cnt < len){
next = num1 + num2;
num1 = num2;
num2 = next;
result.push(next);
cnt++;
}
return result;
}
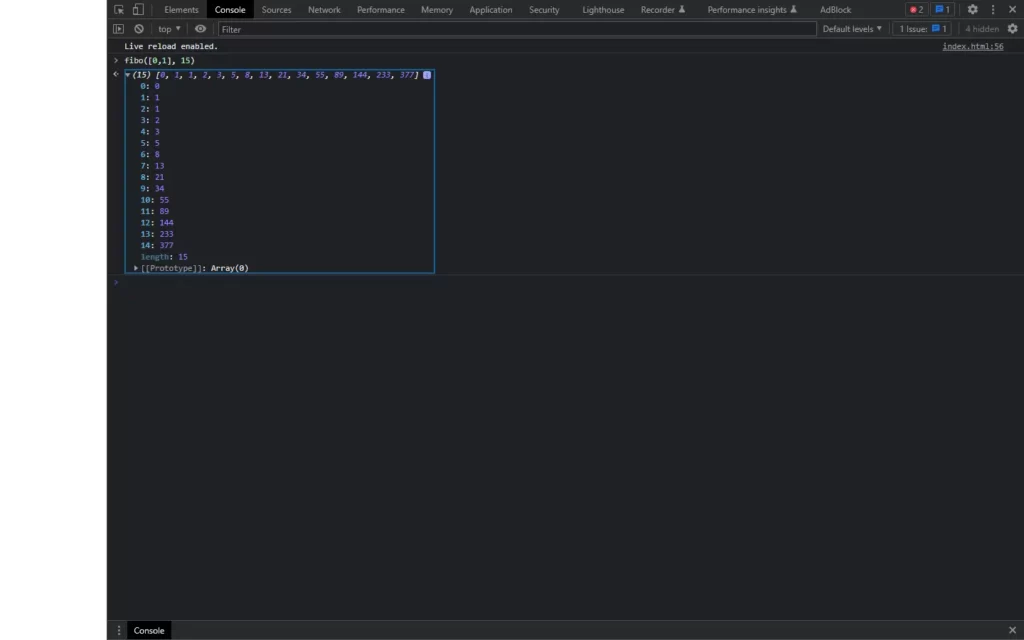
Fibonacci Sequence Using Recursion
Another technique to find Fibonacci sequence is using recursion or function recursion. Function recursion, actually a little bit hard, but it also reduces lines of code. So learning recursion would be good. Basically recursion is a function calls itself, so if the function has some value to do operation then the function dynamically calls itself, so the function will run until we add some break point.
Here we have again added fibo function with result array and length as parameter. Then we will check a condition where the length of the array is greater or equal to the provided length, in that case, we will simply return a result array. Otherwise, we will fetch the second last and last element of the array, and we will perform addition and push its result in the array. Now we will return function call fibo with update array and length to run again function.
var fibo = function fibo(result, len){
if(result.length>= len){
return result;
}
result.push(result[result.length-2]+result[result.length-1]);
return fibo(result, len);
}
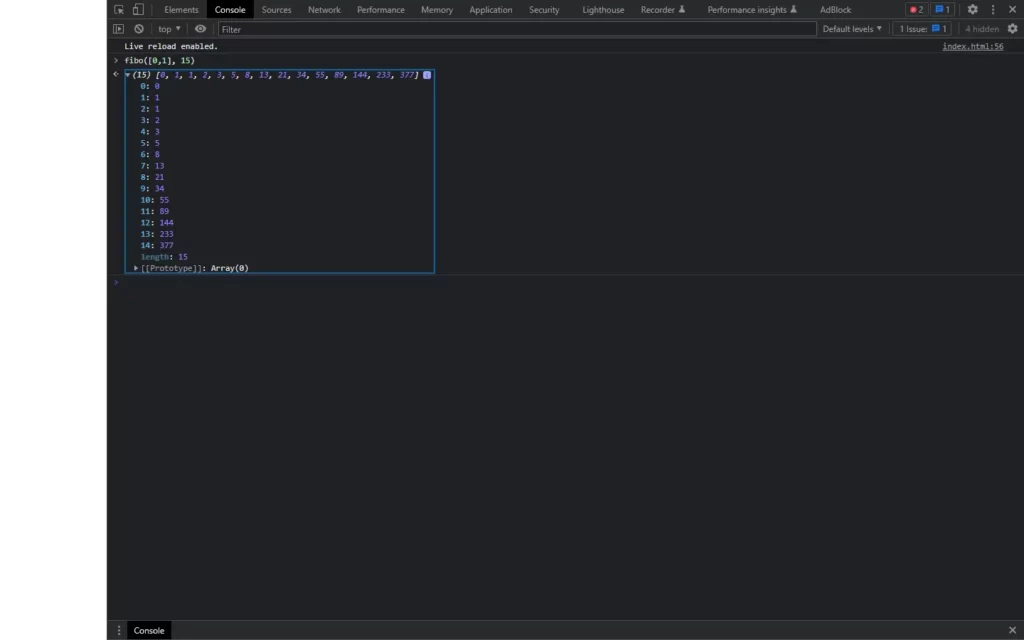