In this article, we will make a program to check if a number is positive, negative, or zero. We will have an input field to get user input, and a button with functionality to check the nature of the number. We will add this functionality using JavaScript. This will be very easy to program and also beginner-friendly. So we will try to learn these programs with easier explanation. So let’s create these programs one by one.
Creating HTML Elements
First of all, we have created an HTML markup, in this we have added an input field with number type, and we gave “data” as ID. Then we have added a button on which we have applied onclick event listener which listens the click event on the button, we also gave a function which will run when the click event fires. After that, we have added a <h1> heading with “res” ID.
Lastly, we have added main.js
file path as our source for JavaScript code. We are here using external JS, so we just need to provide file path in src
attribute.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Addimg two numbers</title>
</head>
<body>
<input type="number" id="data" step="any"/>
<button onclick="check()">Check</button>
<h1 id = "res"></h1>
<script src="main.js"></script>
</body>
</html>
Adding Functionality Using JS
In JS file, we have created a function check()
which we have called in the button. In this function, we have fetched the value of the input field into the value variable using document.getElementById('data').value
. Here, we are targeting the input field with its ID “data” using this document.getElementById('data')
method.
Now we are using Math.sign()
method, this method is used to check the nature of the value. This will return corresponding values, like for negative value we get -1, for zero we get 0, and for positive value we will get 1. Now we have added conditions for these values came from Math.sign()
method. For Positive value, If the result greater than 0 then we add a string which says it is positive. Else if result less than 0 then we add a string which says it is negative. Else, if the result is 0 then we add a string which says it is zero.
Lastly, we need to add the result string using document.getElementById('res').innerText = `${result}`
, here we have targeted <h1> tag using res id and added result using innerText
attribute.
function check(){
var value = document.getElementById('data').value;
var result = Math.sign(value);
if(result>0){
result= `${value} is a positive number`;
}
else if(result<0){
result= `${value} is a negetive number`;
}
else{
result= `${value} is zero`;
}
document.getElementById('res').innerText = `${result}`;
}
Output
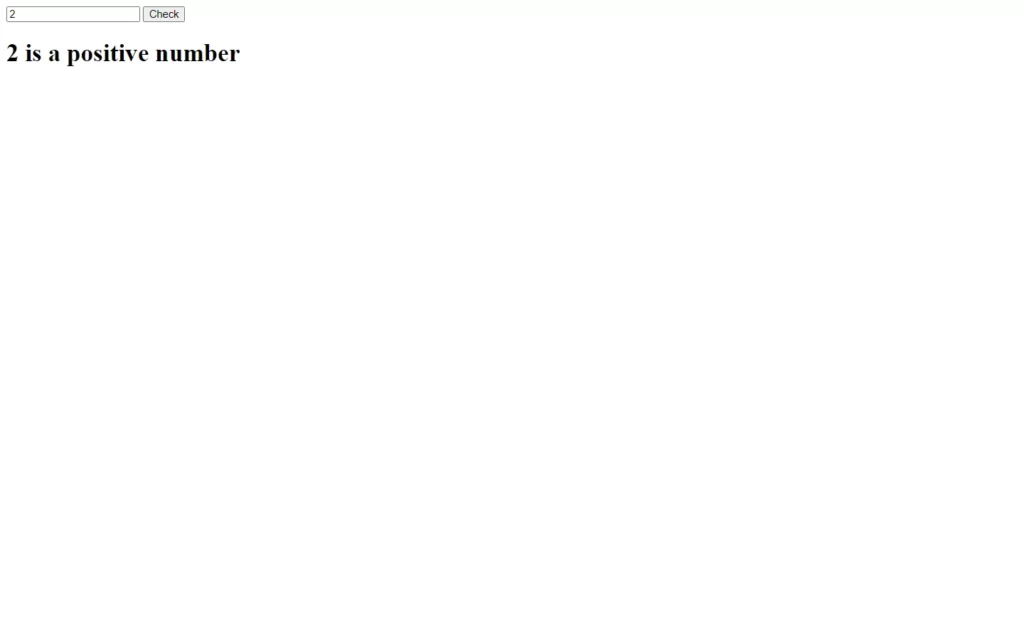
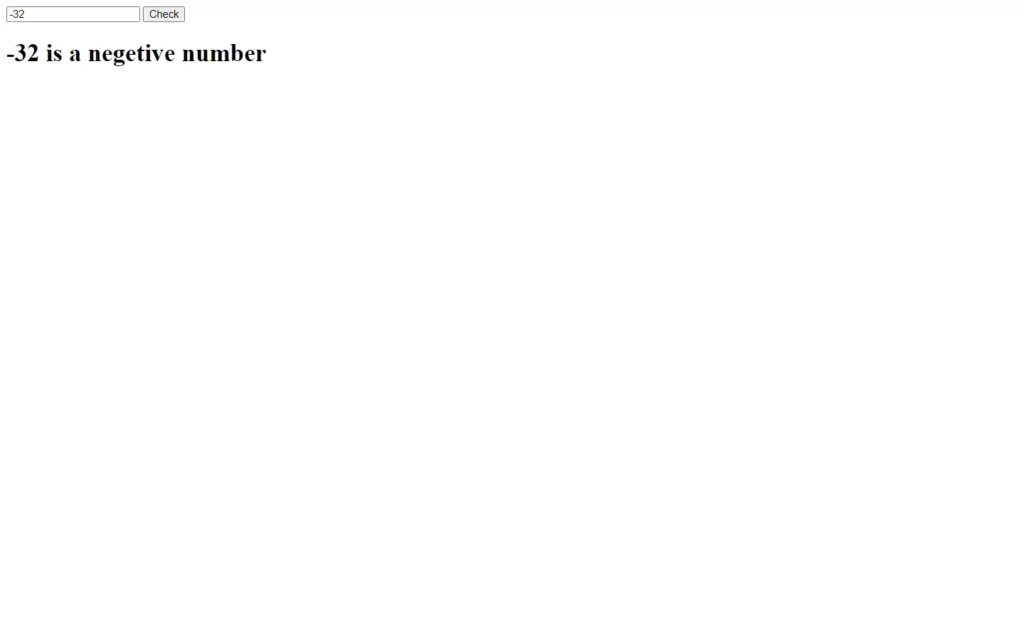
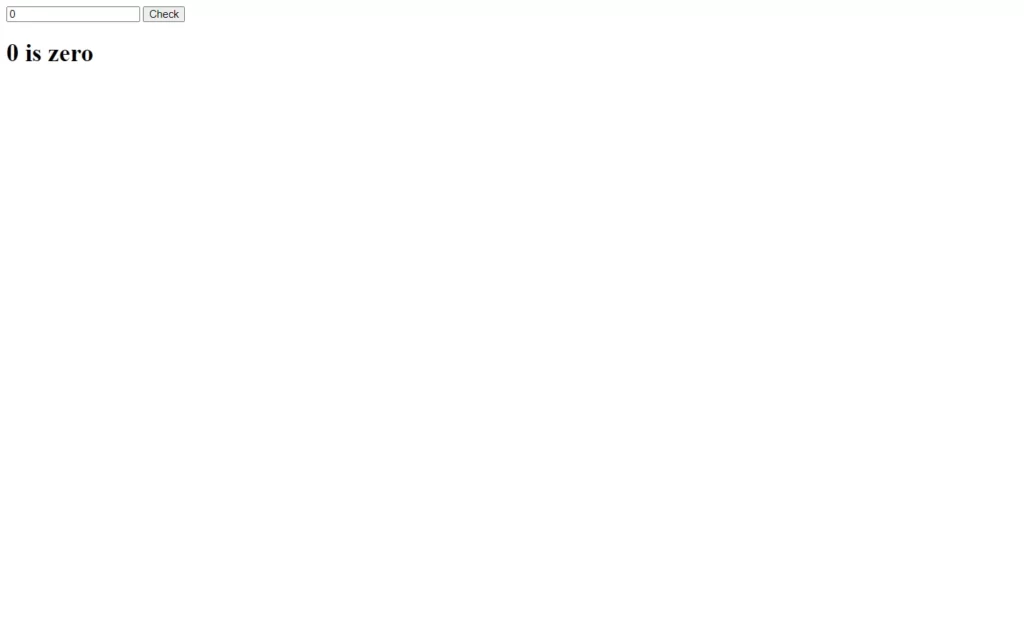