In this article, we will create a program to check if a number is odd or even using JavaScript. In this we will fetch the value given by the user, and we will check the number if it is odd or even using some basic logic. We will make this program using two methods:
- Using the if else statement
- Using ternary operator
This is going to be a very easy and simple program, so let’s just make it with these two methods.
Using If Else Statement
So firstly, we will make our HTML markup, then we have added our <script> tag in which we will write this program. So if you’re unaware with if….else statement, then let’s take a reminder that it is a conditional statement. If the condition becomes true, then it simply executes the code written inside the if statement. And if the condition becomes false, then the code written in the if statement will be skipped and move to next statement like on else statement. Simply if condition is true then executes the code and if condition is wrong then else will be executed.
In JS code, we have declared a variable x with some initial value. Then we have added if statement in which we are checking the condition x%2==0
, here ‘%’ operator returns the remainder of division of x%2. If remainder will be 0 then the value will be even, because we know that if we divide any value with 2 returns 0 remainder then that value will be even. Like 10%2, 24%2 etc.
We have added a <h1> with the message that number is even. If the condition is false then else part will be executed in which we have a message to print number is odd.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script type="text/javascript">
var x = 62;
if(x % 2 ==0){
document.write(`<h1> ${x} is an even number</h1>`);
}
else{
document.write(`<h1> ${x} is an odd number</h1>`);
}
</script>
</body>
</html>
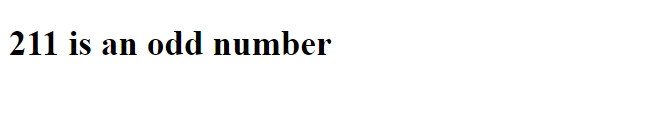
If Else Program Using User’s Input
Okay, we have created the code to understand basic logic of our if…else program. Now we will get the value from the user and check it.
Here, as you can see, program logic is common, but we have added an input field in which we will get the value from the user. And also added a button on which we have added an event listener to listen the click. So if the button gets clicked, then we will call a function named “get”.
We have defined this function inside the JS Code. In this function, we have added a variable in which we have stored the value from the input field. Here, we have used the document.querySelector()
method to fetch the input field element from DOM, and used the value attribute to fetch the value from the input field. Then after, we have just added the same condition as above to check number’s property.
Here a question is raised like what do we need to check 2 or more conditions? So the answer is, you can check the second condition with the help of the else if statement. You can read more about it here.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<input type="number"/>
<button onclick= get()>Click me</button>
<script type="text/javascript">
function get() {
var x = document.querySelector('input').value;
if (x % 2 == 0) {
document.write(`<h1> ${x} is an even number</h1>`);
} else {
document.write(`<h1> ${x} is an odd number</h1>`);
}
}
</script>
</body>
</html>
Using Ternary Operator
Ternary operator is pretty much straight forward and has less code than the if else statement. The syntax for ternary operator is condition? true: false
. So here we will condition if the condition is right than the first statement which is next to question mark will be executed. And if it is wrong, then the second statement which is next to the colon sign.
Now, here we have declared a variable with some random value. Then we have declared another variable in which we have checked the condition if it is true then we will add a string “even”, and if it is false then we will add a string “odd”.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<script type="text/javascript">
var x = 211
var res = x % 2 == 0 ? "even": "odd";
document.write(`<h1>${x} is an ${res} number`)
</script>
</body>
</html>
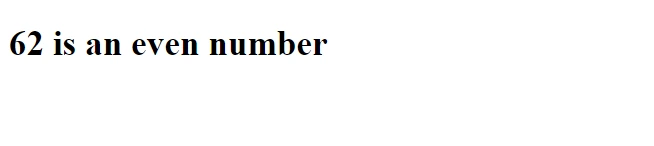
Ternary Program Using User’s Input
Similar to the If else statement code, we kept the main logic as it is, and we have added an input field along with a button on which we have an onclick event listener with a function call. We have just declared this function in our JS code. In this function, we have fetched the input field using document.querySelector
method and used value attribute to get the value from that input.
Then we have applied the same logic to check number’s property.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<input type="number" />
<button onclick=get()>Click Me</button>
<script type="text/javascript">
function get(){
var x = document.querySelector("input").value;
var res = x % 2 == 0 ? "even": "odd";
document.write(`<h1>${x} is an ${res} number`)
}
</script>
</body>
</html>