In this article, we are going to see how to make Neumorphism Profile Card UI Design using only HTML & CSS, there is a profile card with a neomorphic effect. This card contains a profile image, social media buttons, and some social media info. When you hover on particular social media buttons, there is shown a neomorphic effect. This card is fully based on HTML & CSS.
You can see the demo here Neumorphism Profile Card
Pre-requisites To Make Neumorphism Profile Card UI Design using only HTML & CSS
- Good knowledge of HTML.
- Good knowledge of CSS & CSS3.
Creating HTML Markup
<head><script src="<https://kit.fontawesome.com/a076d05399.js>"></script></head>
<body>
<div class="wrapper">
<div class="img-area">
<div class="inner-area">
<img src="<https://images.unsplash.com/photo-1464746133101-a2c3f88e0dd9?crop=entropy&cs=tinysrgb&fm=jpg&ixlib=rb-1.2.1&q=80&raw_url=true&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=843>" alt="">
</div>
</div>
<div class="icon arrow"><i class="fas fa-arrow-left"></i></div>
<div class="icon dots"><i class="fas fa-ellipsis-v"></i></div>
<div class="name">RoCoderes</div>
<div class="about">Designer & Developer</div>
<div class="social-icons">
<a href="#" class="fb"><i class="fab fa-facebook-f"></i></a>
<a href="#" class="twitter"><i class="fab fa-twitter"></i></a>
<a href="#" class="insta"><i class="fab fa-instagram"></i></a>
<a href="#" class="yt"><i class="fab fa-youtube"></i></a>
</div>
<div class="buttons">
<button>Message</button>
<button>Follow</button>
</div>
</div>
</body>
First of all, let’s just create our skeleton or basic elements of the card, so for that we will add an image which can be anything we have added some random image here, after that we will add font awesome, so we can get icons from that. now we need here back button, which is simply left arrow also 3 dots icon which you might often see it.
Now we need social media buttons for this we will add icons for Facebook, Twitter, Instagram and YouTube also we need two buttons for message and follow.
@import url('<https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap>');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
background: #ecf0f3;
}
.wrapper{
position: relative;
width: 350px;
padding: 30px;
border-radius: 10px;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
}
Now let’s move on CSS, here we will add google font named Poppins for this we added link for Poppins font from google, after that we need to remove default margin and padding for that set it to 0, also add Poppins to font family, now we need every element at center for that we will use display to flex, align-items and justify-content to center, also we set min height to 100vh and background to #ecf0f3.
After that, we need position to relative also we adjust the width and padding for the card, now again we will set elements to center.
Customizing The Profile Card Image
.wrapper,
.wrapper .img-area,
.social-icons a,
.buttons button{
background: #ecf0f3;
box-shadow: -3px -3px 7px #ffffff,
3px 3px 5px #ceced1;
}
.wrapper .img-area{
height: 150px;
width: 150px;
border-radius: 50%;
display: flex;
align-items: center;
justify-content: center;
}
.img-area .inner-area{
height: calc(100% - 25px);
width: calc(100% - 25px);
border-radius: 50%;
}
.inner-area img{
height: 100%;
width: 100%;
border-radius: 50%;
object-fit: cover;
}
Now let’s set our card with background color of #ecf0f3 and let’s put some box shadow with this we can see that our card will look like somewhat 3D type, then we need to set image for that we will add area for image for that set height and width, and we need here round area for image.
After that, we will set inner area, or you can say another border to image area for that we used calc function, but we will reduce 25px from 100% of height and width also border radius to 50%, now for image we need to add object fit to cover with the image will be set into image area.
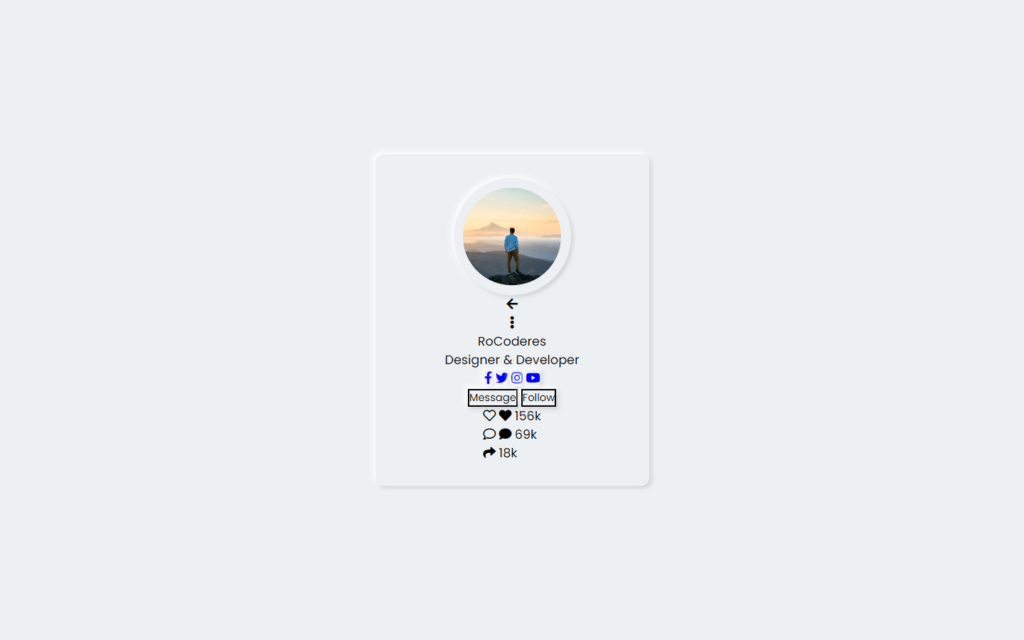
Customizing The Icons
.wrapper .icon{
font-size: 17px;
color: #31344b;
position: absolute;
cursor: pointer;
opacity: 0.7;
top: 15px;
height: 35px;
width: 35px;
text-align: center;
line-height: 35px;
border-radius: 50%;
font-size: 16px;
}
.wrapper .icon i{
position: relative;
z-index: 9;
}
.wrapper .icon.arrow{
left: 15px;
}
.wrapper .icon.dots{
right: 15px;
}
Now let’s set 3 dots and left arrow icons for that we need grey color, cursor to pointer also opacity to 0.7, text align we need here center, then line height will be 35px. after that, we need to set arrow to left side and dots to right side of the card.
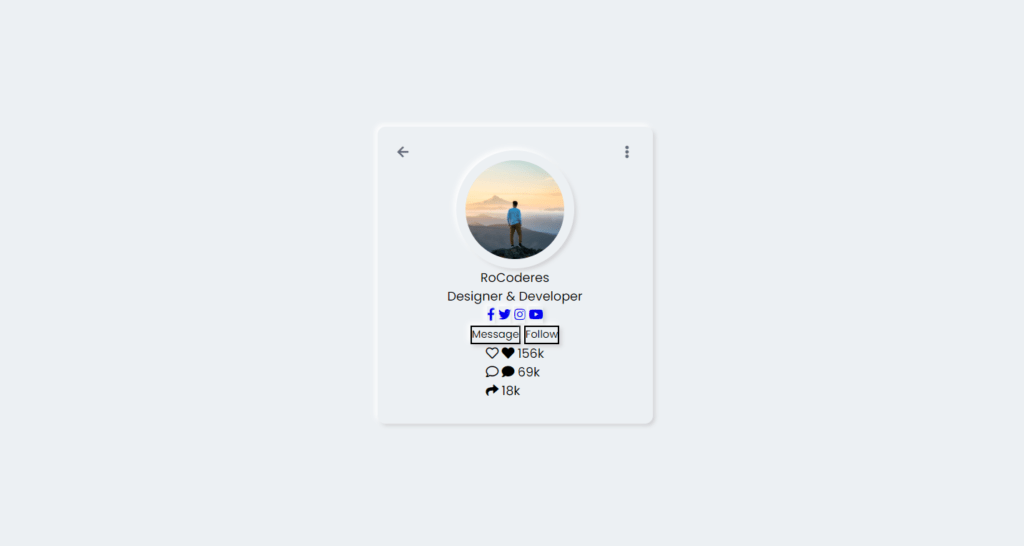
Customizing Social-Media Icons
.wrapper .name{
font-size: 23px;
font-weight: 500;
color: #31344b;
margin: 10px 0 5px 0;
}
.wrapper .about{
color: #44476a;
font-weight: 400;
font-size: 16px;
}
.wrapper .social-icons{
margin: 15px 0 25px 0;
}
.social-icons a{
position: relative;
height: 40px;
width: 40px;
margin: 0 5px;
display: inline-flex;
text-decoration: none;
border-radius: 50%;
}
.social-icons a:hover::before,
.wrapper .icon:hover::before,
.buttons button:hover:before{
content: "";
position: absolute;
top: 0;
left: 0;
bottom: 0;
right: 0;
border-radius: 50%;
background: #ecf0f3;
box-shadow: inset -3px -3px 7px #ffffff,
inset 3px 3px 5px #ceced1;
}
.social-icons a i{
position: relative;
z-index: 3;
text-align: center;
width: 100%;
height: 100%;
line-height: 40px;
}
.social-icons a.fb i{
color: #4267B2;
}
.social-icons a.twitter i{
color: #1DA1F2;
}
.social-icons a.insta i{
color: #E1306C;
}
.social-icons a.yt i{
color: #ff0000;
}
Now we need to set name and about section for that we will add some font weight and some font size, after that we will customize social media icons, we add display to inline-flex with this we will maintain icons in row, then we don’t need any text decoration with this we can remove some underline below the icons, also we add box-shadow in every before hovering effect, after that we will add corresponding colors of social media icons.
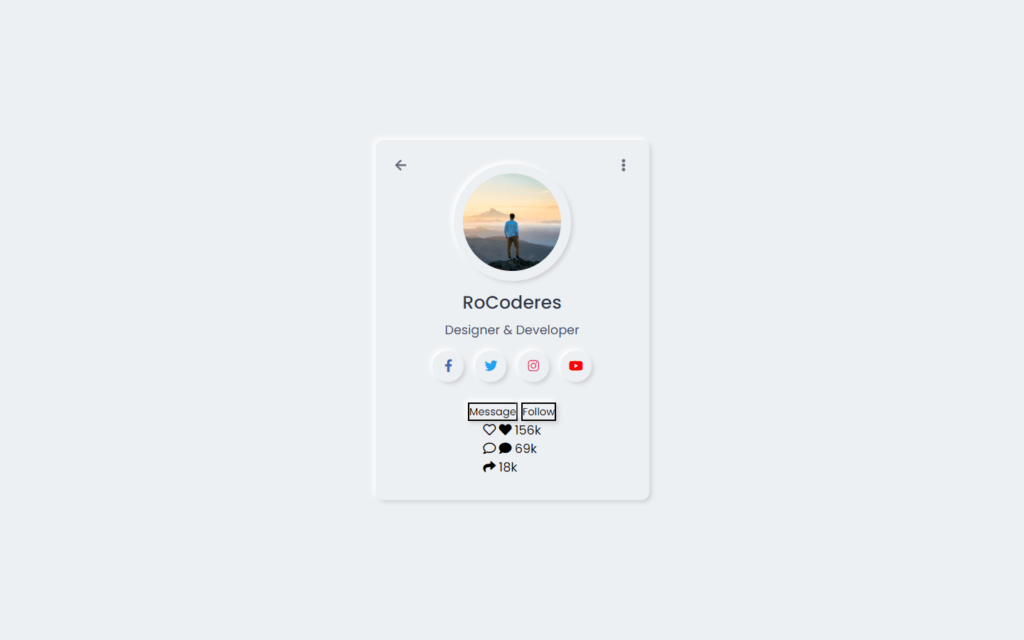
Customizing The Buttons
.wrapper .buttons{
display: flex;
width: 100%;
justify-content: space-between;
}
.buttons button{
position: relative;
width: 100%;
border: none;
outline: none;
padding: 12px 0;
color: #31344b;
font-size: 17px;
font-weight: 400;
border-radius: 5px;
cursor: pointer;
z-index: 4;
}
.buttons button:first-child{
margin-right: 10px;
}
.buttons button:last-child{
margin-left: 10px;
}
Now for the buttons, we need to separate the buttons using space-between, then we will remove border and outline with none value, also font weight of 400 again set the cursor to pointer, and z-index to 4, so it will give 3d effect when clicking on the buttons.
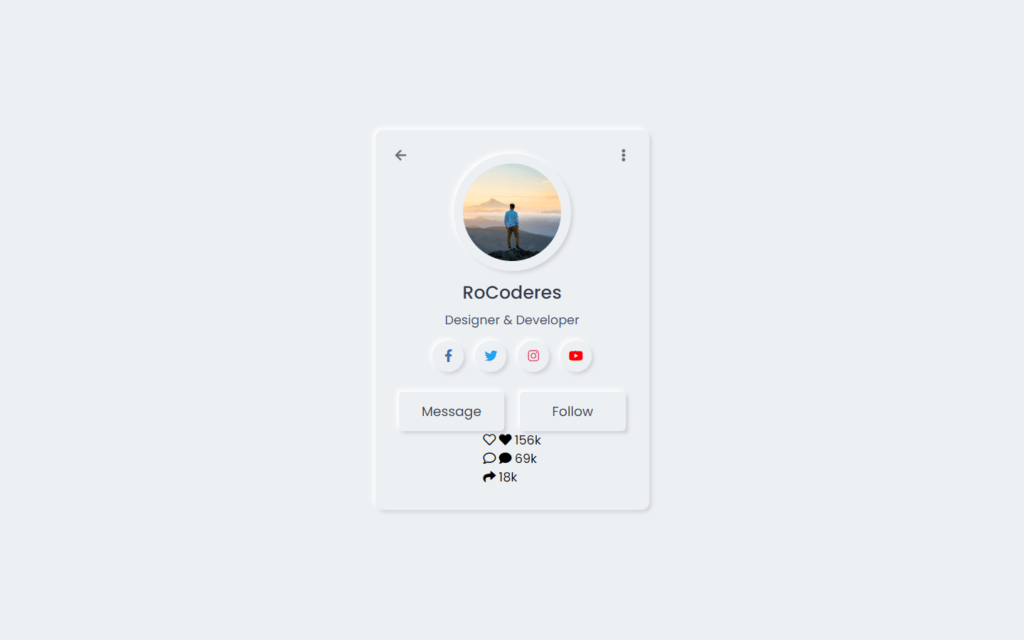
Customizing The Social-Share Buttons
Now we need to add like, comment and share button using the font-awesome:
<div class="social-share">
<div class="row">
<i class="far fa-heart"></i>
<i class="icon-2 fas fa-heart"></i>
<span>156k</span>
</div>
<div class="row">
<i class="far fa-comment"></i>
<i class="icon-2 fas fa-comment"></i>
<span>69k</span>
</div>
<div class="row">
<i class="fas fa-share"></i>
<span>18k</span>
</div>
</div>
.wrapper .social-share{
display: flex;
width: 100%;
margin-top: 30px;
padding: 0 5px;
justify-content: space-between;
}
.social-share .row{
color: #31344b;
font-size: 17px;
cursor: pointer;
position: relative;
}
.social-share .row::before{
position: absolute;
content: "";
height: 100%;
width: 2px;
background: #e0e6eb;
margin-left: -25px;
}
.row:first-child::before{
background: none;
}
.social-share .row i.icon-2{
position: absolute;
left: 0;
top: 50%;
color: #31344b;
transform: translateY(-50%);
opacity: 0;
pointer-events: none;
transition: all 0.3s ease;
}
.row:nth-child(1):hover i.fa-heart,
.row:nth-child(2):hover i.fa-comment{
opacity: 1;
pointer-events: auto;
}
After that, we need to Customize the social share icons for that first we need some space among these icons also we will set cursor to pointer when hovering, after that we remove the background from icons, again we need to translate to Y, and we will add transition for smoother effect and finally, we will add opacity to 1 while hovering the icons also set all pointer events to auto.
Full Source Code Of Neumorphism Profile Card UI Design using only HTML & CSS
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Neumorphism Profile Cardl</title>
<link rel="stylesheet" href="style.css">
<script src="<https://kit.fontawesome.com/a076d05399.js>"></script>
</head>
<body>
<div class="wrapper">
<div class="img-area">
<div class="inner-area">
<img src="<https://images.unsplash.com/photo-1464746133101-a2c3f88e0dd9?crop=entropy&cs=tinysrgb&fm=jpg&ixlib=rb-1.2.1&q=80&raw_url=true&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=843>" alt="">
</div>
</div>
<div class="icon arrow"><i class="fas fa-arrow-left"></i></div>
<div class="icon dots"><i class="fas fa-ellipsis-v"></i></div>
<div class="name">RoCoderes</div>
<div class="about">Designer & Developer</div>
<div class="social-icons">
<a href="#" class="fb"><i class="fab fa-facebook-f"></i></a>
<a href="#" class="twitter"><i class="fab fa-twitter"></i></a>
<a href="#" class="insta"><i class="fab fa-instagram"></i></a>
<a href="#" class="yt"><i class="fab fa-youtube"></i></a>
</div>
<div class="buttons">
<button>Message</button>
<button>Follow</button>
</div>
<div class="social-share">
<div class="row">
<i class="far fa-heart"></i>
<i class="icon-2 fas fa-heart"></i>
<span>156k</span>
</div>
<div class="row">
<i class="far fa-comment"></i>
<i class="icon-2 fas fa-comment"></i>
<span>69k</span>
</div>
<div class="row">
<i class="fas fa-share"></i>
<span>18k</span>
</div>
</div>
</div>
</body>
</html>
style.css
@import url('<https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap>');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
background: #ecf0f3;
}
.wrapper,
.wrapper .img-area,
.social-icons a,
.buttons button{
background: #ecf0f3;
box-shadow: -3px -3px 7px #ffffff,
3px 3px 5px #ceced1;
}
.wrapper{
position: relative;
width: 350px;
padding: 30px;
border-radius: 10px;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
}
.wrapper .icon{
font-size: 17px;
color: #31344b;
position: absolute;
cursor: pointer;
opacity: 0.7;
top: 15px;
height: 35px;
width: 35px;
text-align: center;
line-height: 35px;
border-radius: 50%;
font-size: 16px;
}
.wrapper .icon i{
position: relative;
z-index: 9;
}
.wrapper .icon.arrow{
left: 15px;
}
.wrapper .icon.dots{
right: 15px;
}
.wrapper .img-area{
height: 150px;
width: 150px;
border-radius: 50%;
display: flex;
align-items: center;
justify-content: center;
}
.img-area .inner-area{
height: calc(100% - 25px);
width: calc(100% - 25px);
border-radius: 50%;
}
.inner-area img{
height: 100%;
width: 100%;
border-radius: 50%;
object-fit: cover;
}
.wrapper .name{
font-size: 23px;
font-weight: 500;
color: #31344b;
margin: 10px 0 5px 0;
}
.wrapper .about{
color: #44476a;
font-weight: 400;
font-size: 16px;
}
.wrapper .social-icons{
margin: 15px 0 25px 0;
}
.social-icons a{
position: relative;
height: 40px;
width: 40px;
margin: 0 5px;
display: inline-flex;
text-decoration: none;
border-radius: 50%;
}
.social-icons a:hover::before,
.wrapper .icon:hover::before,
.buttons button:hover:before{
content: "";
position: absolute;
top: 0;
left: 0;
bottom: 0;
right: 0;
border-radius: 50%;
background: #ecf0f3;
box-shadow: inset -3px -3px 7px #ffffff,
inset 3px 3px 5px #ceced1;
}
.buttons button:hover:before{
z-index: -1;
border-radius: 5px;
}
.social-icons a i{
position: relative;
z-index: 3;
text-align: center;
width: 100%;
height: 100%;
line-height: 40px;
}
.social-icons a.fb i{
color: #4267B2;
}
.social-icons a.twitter i{
color: #1DA1F2;
}
.social-icons a.insta i{
color: #E1306C;
}
.social-icons a.yt i{
color: #ff0000;
}
.wrapper .buttons{
display: flex;
width: 100%;
justify-content: space-between;
}
.buttons button{
position: relative;
width: 100%;
border: none;
outline: none;
padding: 12px 0;
color: #31344b;
font-size: 17px;
font-weight: 400;
border-radius: 5px;
cursor: pointer;
z-index: 4;
}
.buttons button:first-child{
margin-right: 10px;
}
.buttons button:last-child{
margin-left: 10px;
}
.wrapper .social-share{
display: flex;
width: 100%;
margin-top: 30px;
padding: 0 5px;
justify-content: space-between;
}
.social-share .row{
color: #31344b;
font-size: 17px;
cursor: pointer;
position: relative;
}
.social-share .row::before{
position: absolute;
content: "";
height: 100%;
width: 2px;
background: #e0e6eb;
margin-left: -25px;
}
.row:first-child::before{
background: none;
}
.social-share .row i.icon-2{
position: absolute;
left: 0;
top: 50%;
color: #31344b;
transform: translateY(-50%);
opacity: 0;
pointer-events: none;
transition: all 0.3s ease;
}
.row:nth-child(1):hover i.fa-heart,
.row:nth-child(2):hover i.fa-comment{
opacity: 1;
pointer-events: auto;
}
Output
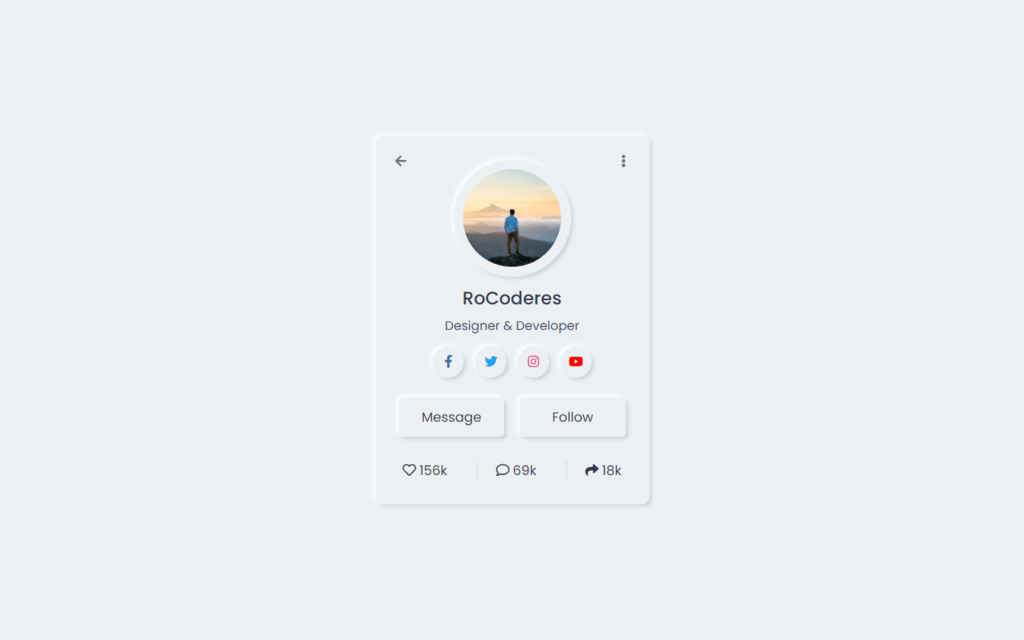
Check out Full Source Code On Github