In this article, we will make a language translator in JavaScript. In here, we will provide two fields for text, one for text input and another for output text. To get translate the language, we will use an API of mymemory. Using this API, we will also add other languages for selection, and also we provide a button to listen the text in the speech form. Lastly, we have added another button to copy the text, and also we will provide a button to translate the text.
This is going to be an interesting and cool project to do. So let’s make it step-by-step.
Pre-requisite to Make Language Translator in JavaScript
- Basic Knowledge of HTML.
- Basic Knowledge of CSS.
- Basic Knowledge of JavaScript.
- API key of mymemory translator.
Creating Skeleton
For this project, we need to basically three files. First will be our index.html, in this we will add our elements, and you can simply say we will create the skeleton of the project using HTML file. Then for designing purpose we will be adding our style.css file, with this we will add some styles to our HTML, this is going to be purely based on you, like you can customize it any way. And lastly, our script.js file, this will be our main file because we will add functionality so that we can translate the text using the JavaScript file.
Now in HTML, we have firstly added a font-awesome link to use icons. Then in body part, we have added two text-area for input and output text. In output text-area, we have added an attribute name readonly-disabled class, which is actually used to disable modification for the specific class. Simply, it will make output text-area which will be unchangeable.
After that, we have added two icons for volume and copy icons. Then we have added a select menu which is currently empty, but we will fill it using JS code. Also for output field, we again added same icons and select menu. Lastly, we have added a button to translate text from one language to targeted language.
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Language Translator</title>
<link rel="stylesheet" href="style.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Font Awesome CDN Link for Icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"/>
</head>
<body>
<div class="container">
<div class="wrapper">
<div class="text-input">
<textarea spellcheck="false" class="from-text" placeholder="Enter text"></textarea>
<textarea spellcheck="false" readonly disabled class="to-text" placeholder="Translation"></textarea>
</div>
<ul class="controls">
<li class="row from">
<div class="icons">
<i id="from" class="fas fa-volume-up"></i>
<i id="from" class="fas fa-copy"></i>
</div>
<select></select>
</li>
<li class="exchange"><i class="fas fa-exchange-alt"></i></li>
<li class="row to">
<select></select>
<div class="icons">
<i id="to" class="fas fa-volume-up"></i>
<i id="to" class="fas fa-copy"></i>
</div>
</li>
</ul>
</div>
<button>Translate Text</button>
</div>
<script src="js/countries.js"></script>
<script src="js/script.js"></script>
</body>
</html>
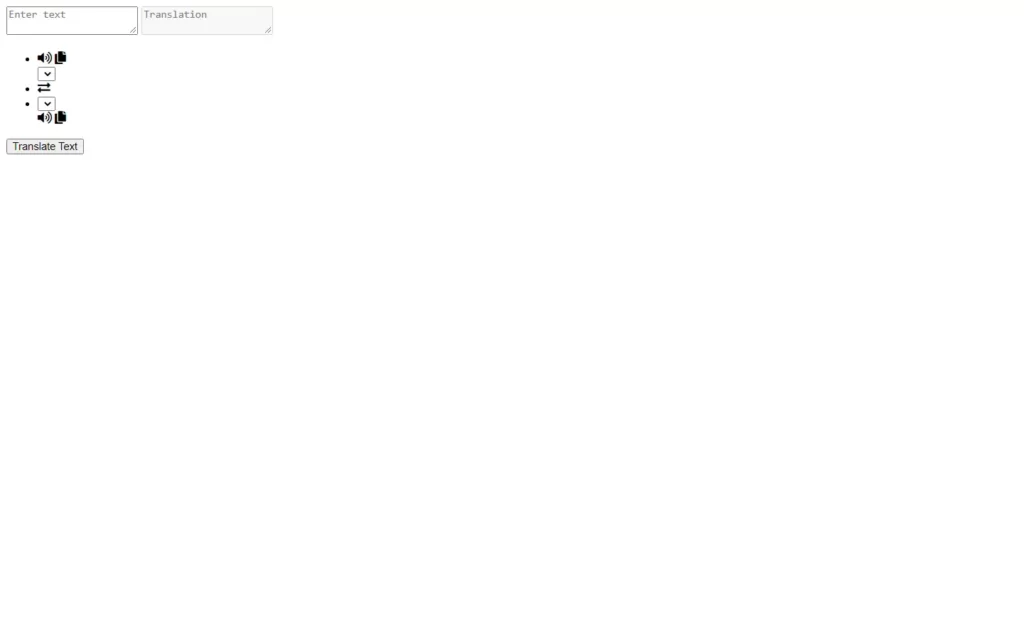
Customizing And Styling Our Project
So after adding the basic elements which is actually perfect, but we need to add some CSS styling so that our project looks a little bit good. For that we just added some background color, added a font family, and did some customizations to our elements as well as we centered our project, added some border, color, transitions etc. to make the project interactive. CSS styling is purely depending on the developer to give more interactive look, so we won’t discuss much about it.
All source code will be provided below, so you can simply copy and paste it.
Import Google Font - Poppins
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;700&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
align-items: center;
justify-content: center;
padding: 0 10px;
min-height: 100vh;
background: #5372F0;
}
.container{
max-width: 690px;
width: 100%;
padding: 30px;
background: #fff;
border-radius: 7px;
box-shadow: 0 10px 20px rgba(0,0,0,0.01);
}
.wrapper{
border-radius: 5px;
border: 1px solid #ccc;
}
.wrapper .text-input{
display: flex;
border-bottom: 1px solid #ccc;
}
.text-input .to-text{
border-radius: 0px;
border-left: 1px solid #ccc;
}
.text-input textarea{
height: 250px;
width: 100%;
border: none;
outline: none;
resize: none;
background: none;
font-size: 18px;
padding: 10px 15px;
border-radius: 5px;
}
.text-input textarea::placeholder{
color: #b7b6b6;
}
.controls, li, .icons, .icons i{
display: flex;
align-items: center;
justify-content: space-between;
}
.controls{
list-style: none;
padding: 12px 15px;
}
.controls .row .icons{
width: 38%;
}
.controls .row .icons i{
width: 50px;
color: #adadad;
font-size: 14px;
cursor: pointer;
transition: transform 0.2s ease;
justify-content: center;
}
.controls .row.from .icons{
padding-right: 15px;
border-right: 1px solid #ccc;
}
.controls .row.to .icons{
padding-left: 15px;
border-left: 1px solid #ccc;
}
.controls .row select{
color: #333;
border: none;
outline: none;
font-size: 18px;
background: none;
padding-left: 5px;
}
.text-input textarea::-webkit-scrollbar{
width: 4px;
}
.controls .row select::-webkit-scrollbar{
width: 8px;
}
.text-input textarea::-webkit-scrollbar-track,
.controls .row select::-webkit-scrollbar-track{
background: #fff;
}
.text-input textarea::-webkit-scrollbar-thumb{
background: #ddd;
border-radius: 8px;
}
.controls .row select::-webkit-scrollbar-thumb{
background: #999;
border-radius: 8px;
border-right: 2px solid #ffffff;
}
.controls .exchange{
color: #adadad;
cursor: pointer;
font-size: 16px;
transition: transform 0.2s ease;
}
.controls i:active{
transform: scale(0.9);
}
.container button{
width: 100%;
padding: 14px;
outline: none;
border: none;
color: #fff;
cursor: pointer;
margin-top: 20px;
font-size: 17px;
border-radius: 5px;
background: #5372F0;
}
@media (max-width: 660px){
.container{
padding: 20px;
}
.wrapper .text-input{
flex-direction: column;
}
.text-input .to-text{
border-left: 0px;
border-top: 1px solid #ccc;
}
.text-input textarea{
height: 200px;
}
.controls .row .icons{
display: none;
}
.container button{
padding: 13px;
font-size: 16px;
}
.controls .row select{
font-size: 16px;
}
.controls .exchange{
font-size: 14px;
}
}
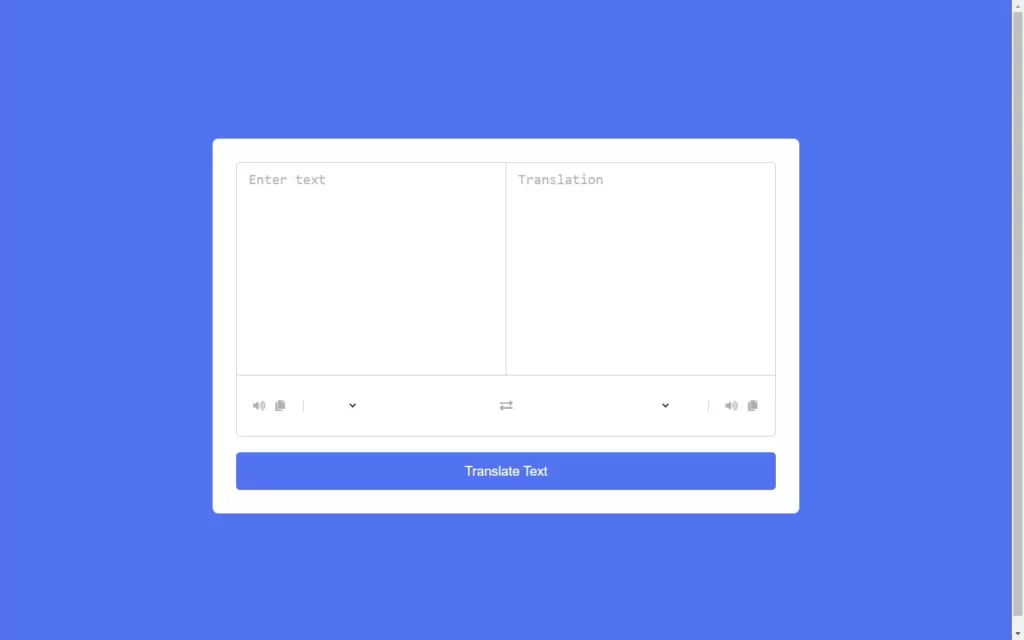
Adding JS Constant
Then we have also made another JS file in which we will add the country’s language names and country code. These country language names will be added in select menu later on.
const fromText = document.querySelector(".from-text"),
toText = document.querySelector(".to-text"),
exchageIcon = document.querySelector(".exchange"),
selectTag = document.querySelectorAll("select"),
icons = document.querySelectorAll(".row i");
translateBtn = document.querySelector("button"),
countries.js
const countries = {
"am-ET": "Amharic",
"ar-SA": "Arabic",
"be-BY": "Bielarus",
"bem-ZM": "Bemba",
"bi-VU": "Bislama",
"bjs-BB": "Bajan",
"bn-IN": "Bengali",
"bo-CN": "Tibetan",
"br-FR": "Breton",
"bs-BA": "Bosnian",
"ca-ES": "Catalan",
"cop-EG": "Coptic",
"cs-CZ": "Czech",
"cy-GB": "Welsh",
"da-DK": "Danish",
"dz-BT": "Dzongkha",
"de-DE": "German",
"dv-MV": "Maldivian",
"el-GR": "Greek",
"en-GB": "English",
"es-ES": "Spanish",
"et-EE": "Estonian",
"eu-ES": "Basque",
"fa-IR": "Persian",
"fi-FI": "Finnish",
"fn-FNG": "Fanagalo",
"fo-FO": "Faroese",
"fr-FR": "French",
"gl-ES": "Galician",
"gu-IN": "Gujarati",
"ha-NE": "Hausa",
"he-IL": "Hebrew",
"hi-IN": "Hindi",
"hr-HR": "Croatian",
"hu-HU": "Hungarian",
"id-ID": "Indonesian",
"is-IS": "Icelandic",
"it-IT": "Italian",
"ja-JP": "Japanese",
"kk-KZ": "Kazakh",
"km-KM": "Khmer",
"kn-IN": "Kannada",
"ko-KR": "Korean",
"ku-TR": "Kurdish",
"ky-KG": "Kyrgyz",
"la-VA": "Latin",
"lo-LA": "Lao",
"lv-LV": "Latvian",
"men-SL": "Mende",
"mg-MG": "Malagasy",
"mi-NZ": "Maori",
"ms-MY": "Malay",
"mt-MT": "Maltese",
"my-MM": "Burmese",
"ne-NP": "Nepali",
"niu-NU": "Niuean",
"nl-NL": "Dutch",
"no-NO": "Norwegian",
"ny-MW": "Nyanja",
"ur-PK": "Pakistani",
"pau-PW": "Palauan",
"pa-IN": "Panjabi",
"ps-PK": "Pashto",
"pis-SB": "Pijin",
"pl-PL": "Polish",
"pt-PT": "Portuguese",
"rn-BI": "Kirundi",
"ro-RO": "Romanian",
"ru-RU": "Russian",
"sg-CF": "Sango",
"si-LK": "Sinhala",
"sk-SK": "Slovak",
"sm-WS": "Samoan",
"sn-ZW": "Shona",
"so-SO": "Somali",
"sq-AL": "Albanian",
"sr-RS": "Serbian",
"sv-SE": "Swedish",
"sw-SZ": "Swahili",
"ta-LK": "Tamil",
"te-IN": "Telugu",
"tet-TL": "Tetum",
"tg-TJ": "Tajik",
"th-TH": "Thai",
"ti-TI": "Tigrinya",
"tk-TM": "Turkmen",
"tl-PH": "Tagalog",
"tn-BW": "Tswana",
"to-TO": "Tongan",
"tr-TR": "Turkish",
"uk-UA": "Ukrainian",
"uz-UZ": "Uzbek",
"vi-VN": "Vietnamese",
"wo-SN": "Wolof",
"xh-ZA": "Xhosa",
"yi-YD": "Yiddish",
"zu-ZA": "Zulu"
}
Adding Languages to Select Menus
Now, we will need to add languages to select menu, for that we are using for loop to enter every language. Then we have added this line of code let option = `<option ${selected} value="${country_code}">${countries[country_code]}</option>`;
to add options with value if country code and using array countries we will fetch the corresponding language name. Then after, we have made English language as selected option for input and Spanish language for output.
selectTag.forEach((tag, id) => {
for (let country_code in countries) {
let option = `<option ${selected} value="${country_code}">${countries[country_code]}</option>`;
let selected = id == 0 ? country_code == "en-GB" ? "selected" : "" : country_code == "es-ES" ? "selected" : "";
tag.insertAdjacentHTML("beforeend", option);
}
});
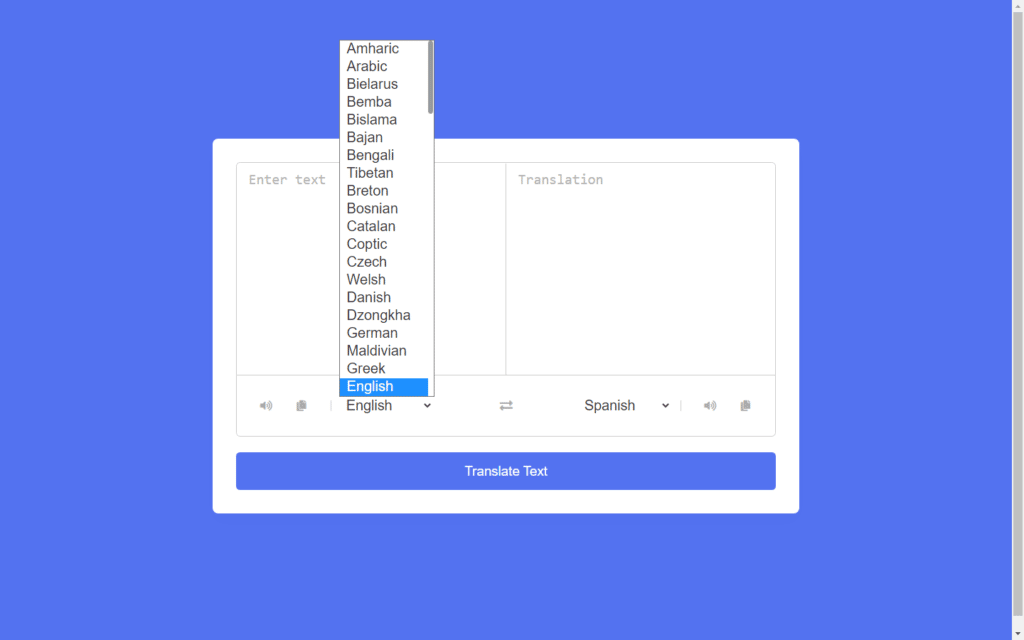
Adding Functionality to Exchange Icon
Now let’s move on to icons, first we will target our exchange icon. We have added an event listener for click event, here we have added a variable tempText in which we have assigned value of input area, then we have added selected language in tempLang variable. Then we have swapped the value of text areas and also swapped languages.
exchageIcon.addEventListener("click", () => {
let tempText = fromText.value,
tempLang = selectTag[0].value;
fromText.value = toText.value;
toText.value = tempText;
selectTag[0].value = selectTag[1].value;
selectTag[1].value = tempLang;
});
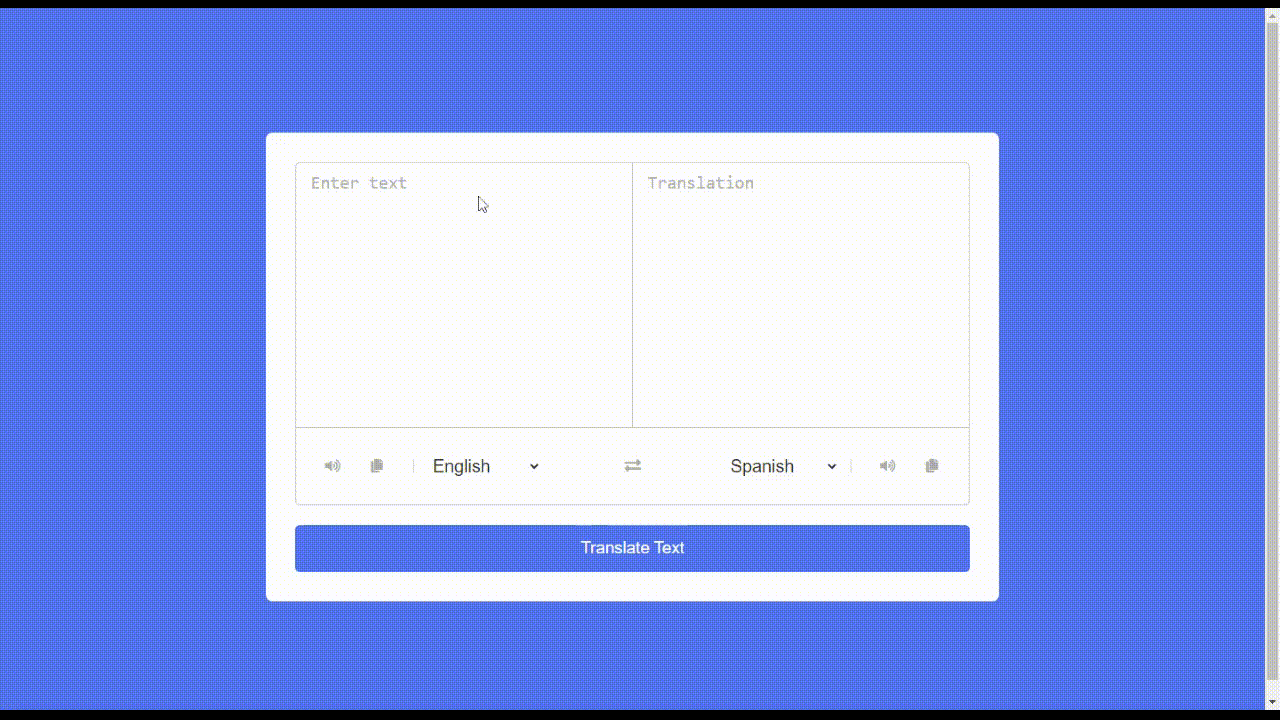
Adding Functionality to Other Icons
Now we have added a click event listener, we have added a condition to check copy button using target.classList.contains("fa-copy")
. Then if target.id is equal to from then we will copy the text using navigator.clipboard.writeText(fromText.value)
which is input field. Else we will copy text from output field. Now, for speech icon, we have added a variable named utterance. Here we have to again check target id to identify input area, if yes, then we have created an object of SpeechSynthesisUtterance() method. And using lang attribute we have assigned the selected language.
Else for output area, we have again created SpeechSynthesisUtterance() object. And again we have selected language indexed of 1 which language of output field.
icons.forEach(icon => {
icon.addEventListener("click", ({target}) => {
if(!fromText.value || !toText.value) return;
if(target.classList.contains("fa-copy")) {
if(target.id == "from") {
navigator.clipboard.writeText(fromText.value);
} else {
navigator.clipboard.writeText(toText.value);
}
} else {
let utterance;
if(target.id == "from") {
utterance = new SpeechSynthesisUtterance(fromText.value);
utterance.lang = selectTag[0].value;
} else {
utterance = new SpeechSynthesisUtterance(toText.value);
utterance.lang = selectTag[1].value;
}
speechSynthesis.speak(utterance);
}
});
});
Adding Functionality to Translate Button
Now for translation button, we have added a variable named text in which we will add text using fromText.value.trim()
method. Then we have assigned the selected languages to variables for input and output fields. We have changed the placeholder to translating using toText.setAttribute("placeholder", "Translating...");
. After that, we have added a API URL, in which text, translateFrom and translateTo will be variable and these will be dynamic. Then we have used fetch() to get data in JSON format, and then we have used data.responseData.translatedText
to get translated text to output area. Then we used data.translation to translate the data. Lastly, we will again change placeholder to translation.
translateBtn.addEventListener("click", () => {
let text = fromText.value.trim(),
translateFrom = selectTag[0].value,
translateTo = selectTag[1].value;
if(!text) return;
toText.setAttribute("placeholder", "Translating...");
let apiUrl = `https://api.mymemory.translated.net/get?q=${text}&langpair=${translateFrom}|${translateTo}`;
fetch(apiUrl).then(res => res.json()).then(data => {
toText.value = data.responseData.translatedText;
data.matches.forEach(data => {
if(data.id === 0) {
toText.value = data.translation;
}
});
toText.setAttribute("placeholder", "Translation");
});
});
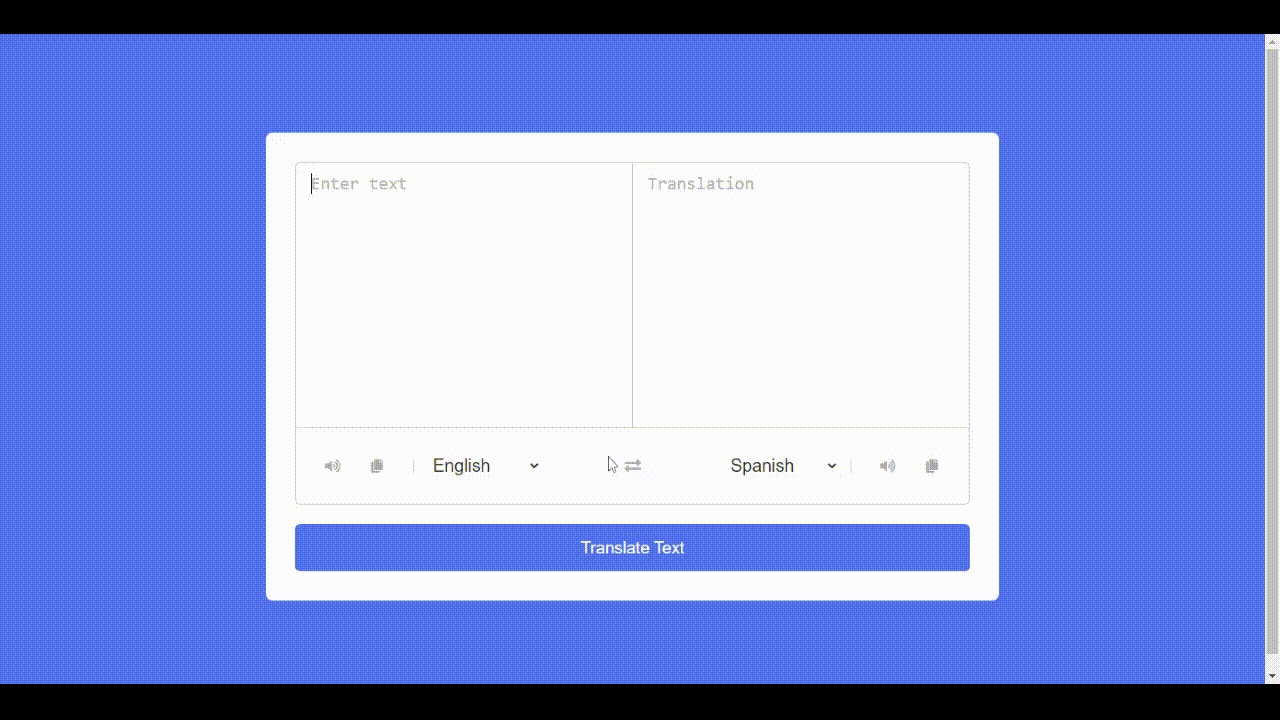
Full Source Code to Make Language Translator in JavaScript
index.html
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Language Translator</title>
<link rel="stylesheet" href="style.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Font Awesome CDN Link for Icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"/>
</head>
<body>
<div class="container">
<div class="wrapper">
<div class="text-input">
<textarea spellcheck="false" class="from-text" placeholder="Enter text"></textarea>
<textarea spellcheck="false" readonly disabled class="to-text" placeholder="Translation"></textarea>
</div>
<ul class="controls">
<li class="row from">
<div class="icons">
<i id="from" class="fas fa-volume-up"></i>
<i id="from" class="fas fa-copy"></i>
</div>
<select></select>
</li>
<li class="exchange"><i class="fas fa-exchange-alt"></i></li>
<li class="row to">
<select></select>
<div class="icons">
<i id="to" class="fas fa-volume-up"></i>
<i id="to" class="fas fa-copy"></i>
</div>
</li>
</ul>
</div>
<button>Translate Text</button>
</div>
<script src="js/countries.js"></script>
<script src="js/script.js"></script>
</body>
</html>
style.css
Import Google Font - Poppins
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;700&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
align-items: center;
justify-content: center;
padding: 0 10px;
min-height: 100vh;
background: #5372F0;
}
.container{
max-width: 690px;
width: 100%;
padding: 30px;
background: #fff;
border-radius: 7px;
box-shadow: 0 10px 20px rgba(0,0,0,0.01);
}
.wrapper{
border-radius: 5px;
border: 1px solid #ccc;
}
.wrapper .text-input{
display: flex;
border-bottom: 1px solid #ccc;
}
.text-input .to-text{
border-radius: 0px;
border-left: 1px solid #ccc;
}
.text-input textarea{
height: 250px;
width: 100%;
border: none;
outline: none;
resize: none;
background: none;
font-size: 18px;
padding: 10px 15px;
border-radius: 5px;
}
.text-input textarea::placeholder{
color: #b7b6b6;
}
.controls, li, .icons, .icons i{
display: flex;
align-items: center;
justify-content: space-between;
}
.controls{
list-style: none;
padding: 12px 15px;
}
.controls .row .icons{
width: 38%;
}
.controls .row .icons i{
width: 50px;
color: #adadad;
font-size: 14px;
cursor: pointer;
transition: transform 0.2s ease;
justify-content: center;
}
.controls .row.from .icons{
padding-right: 15px;
border-right: 1px solid #ccc;
}
.controls .row.to .icons{
padding-left: 15px;
border-left: 1px solid #ccc;
}
.controls .row select{
color: #333;
border: none;
outline: none;
font-size: 18px;
background: none;
padding-left: 5px;
}
.text-input textarea::-webkit-scrollbar{
width: 4px;
}
.controls .row select::-webkit-scrollbar{
width: 8px;
}
.text-input textarea::-webkit-scrollbar-track,
.controls .row select::-webkit-scrollbar-track{
background: #fff;
}
.text-input textarea::-webkit-scrollbar-thumb{
background: #ddd;
border-radius: 8px;
}
.controls .row select::-webkit-scrollbar-thumb{
background: #999;
border-radius: 8px;
border-right: 2px solid #ffffff;
}
.controls .exchange{
color: #adadad;
cursor: pointer;
font-size: 16px;
transition: transform 0.2s ease;
}
.controls i:active{
transform: scale(0.9);
}
.container button{
width: 100%;
padding: 14px;
outline: none;
border: none;
color: #fff;
cursor: pointer;
margin-top: 20px;
font-size: 17px;
border-radius: 5px;
background: #5372F0;
}
@media (max-width: 660px){
.container{
padding: 20px;
}
.wrapper .text-input{
flex-direction: column;
}
.text-input .to-text{
border-left: 0px;
border-top: 1px solid #ccc;
}
.text-input textarea{
height: 200px;
}
.controls .row .icons{
display: none;
}
.container button{
padding: 13px;
font-size: 16px;
}
.controls .row select{
font-size: 16px;
}
.controls .exchange{
font-size: 14px;
}
}
countries.js
const countries = {
"am-ET": "Amharic",
"ar-SA": "Arabic",
"be-BY": "Bielarus",
"bem-ZM": "Bemba",
"bi-VU": "Bislama",
"bjs-BB": "Bajan",
"bn-IN": "Bengali",
"bo-CN": "Tibetan",
"br-FR": "Breton",
"bs-BA": "Bosnian",
"ca-ES": "Catalan",
"cop-EG": "Coptic",
"cs-CZ": "Czech",
"cy-GB": "Welsh",
"da-DK": "Danish",
"dz-BT": "Dzongkha",
"de-DE": "German",
"dv-MV": "Maldivian",
"el-GR": "Greek",
"en-GB": "English",
"es-ES": "Spanish",
"et-EE": "Estonian",
"eu-ES": "Basque",
"fa-IR": "Persian",
"fi-FI": "Finnish",
"fn-FNG": "Fanagalo",
"fo-FO": "Faroese",
"fr-FR": "French",
"gl-ES": "Galician",
"gu-IN": "Gujarati",
"ha-NE": "Hausa",
"he-IL": "Hebrew",
"hi-IN": "Hindi",
"hr-HR": "Croatian",
"hu-HU": "Hungarian",
"id-ID": "Indonesian",
"is-IS": "Icelandic",
"it-IT": "Italian",
"ja-JP": "Japanese",
"kk-KZ": "Kazakh",
"km-KM": "Khmer",
"kn-IN": "Kannada",
"ko-KR": "Korean",
"ku-TR": "Kurdish",
"ky-KG": "Kyrgyz",
"la-VA": "Latin",
"lo-LA": "Lao",
"lv-LV": "Latvian",
"men-SL": "Mende",
"mg-MG": "Malagasy",
"mi-NZ": "Maori",
"ms-MY": "Malay",
"mt-MT": "Maltese",
"my-MM": "Burmese",
"ne-NP": "Nepali",
"niu-NU": "Niuean",
"nl-NL": "Dutch",
"no-NO": "Norwegian",
"ny-MW": "Nyanja",
"ur-PK": "Pakistani",
"pau-PW": "Palauan",
"pa-IN": "Panjabi",
"ps-PK": "Pashto",
"pis-SB": "Pijin",
"pl-PL": "Polish",
"pt-PT": "Portuguese",
"rn-BI": "Kirundi",
"ro-RO": "Romanian",
"ru-RU": "Russian",
"sg-CF": "Sango",
"si-LK": "Sinhala",
"sk-SK": "Slovak",
"sm-WS": "Samoan",
"sn-ZW": "Shona",
"so-SO": "Somali",
"sq-AL": "Albanian",
"sr-RS": "Serbian",
"sv-SE": "Swedish",
"sw-SZ": "Swahili",
"ta-LK": "Tamil",
"te-IN": "Telugu",
"tet-TL": "Tetum",
"tg-TJ": "Tajik",
"th-TH": "Thai",
"ti-TI": "Tigrinya",
"tk-TM": "Turkmen",
"tl-PH": "Tagalog",
"tn-BW": "Tswana",
"to-TO": "Tongan",
"tr-TR": "Turkish",
"uk-UA": "Ukrainian",
"uz-UZ": "Uzbek",
"vi-VN": "Vietnamese",
"wo-SN": "Wolof",
"xh-ZA": "Xhosa",
"yi-YD": "Yiddish",
"zu-ZA": "Zulu"
}
script.js
const fromText = document.querySelector(".from-text"),
toText = document.querySelector(".to-text"),
exchageIcon = document.querySelector(".exchange"),
selectTag = document.querySelectorAll("select"),
icons = document.querySelectorAll(".row i");
translateBtn = document.querySelector("button"),
selectTag.forEach((tag, id) => {
for (let country_code in countries) {
let selected = id == 0 ? country_code == "en-GB" ? "selected" : "" : country_code == "es-ES" ? "selected" : "";
let option = `<option ${selected} value="${country_code}">${countries[country_code]}</option>`;
tag.insertAdjacentHTML("beforeend", option);
}
});
exchageIcon.addEventListener("click", () => {
let tempText = fromText.value,
tempLang = selectTag[0].value;
fromText.value = toText.value;
toText.value = tempText;
selectTag[0].value = selectTag[1].value;
selectTag[1].value = tempLang;
});
fromText.addEventListener("keyup", () => {
if(!fromText.value) {
toText.value = "";
}
});
translateBtn.addEventListener("click", () => {
let text = fromText.value.trim(),
translateFrom = selectTag[0].value,
translateTo = selectTag[1].value;
if(!text) return;
toText.setAttribute("placeholder", "Translating...");
let apiUrl = `https://api.mymemory.translated.net/get?q=${text}&langpair=${translateFrom}|${translateTo}`;
fetch(apiUrl).then(res => res.json()).then(data => {
toText.value = data.responseData.translatedText;
data.matches.forEach(data => {
if(data.id === 0) {
toText.value = data.translation;
}
});
toText.setAttribute("placeholder", "Translation");
});
});
icons.forEach(icon => {
icon.addEventListener("click", ({target}) => {
if(!fromText.value || !toText.value) return;
if(target.classList.contains("fa-copy")) {
if(target.id == "from") {
navigator.clipboard.writeText(fromText.value);
} else {
navigator.clipboard.writeText(toText.value);
}
} else {
let utterance;
if(target.id == "from") {
utterance = new SpeechSynthesisUtterance(fromText.value);
utterance.lang = selectTag[0].value;
} else {
utterance = new SpeechSynthesisUtterance(toText.value);
utterance.lang = selectTag[1].value;
}
speechSynthesis.speak(utterance);
}
});
});
Output
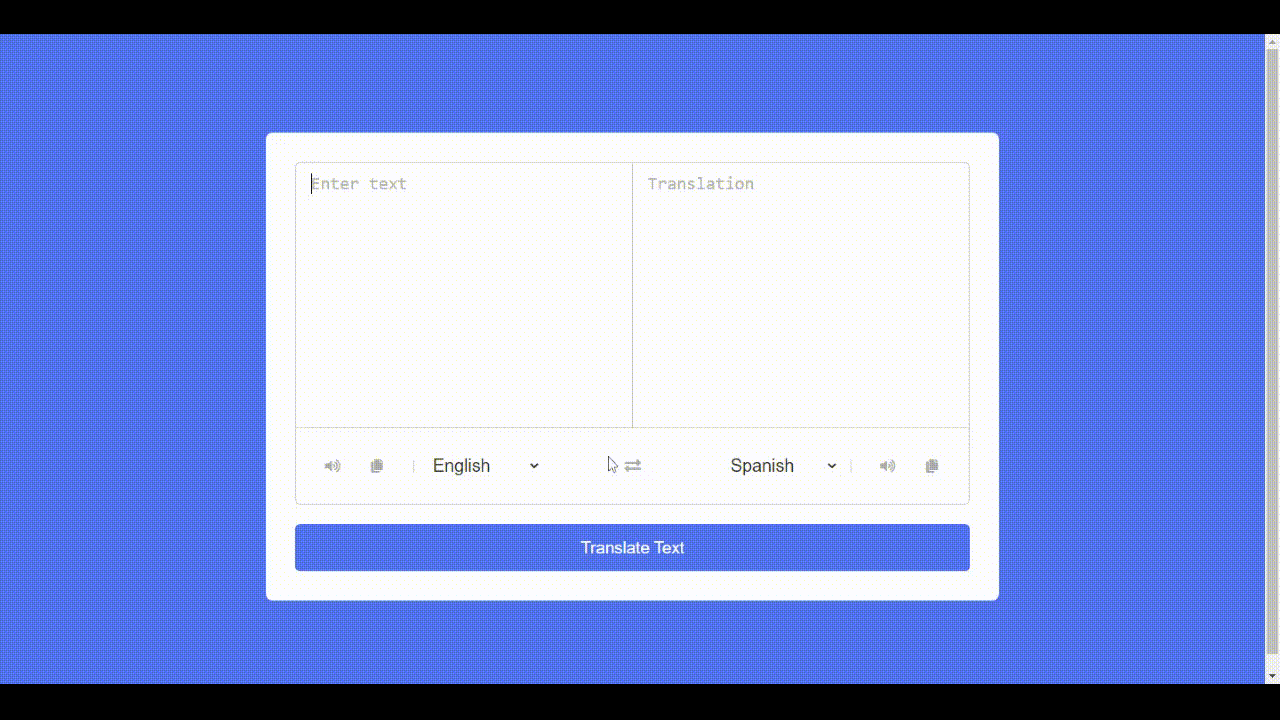
Check out awesome video reference here: