In this article, we will make a program to find the largest among three numbers. In this program we will have three numbers and among these numbers we will find out largest among them. We will get the result using two methods: (1) using Math.max() method (2) using user defined function.
Using Math.max()
Math.max()
method returns the largest number among the provided numbers. It is a simple JavaScript method which will be very useful, so you don’t need to write whole logic manually.
Syntax:
Math.max(n1,n2,n3,....,n)
So firstly, we will make an HTML markup as always. Then in this we have to add a script tag to write the JS code. You can also make a separate file for the JS and add the path in the HTML. Now in HTML we need to add 3 input fields for numbers and a button on which we applied onclick event with a function call named getresult
.
Now, in this function, we have fetched the values from the input fields to assign some variables using the document.getElementById().value
method. We have gave different IDs to these input fields, so they are easily accessible. Now we have added another variable, in which we have used a Math.max()
method, in which we added these variables to find out max value. And then we just printed the result.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="number" placeholder="enter first number" id="num1"/>
<input type="number" placeholder="enter second number" id="num2"/>
<input type="number" placeholder="enter third number" id="num3"/>
<button onclick=result()>get result</button>
<script>
function result(){
var a = document.getElementById("num1").value;
var b = document.getElementById("num2").value;
var c = document.getElementById("num3").value;
const res = Math.max(a,b,c);
document.write(`<h1>${res} is largest among the ${a} , ${b} , ${c}`)
}
</script>
</body>
</html>
Using User Defined Method
Since we have seen a very straight-forward and simple program to do this project. But we will make this from the scratch and with whole logic. For that, we have just copied above HTML code, and we need to modify the JS code.
Here we have some variables in which we have fetched the values from input fields same as above we’ve done, then we have added another function in which we will add the logic. In this function, we added conditions where we have compared the first value with second and third. If this condition becomes true, then return the first value. But if false then we will compare second value with first value and third value, if it is true then second value will be returned but if it is false then we will return the third value. Here we have used ‘&&’ operator which indicates if both conditions are true then and then if part will be executed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="number" placeholder="enter first number" id="num1"/>
<input type="number" placeholder="enter second number" id="num2"/>
<input type="number" placeholder="enter third number" id="num3"/>
<button onclick=result()>get result</button>
<script>
function result(){
var a = document.getElementById("num1").value;
var b = document.getElementById("num2").value;
var c = document.getElementById("num3").value;
function result(a,b,c){
if(a>b && a>c){
return a;
}
else if(b>c && b>a){
return b;
}
else{
return c;
}
}
const res = result(a,b,c);
document.write(`<h1>${res} is largest among the ${a} , ${b} , ${c}`)
}
</script>
</body>
</html>
Output
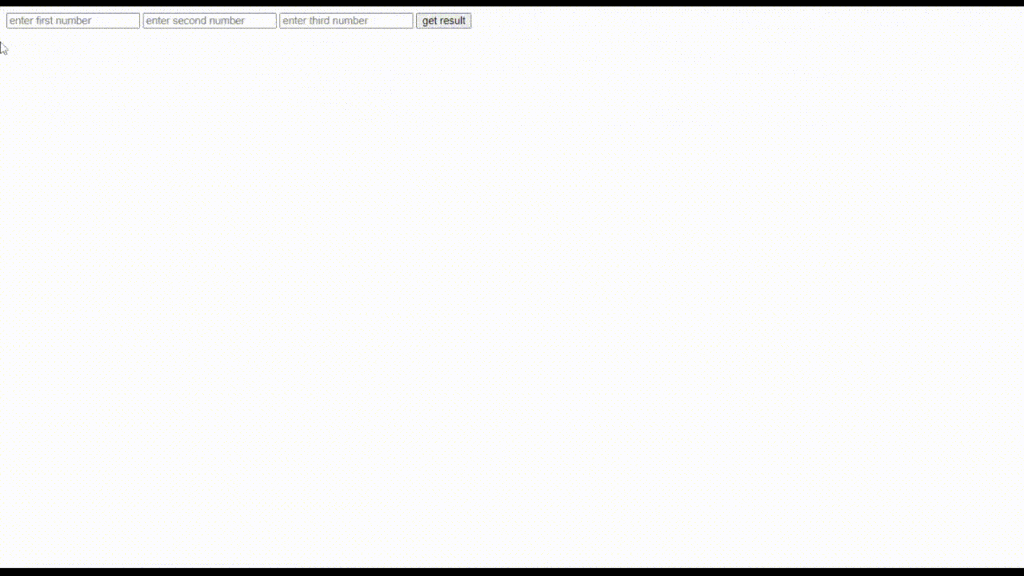