In this article, we will create a program to find the factorial of a number. In this program, we will get a number from the user, and we apply some logic to find out the factorial if the value is valid. We will get the result using two methods: (1) using normal loop, (2) using recursive function.
What is a Factorial of a Number?
So in mathematic, factorial of a number is the product or multiplication of all the numbers from 1 to that number. For example, if we want to find out factorial of 4 is equals to 4*3*2*1 = 24. Factorial of negative number cannot be possible and also factorial of 0 will be 1.
Using for Loop
So firstly, we will make an HTML markup as always. Then in this we have to add a script tag to write the JS code. You can also make a separate file for the JS and add the path in the HTML. In this HTML, we have added an input field to get the user’s input and a button, on which we have applied onclick event listener with a function call to get the result.
Now, in this function, we have declared a variable x in which we have to fetch the value using document.querySelector("num1")
to get value from the input value. Then we have added another variable in which we will store the value for factorial of a number. Now we have to check where the value is valid or not, so for that we applied a condition in which we are checking where x is equals to 0, if yes then we will print a message. If it is false, then we will move to the else part, in which we again check where value is negative or not. If yes then we print that factorial is not possible, and if false then we will again move to another else part.
In this, we will apply the logic to get factorial. For that we have added a for loop which will starts from 1 value and cycle till the inputted value. In this loop, we will just multiply the i with result and store in the result. Once the loop gets completed, then we print the result.
If you want to make it in JS only, then you can remove input field and button. And you can use prompt() to get the user’s value.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="num" id="num1"></input>
<button onclick= getres()>Result</button>
<script>
function getres(){
var x = document.querySelector("input").value;
var res = 1;
if (x==0){
document.write(`<h1> The factorial of ${x} is 1</h1>`);
}
else if(x < 0){
document.write(`<h1> The factorial of ${x} is not possible</h1>`);
}
else{
for(var i=1; i<=x; i++){
res = res * i;
}
document.write(`<h1>The factorial of ${x} will be ${res} </h1>`);
}
}
</script>
</body>
</html>
Using Recursive Function
Since we have seen a very straight-forward and simple program to do this project. But we will make this using recursive function, or you can say using recursion.
What is Recursion?
The process in which a function calls itself directly or indirectly is called recursion, and the corresponding function is called as recursive function.
Here we have some variables in which we have fetched the values from input fields same as above we’ve done, then we have added another function in which we will add the logic. In this function, we added conditions where we have compared the first value with second and third. If this condition becomes true, then return the first value. But if false then we will compare second value with first value and third value, if it is true then second value will be returned but if it is false then we will return the third value. Here we have used ‘&&’ operator which indicates if both conditions are true then and then if part will be executed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="number" placeholder="enter first number" id="num1"/>
<input type="number" placeholder="enter second number" id="num2"/>
<input type="number" placeholder="enter third number" id="num3"/>
<button onclick=result()>get result</button>
<script>
function result(){
var a = document.getElementById("num1").value;
var b = document.getElementById("num2").value;
var c = document.getElementById("num3").value;
function result(a,b,c){
if(a>b && a>c){
return a;
}
else if(b>c && b>a){
return b;
}
else{
return c;
}
}
const res = result(a,b,c);
document.write(`<h1>${res} is largest among the ${a} , ${b} , ${c}`)
}
</script>
</body>
</html>
Output
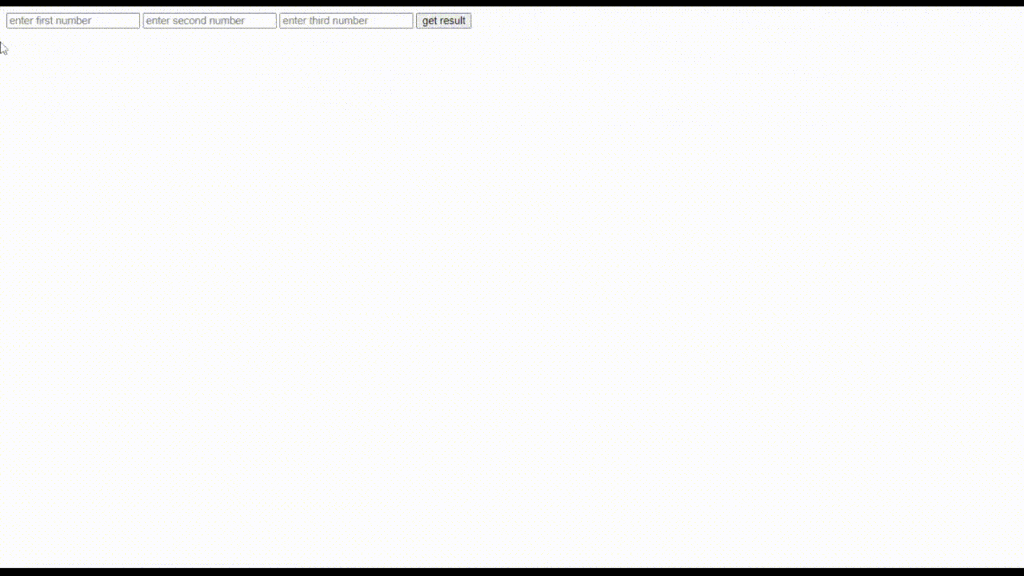