In this article, we are going to see how we to do Switching Name Order Using Capturing Groups in Regular Expressions. So we will have an array of name where we have some values, and we will use a regular expression to exchange their positions. There are multiple possibilities to make this thing happen, but we are going to see a very good method to get optimal solution.
Solution:
Okay now lets see the code solution, Here we created an array with some name, and we added a comma and space between these last names and first names. Here we are aiming to reverse this name in order of first name and last name. Then we applied map() method on this array, also we have added a callback function with name as parameter. As we know, map() is used to iterate through the array, and this will work as a loop. So in name parameter, all name strings pass through the name parameter.
Now we need to apply some logic on these names, so that we can get switched values in string. For that we used replace method where we applied a regular expression as a first parameter. To write regular expression, we will use //
and in between this (\w+), (\w+)
. First (\w+) will target first string’s word, and we used , (\w+)
to target second word, we need to apply comma and space in regular expression. We need to apply the same spaces and characters, so we can get all words. This thing known as capturing words.
Now after getting both words, we have added a second parameter, where we used $2 which will target second word then we added $1 which targets first word. This parameter works for switching parameter.
let namesArr = ["Doe, John", "Yeager, Eren", "Hales, Alex", "Peterson, Kevin"];
let newArr = namesArr.map((name) =>{
return name.replace(/(\w+), (\w+)/,"$2 $1");
});
console.log(newArr);
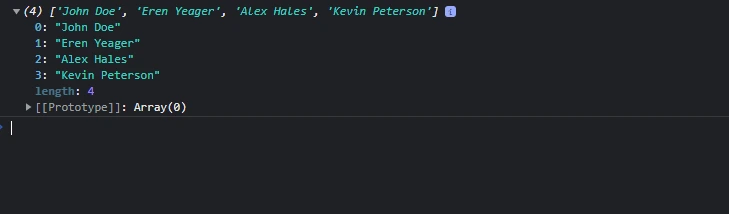