In this article, we will make a word scramble game in HTML, CSS & JavaScript. In this, we will provide some words along with a hint and the player needs to identify and guess the correct word as per the given hint. This project’s logic will be added using JavaScript.
This is going to be a beginner-friendly project and basic project. So let’s make it step-by-step.
Pre-requisite to Make Word Scramble Game in HTML, CSS & JavaScript
- Basic Knowledge of HTML.
- Basic Knowledge of CSS.
- Basic Knowledge of JavaScript.
Creating HTML Skeleton
For this project, we need to basically three files. First will be our index.html, in this we will add our elements, and you can simply say we will create the skeleton of the project using HTML file. Then for designing purpose we will be adding our style.css file, with this we will add some styles to our HTML, this is going to be purely based on you, like you can customize it any way. And lastly, our script.js file, this will be our main file because we will add functionality so that we can check where number or text is palindrome or not using the JavaScript file.
Now in HTML, we have added a container <div>, in which we have added a header with some text. And then we have added a paragraph to add hind and time span.Then we have to add an input field to get the input text with spell check to false. Lastly, we need a button to refresh word and also another button to check the guessed word with actual word. So this is it for our HTML.
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Word Scramble Game</title>
<link rel="stylesheet" href="style.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="js/words.js" defer></script>
<script src="js/script.js" defer></script>
</head>
<body>
<div class="container">
<h2>Word Scramble</h2>
<div class="content">
<p class="word"></p>
<div class="details">
<p class="hint">Hint: <span></span></p>
<p class="time">Time Left: <span><b>30</b>s</span></p>
</div>
<input type="text" spellcheck="false" placeholder="Enter a valid word">
<div class="buttons">
<button class="refresh-word">Refresh Word</button>
<button class="check-word">Check Word</button>
</div>
</div>
</div>
</body>
</html>
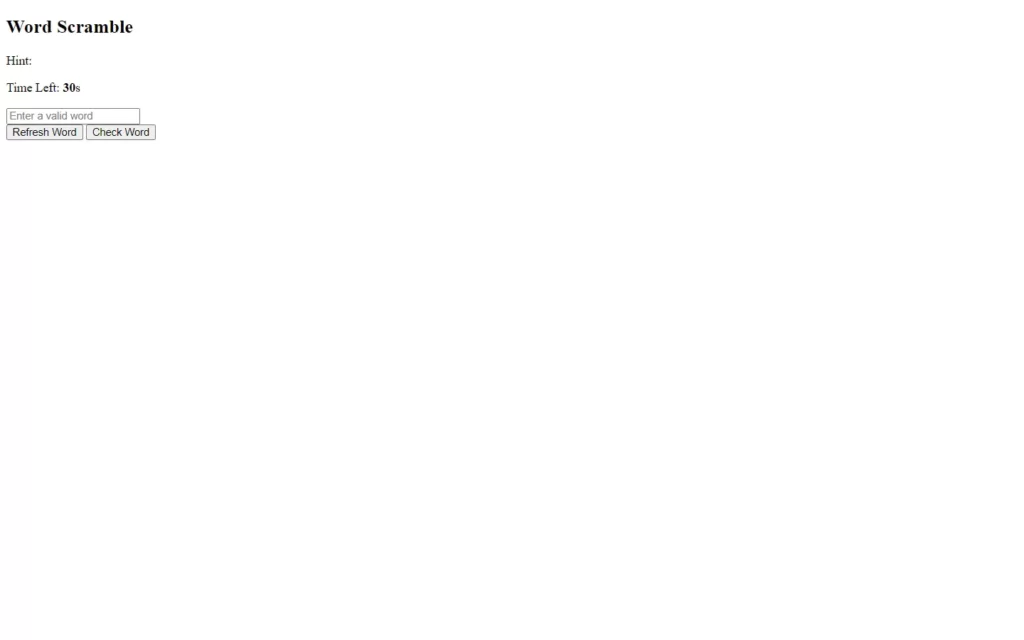
Customizing And Styling Our Project
So after adding the basic elements which is actually perfect, but we need to add some CSS styling so that our project looks a little bit good. For that we just added some background color, added a font family, and did some customizations to our elements as well as we centered our project, added some border, color, transitions etc. to make the project interactive. CSS styling is purely depending on the developer to give more interactive look, so we won’t discuss much about it.
All source code will be provided below, so you can simply copy and paste it.
/* Import Google font - Poppins */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
padding: 0 10px;
align-items: center;
justify-content: center;
min-height: 100vh;
background: #5372F0;
}
.container{
width: 440px;
border-radius: 7px;
background: #fff;
box-shadow: 0 10px 20px rgba(0,0,0,0.08);
}
.container h2{
font-size: 25px;
font-weight: 500;
padding: 16px 25px;
border-bottom: 1px solid #ccc;
}
.container .content{
margin: 25px 20px 35px;
}
.content .word{
user-select: none;
font-size: 33px;
font-weight: 500;
text-align: center;
letter-spacing: 24px;
margin-right: -24px;
word-break: break-all;
text-transform: uppercase;
}
.content .details{
margin: 25px 0 20px;
}
.details p{
font-size: 18px;
margin-bottom: 10px;
}
.details p b{
font-weight: 500;
}
.content input{
width: 100%;
height: 60px;
outline: none;
padding: 0 16px;
font-size: 18px;
border-radius: 5px;
border: 1px solid #bfbfbf;
}
.content input:focus{
box-shadow: 0px 2px 4px rgba(0,0,0,0.08);
}
.content input::placeholder{
color: #aaa;
}
.content input:focus::placeholder{
color: #bfbfbf;
}
.content .buttons{
display: flex;
margin-top: 20px;
justify-content: space-between;
}
.buttons button{
border: none;
outline: none;
color: #fff;
cursor: pointer;
padding: 15px 0;
font-size: 17px;
border-radius: 5px;
width: calc(100% / 2 - 8px);
transition: all 0.3s ease;
}
.buttons button:active{
transform: scale(0.97);
}
.buttons .refresh-word{
background: #6C757D;
}
.buttons .refresh-word:hover{
background: #5f666d;
}
.buttons .check-word{
background: #5372F0;
}
.buttons .check-word:hover{
background: #2c52ed;
}
@media screen and (max-width: 470px) {
.container h2{
font-size: 22px;
padding: 13px 20px;
}
.content .word{
font-size: 30px;
letter-spacing: 20px;
margin-right: -20px;
}
.container .content{
margin: 20px 20px 30px;
}
.details p{
font-size: 16px;
margin-bottom: 8px;
}
.content input{
height: 55px;
font-size: 17px;
}
.buttons button{
padding: 14px 0;
font-size: 16px;
width: calc(100% / 2 - 7px);
}
}
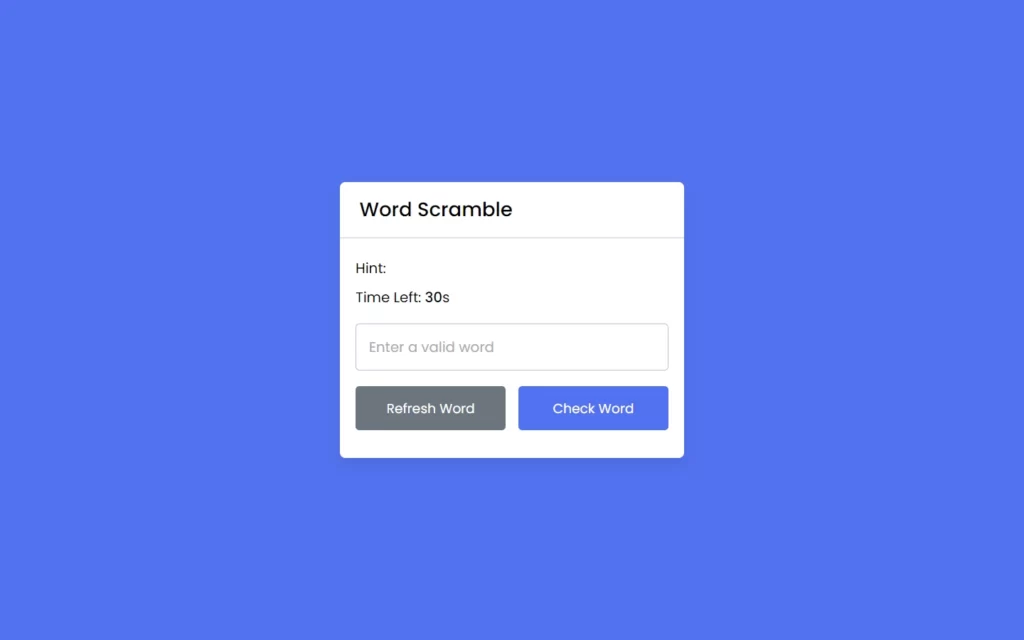
Adding JS Constant
const wordText = document.querySelector(".word"),
hintText = document.querySelector(".hint span"),
timeText = document.querySelector(".time b"),
inputField = document.querySelector("input"),
refreshBtn = document.querySelector(".refresh-word"),
checkBtn = document.querySelector(".check-word");
let correctWord, timer;
Adding Words and Hints
Now we will add a JS file in which we will add some words and hints as an array.
let words = [
{
word: "addition",
hint: "The process of adding numbers"
},
{
word: "meeting",
hint: "Event in which people come together"
},
{
word: "number",
hint: "Math symbol used for counting"
},
{
word: "exchange",
hint: "The act of trading"
},
{
word: "canvas",
hint: "Piece of fabric for oil painting"
},
{
word: "garden",
hint: "Space for planting flower and plant"
},
{
word: "position",
hint: "Location of someone or something"
},
{
word: "feather",
hint: "Hair like outer covering of bird"
},
{
word: "comfort",
hint: "A pleasant feeling of relaxation"
},
{
word: "tongue",
hint: "The muscular organ of mouth"
},
{
word: "expansion",
hint: "The process of increase or grow"
},
{
word: "country",
hint: "A politically identified region"
},
{
word: "group",
hint: "A number of objects or persons"
},
{
word: "taste",
hint: "Ability of tongue to detect flavour"
},
{
word: "store",
hint: "Large shop where goods are traded"
},
{
word: "field",
hint: "Area of land for farming activities"
},
{
word: "friend",
hint: "Person other than a family member"
},
{
word: "pocket",
hint: "A bag for carrying small items"
},
{
word: "needle",
hint: "A thin and sharp metal pin"
},
{
word: "expert",
hint: "Person with extensive knowledge"
},
{
word: "statement",
hint: "A declaration of something"
},
{
word: "second",
hint: "One-sixtieth of a minute"
},
{
word: "library",
hint: "Place containing collection of books"
},
]
Making Initial Timer
Now we will create a function to initialize and reduce timer. For that we have used the setInterval()
method to set the time, inside this we will check condition maxTime>0
then we will reduce the maxTime by 1, and we will return in innerText. Then we will alert time over once maxTime become 0. And then we will call the initGame()
function, in which we will initialize the game later. We need to reduce the timer every second for that we have provided 1000 in setInterval()
.
const initTimer = maxTime => {
clearInterval(timer);
timer = setInterval(() => {
if(maxTime > 0) {
maxTime--;
return timeText.innerText = maxTime;
}
alert(`Time off! ${correctWord.toUpperCase()} was the correct word`);
initGame();
}, 1000);
}
Initializing The Game
Now to initialize the game we have added a function for it. And in this, we have called the initTimer(30)
function with 30-second timer. Then we have called words, which is our array of words, on which we have applied Math.floor(Math.random() * words.length)
to get random word object. Then we have created wordArray to split each letter from the word using this line of code randomObj.word.split("")
.
Now we have added a for loop from word length to 1, in which we have added a variable with a random number using Math.floor(Math.random() * (i + 1));
. Now we will shuffle and swift letter randomly using [wordArray[i], wordArray[j]] = [wordArray[j], wordArray[i]];
.
After that, we will replace the word text with shuffled word using wordArray.join("")
. Then we have fetched a hint for the word using hintText.innerText = randomObj.hint
. Then we have turned word to lowercase word, and we have cleared input field also we have set maxLength with correctWord’s length.
const initGame = () => {
initTimer(30);
let randomObj = words[Math.floor(Math.random() * words.length)];
let wordArray = randomObj.word.split("");
for (let i = wordArray.length - 1; i > 0; i--) {
let j = Math.floor(Math.random() * (i + 1));
[wordArray[i], wordArray[j]] = [wordArray[j], wordArray[i]];
}
wordText.innerText = wordArray.join("");
hintText.innerText = randomObj.hint;
correctWord = randomObj.word.toLowerCase();;
inputField.value = "";
inputField.setAttribute("maxlength", correctWord.length);
}
initGame();
Checking The Word’s Correctness
Now we need to check where the guessed word is correct or not, for that we have added a function, in which we have fetched the value from the input field, and then we have added a condition. In which, if the guessed word doesn’t match with the actual word then player will lose, otherwise player wins. Then we have added event listener on refresh button to call initGame()
again and on check button we will call checkWord()
function to check the result.
const checkWord = () => {
let userWord = inputField.value.toLowerCase();
if(!userWord) return alert("Please enter the word to check!");
if(userWord !== correctWord) return alert(`Oops! ${userWord} is not a correct word`);
alert(`Congrats! ${correctWord.toUpperCase()} is the correct word`);
initGame();
}
refreshBtn.addEventListener("click", initGame);
checkBtn.addEventListener("click", checkWord);
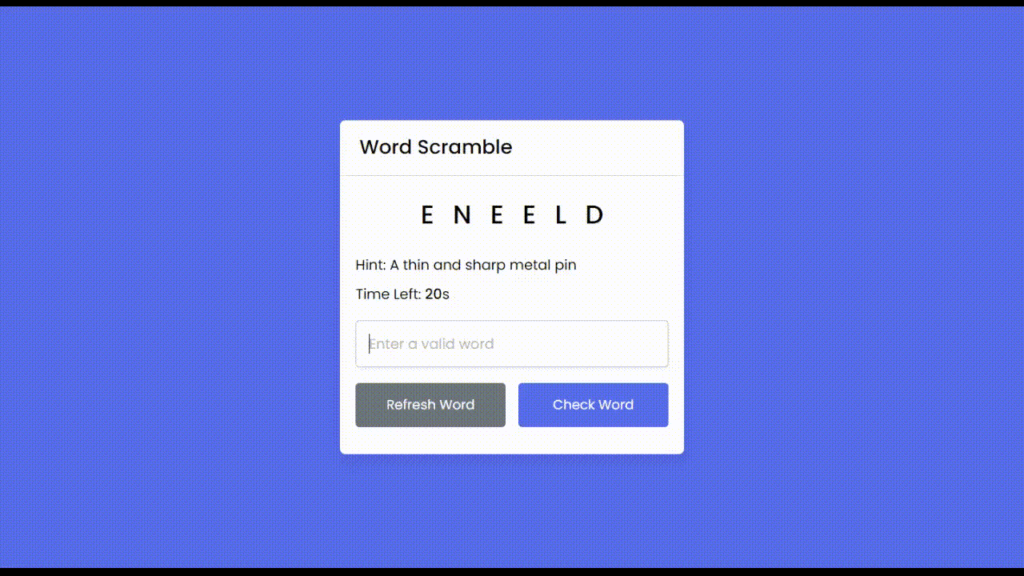
Full Source Code to Make Word Scramble Game in HTML, CSS & JavaScript
index.html
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Word Scramble Game</title>
<link rel="stylesheet" href="style.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="js/words.js" defer></script>
<script src="js/script.js" defer></script>
</head>
<body>
<div class="container">
<h2>Word Scramble</h2>
<div class="content">
<p class="word"></p>
<div class="details">
<p class="hint">Hint: <span></span></p>
<p class="time">Time Left: <span><b>30</b>s</span></p>
</div>
<input type="text" spellcheck="false" placeholder="Enter a valid word">
<div class="buttons">
<button class="refresh-word">Refresh Word</button>
<button class="check-word">Check Word</button>
</div>
</div>
</div>
</body>
</html>
style.css
/* Import Google font - Poppins */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
padding: 0 10px;
align-items: center;
justify-content: center;
min-height: 100vh;
background: #5372F0;
}
.container{
width: 440px;
border-radius: 7px;
background: #fff;
box-shadow: 0 10px 20px rgba(0,0,0,0.08);
}
.container h2{
font-size: 25px;
font-weight: 500;
padding: 16px 25px;
border-bottom: 1px solid #ccc;
}
.container .content{
margin: 25px 20px 35px;
}
.content .word{
user-select: none;
font-size: 33px;
font-weight: 500;
text-align: center;
letter-spacing: 24px;
margin-right: -24px;
word-break: break-all;
text-transform: uppercase;
}
.content .details{
margin: 25px 0 20px;
}
.details p{
font-size: 18px;
margin-bottom: 10px;
}
.details p b{
font-weight: 500;
}
.content input{
width: 100%;
height: 60px;
outline: none;
padding: 0 16px;
font-size: 18px;
border-radius: 5px;
border: 1px solid #bfbfbf;
}
.content input:focus{
box-shadow: 0px 2px 4px rgba(0,0,0,0.08);
}
.content input::placeholder{
color: #aaa;
}
.content input:focus::placeholder{
color: #bfbfbf;
}
.content .buttons{
display: flex;
margin-top: 20px;
justify-content: space-between;
}
.buttons button{
border: none;
outline: none;
color: #fff;
cursor: pointer;
padding: 15px 0;
font-size: 17px;
border-radius: 5px;
width: calc(100% / 2 - 8px);
transition: all 0.3s ease;
}
.buttons button:active{
transform: scale(0.97);
}
.buttons .refresh-word{
background: #6C757D;
}
.buttons .refresh-word:hover{
background: #5f666d;
}
.buttons .check-word{
background: #5372F0;
}
.buttons .check-word:hover{
background: #2c52ed;
}
@media screen and (max-width: 470px) {
.container h2{
font-size: 22px;
padding: 13px 20px;
}
.content .word{
font-size: 30px;
letter-spacing: 20px;
margin-right: -20px;
}
.container .content{
margin: 20px 20px 30px;
}
.details p{
font-size: 16px;
margin-bottom: 8px;
}
.content input{
height: 55px;
font-size: 17px;
}
.buttons button{
padding: 14px 0;
font-size: 16px;
width: calc(100% / 2 - 7px);
}
}
script.js
const wordText = document.querySelector(".word"),
hintText = document.querySelector(".hint span"),
timeText = document.querySelector(".time b"),
inputField = document.querySelector("input"),
refreshBtn = document.querySelector(".refresh-word"),
checkBtn = document.querySelector(".check-word");
let correctWord, timer;
const initTimer = maxTime => {
clearInterval(timer);
timer = setInterval(() => {
if(maxTime > 0) {
maxTime--;
return timeText.innerText = maxTime;
}
alert(`Time off! ${correctWord.toUpperCase()} was the correct word`);
initGame();
}, 1000);
}
const initGame = () => {
initTimer(30);
let randomObj = words[Math.floor(Math.random() * words.length)];
let wordArray = randomObj.word.split("");
for (let i = wordArray.length - 1; i > 0; i--) {
let j = Math.floor(Math.random() * (i + 1));
[wordArray[i], wordArray[j]] = [wordArray[j], wordArray[i]];
}
wordText.innerText = wordArray.join("");
hintText.innerText = randomObj.hint;
correctWord = randomObj.word.toLowerCase();;
inputField.value = "";
inputField.setAttribute("maxlength", correctWord.length);
}
initGame();
const checkWord = () => {
let userWord = inputField.value.toLowerCase();
if(!userWord) return alert("Please enter the word to check!");
if(userWord !== correctWord) return alert(`Oops! ${userWord} is not a correct word`);
alert(`Congrats! ${correctWord.toUpperCase()} is the correct word`);
initGame();
}
refreshBtn.addEventListener("click", initGame);
checkBtn.addEventListener("click", checkWord);
words.js
let words = [
{
word: "addition",
hint: "The process of adding numbers"
},
{
word: "meeting",
hint: "Event in which people come together"
},
{
word: "number",
hint: "Math symbol used for counting"
},
{
word: "exchange",
hint: "The act of trading"
},
{
word: "canvas",
hint: "Piece of fabric for oil painting"
},
{
word: "garden",
hint: "Space for planting flower and plant"
},
{
word: "position",
hint: "Location of someone or something"
},
{
word: "feather",
hint: "Hair like outer covering of bird"
},
{
word: "comfort",
hint: "A pleasant feeling of relaxation"
},
{
word: "tongue",
hint: "The muscular organ of mouth"
},
{
word: "expansion",
hint: "The process of increase or grow"
},
{
word: "country",
hint: "A politically identified region"
},
{
word: "group",
hint: "A number of objects or persons"
},
{
word: "taste",
hint: "Ability of tongue to detect flavour"
},
{
word: "store",
hint: "Large shop where goods are traded"
},
{
word: "field",
hint: "Area of land for farming activities"
},
{
word: "friend",
hint: "Person other than a family member"
},
{
word: "pocket",
hint: "A bag for carrying small items"
},
{
word: "needle",
hint: "A thin and sharp metal pin"
},
{
word: "expert",
hint: "Person with extensive knowledge"
},
{
word: "statement",
hint: "A declaration of something"
},
{
word: "second",
hint: "One-sixtieth of a minute"
},
{
word: "library",
hint: "Place containing collection of books"
},
]
Output
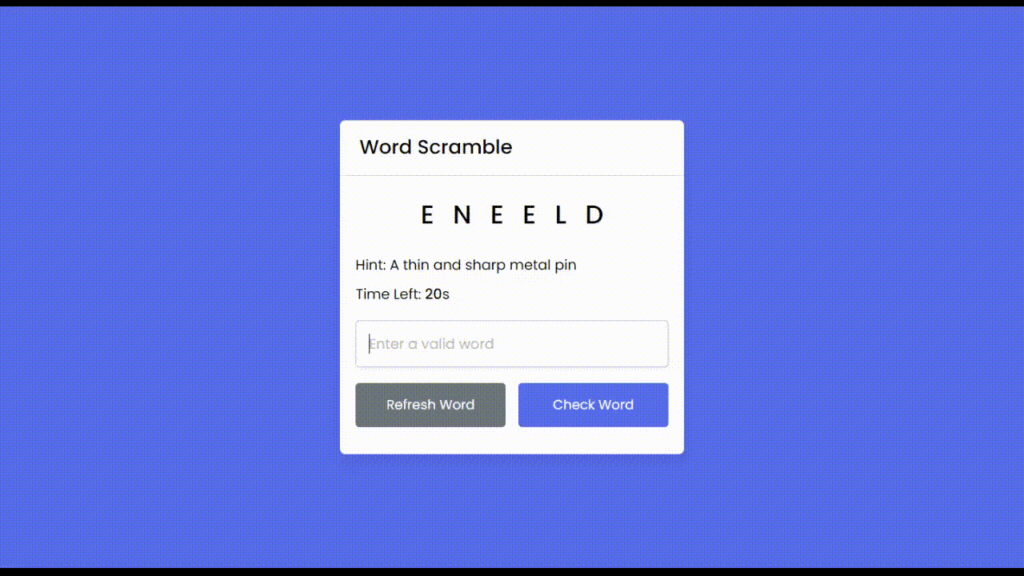
Check out awesome video reference here: