In this article, we will make a program to check if a number is a prime number or not. So basically, we will have an input field in which we will get the number from the user then we also have a button, by clicking it we will run this program and get the result for the input. We will make this in 2 ways, one with pure JS code and another one with the help of little HTML code.
What is a Prime Number?
So before going to the code, let’s understand the logic first. Prime numbers are those numbers that are only divisible by 1 and the number itself. For example, 13 is only divisible by 1 and 13 only. In this program, we will just get the remainder to compare with 0. So the remainder will be 0 only if we divide the number by 1 and 13.
Using Pure JS Code
So firstly, we have made an HTML markup in order to run JS code. In that, we have a script tag for JS code, Here, we have added a variable x with the prompt message to get the input value. Then we have checked a condition to where the number is 1, if yes then we have printed a message that it is not a prime nor composite. If not, then we have checked another condition to check where the provided number is below 1 then we have printed that the number is not a prime.
If both conditions are false, then we have added an else part, in which we have added a for a loop. In this loop, we have declared a variable ‘i’ with an initial value of 2. This loop will run to the provided value, like if we provide 10 then 9 will be the last value for ‘i’. In this loop, we will check the condition where if the number returns 0 remainders, if yes then it won’t be a prime number. And if no then we will again loop this with an increased value of ‘i’ to check again the same condition.
Here if every time the else part gets executed then the number will prime. If not then it is not a prime number.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<script type="text/javascript">
var x = prompt("Please Enter The Number:");
if (x == 1){
document.write(`${x} is neither prime not composite`);
}
else if(x < 1){
document.write(`${x} is not a prime number`);
}
else{
for(var i=2; i < x; i++){
if(x % i ==0){
var res = `${x} is not a prime number`;
}
else{
var res = `${x} is a prime number`;
}
}
document.write(`<h1> ${res} </h1>`);
}
</script>
</body>
</html>
Using HTML Element
In this, we will apply the same logic, but we will get the value from the user inside the input field which is an HTML element. Also, we will provide a button on which we need to add an event listener to get the output. As we can see, we have some increased code, but it is necessary to learn in order to learn JavaScript and its events.
So here we just fetched the value from the input value from the user using document.querySelector().value the method. This is useful when we need to manipulate or update an element from the DOM. On the button, we have added an event listener for the click event, in which we will run a function when it gets clicked. You can see we have created a function and put the whole logic in it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<input type="number" />
<button onclick=result>Result</button>
<script type="text/javascript">
function result(){
var x = document.querySelector("input").value;
if (x == 1){
document.write(`${x} is neither prime not composite`);
}
else if(x < 1){
document.write(`${x} is not a prime number`);
}
else{
for(var i=2; i < x; i++){
if(x % i ==0){
var res = `${x} is not a prime number`;
}
else{
var res = `${x} is a prime number`;
}
}
document.write(`<h1> ${res} </h1>`);
}
}
</script>
</body>
</html>
Output
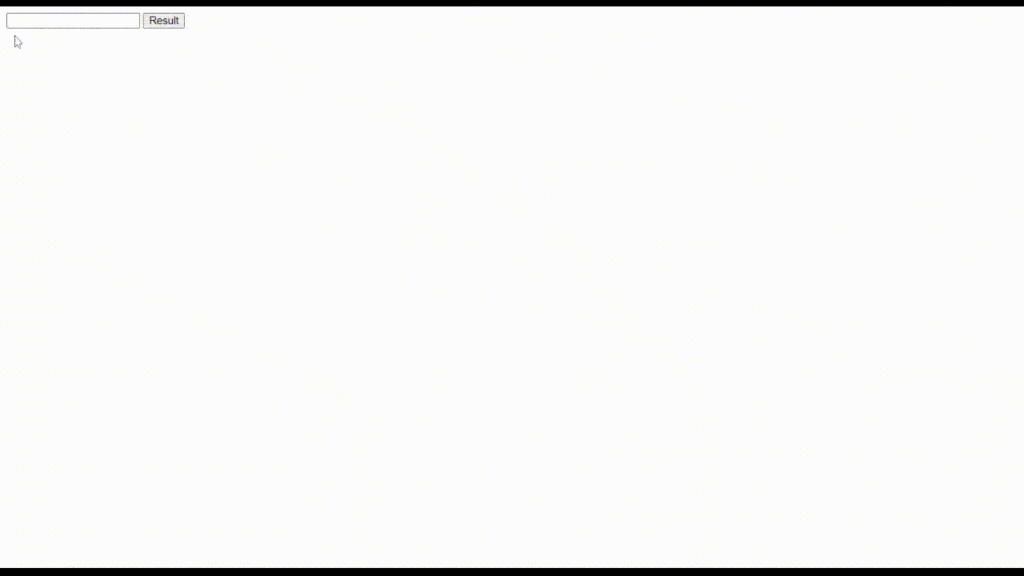