In this article, we will make two programs to convert kilometers to miles and Celsius to Fahrenheit. These will be very easy program and also beginner-friendly. So we will try to learn these programs with easier explanation. So let’s create these programs one by one.
So firstly we will make a convertor to convert kilometers to miles, and then we will see Celsius to Fahrenheit convertor.
Kilometer to Miles Convertor
In this firstly, we will add the HTML file as always, and we will use some HTML elements to get the user’s value. Here we have added an input field with number type, and we provided data as ID for this input field. Then we have added a button for convert, we also provided an event listener “onclick” in which we have added a function named convert(), which we will define in the JavaScript code. Here convert() function will trigger when we will click on the button, event listener listens the click event and call the provided function.
Now we also, added a <h1> with “res” ID, so we will add the result in this.
Let’s move to JS part, we have defined a function same name as we provided on the button. In this, we have declared a variable “kms” in which we have fetched input field value with its ID “data”. We have used document.getElementById('data')
to target input field which have “data” ID. We used document.getElementById('data').value
to get the value inside the input field. Now we have added a constant factor with the value 0.621371, this is basically, a value when we convert 1 km to miles. Now we have multiplied the factor and “kms” values, and store in the miles variable.
After that, we have fetched the “res” ID using document.getElementById('res')
, and we have added the values of miles into the DOM using innerText
property. In this, we have used ${miles}
to write the value directly.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Addimg two numbers</title>
</head>
<body>
<input type="number" id="data" step="any"/>Kms
<button onclick="convert()">convert</button>
<h1 id = "res"></h1>
<script>
function convert(){
var kms = document.getElementById('data').value;
const factor = 0.621371;
var miles = kms * factor;
document.getElementById('res').innerText = `${miles}`+ "miles";
}
</script>
</body>
</html>
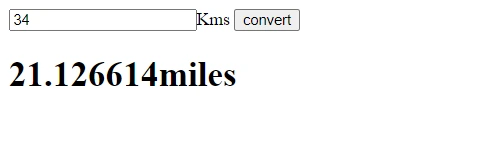
Celsius to Fahrenheit Convertor
In this firstly, we will add the HTML file as always, and we will use some HTML elements to get the user’s value. Here we have added an input field with number type, and we provided data as ID for this input field. Then we have added a button for convert, we also provided an event listener “onclick” in which we have added a function named convert(), which we will define in the JavaScript code. Here convert() function will trigger when we will click on the button, event listener listens the click event and call the provided function.
Now we also, added a <h1> with “res” ID, so we will add the result in this.
Let’s move to JS part, we have defined a function same name as we provided on the button. In this, we have declared a variable “C” in which we have fetched input field value with its ID “data”. We have used document.getElementById('data')
to target input field which have “data” ID. We used document.getElementById('data').value
to get the value inside the input field. Now we have declared a variable “F” in which we have added a formula to convert Celsius to Fahrenheit.
After that, we have fetched the “res” ID using document.getElementById('res')
, and we have added the values of miles into the DOM using innerText
property. In this, we have used ${miles}
to write the value directly.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Addimg two numbers</title>
</head>
<body>
<input type="number" id="data" step="any"/>C
<button onclick="convert()">convert</button>
<h1 id = "res"></h1>
<script>
function convert(){
var C = document.getElementById('data').value;
var F = (C * 1.8) + 32;
document.getElementById('res').innerText = `${F}`+ "F";
}
</script>
</body>
</html>
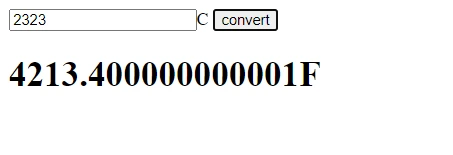