Today, we will learn about positioning in CSS. let’s look at how to use CSS to specify the arrangement of these boxes on our web pages. In this topic, we’ll be looking at two of the most important concepts in CSS: positioning and floating. Together, these two properties handle the layout of our web pages.
Positioning in CSS
The CSS positioning property allows you to position an element and specify which element should be on top in case of an overlap. There are four methods to position an element. To understand how the four methods work, I strongly encourage you to try out the exercise at the end of this chapter. This is a topic that is difficult to understand without a hands-on approach.
Static Positioning in CSS
The first positioning method is static positioning. Static doesn’t mean much, it just means that the elements are positioned according to the normal flow of the page. All HTML elements are positioned using this method by default. If you want to specify that static positioning be used (for instance to override another positioning rule for the same element), you write position: static;
Relative Positioning in CSS
The second method to position an element is the relative positioning method. This method positions an element relative to its normal position. Normal position refers to the default position of the element when no positioning rule is specified or when static positioning is used.
Suppose we have two boxes, box1 and box2 with no positioning specified. If we create box2 after box1 in our HTML code, box2 will be positioned below box1 by default (refer to code below).
<!DOCTYPE html>
<html>
<head>
<style>
#box1 {
/*Some rules for styling box1*/
}
#box2 {
/*Some rules for styling box2*/
}
</style>
</head>
<body>
<div id="box1">Box 1</div>
<div id="box2">Box 2</div>
</body>
</html>
Now suppose we add the following rules to the CSS declaration of box2.
position: relative;
left: 150px;
top: 50px;
What we’ve done is change the positioning of box2 to relative positioning.
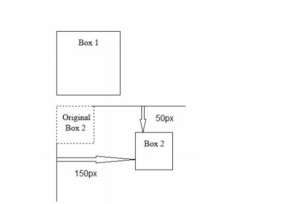
The image above shows the position of box2 when relative positioning is used. A white dotted box is added to show the original position of box2.
When relative positioning is applied, box2 is moved relative to its normal position. The line top: 50px; moves the box 50px away from the top of the original position and the line left: 150px; moves it 150px away from the left.
You can also use the right and bottom properties to position box2. For instance, bottom: 50px; will move box2 50px up from the original bottom of box2 while right: 50px; will move it 50px left from the original right edge of box2. In addition, you can use negative pixels to position the boxes.
Note that when relative positioning is used, the element can end up overlapping other elements.
If you want to specify which element should be in front, you have to use the z-index property. The z-index property works on any element that has a position of either relative, fixed, or absolute (i.e. not static). An element with a greater z-index value will be positioned in front of an element with a lower z-index value.
Suppose you have two boxes, greenBox and redBox, and they overlap each other. If you declare the z-index of greenBox as z-index: 1;
and the z-index of redBox as z-index: 2;
redBox will be on top of greenBox as it has a greater z-index value
Fixed Positioning in CSS
The third positioning method is fixed positioning. As the name suggests, an element that is positioned using the fixed method will always stay at its assigned location; it will not move even when the page is scrolled. Fixed positioning is commonly used to position social sharing buttons at the side of a web page. To use fixed positioning, we write position: fixed;
When using fixed positioning, we can use the top property to specify the number of pixels the box should be from the top of the page while the left property specifies the number of pixels it should be from the left of the page.
In addition to top and left, there are also the right and bottom properties, which specify the number of pixels the box should be from the right side and bottom of the page respectively.
Absolute Positioning in CSS
The final method is absolute positioning.
When absolute positioning is used, an element is positioned relative to the first parent element that has a position other than static. If no such element is found, it is positioned relative to the page.
For instance, suppose we have the following HTML code:
<div id="box1">Content in Box 1</div>
<div id="box2">Content in Box 2</div>
and the following CSS declaration:
#box1 {
position: relative;
}
#box2 {
position: absolute;
top: 50px;
left: 150px;
}
Assuming that box2 is not a child element of any element that has a non static positioning, it’ll be positioned relative to the page. That is, it is positioned 50px from the top of the page and 150px from the left.