In this article, we will make a program to print hello world using JavaScript. As we know that, in every language, printing “hello world” is the first program. As a beginner of JavaScript, This is going to be our first ever program, or you can say this will be our first step into JavaScript.
We will complete this program with using three ways:
console.log()
alert()
document.write()
These all are also known as JavaScript methods, for simplicity you can call them functions as well. These methods are not the same at anyhow, but we will use these, so you can identify the differences between them.
Using console.log()
Firstly, let me tell you that, you can’t use JavaScript methods directly you need HTML file as a base with which you can do this program perfectly. So just make a little program of HTML in which we will add the JavaScript code within the <script> tag.
console.log()
is used in debugging the code. In most of the JavaScript programs, we can add console.log()
to debug the code to check out flow of the program.
Syntax:
console.log("your message")
<html>
<body>
<script>
console.log("Hello World!!!")
</script>
</body>
</html>
Here, we have added a basic HTML code, in this we just added script tag to write JavaScript code. There are other ways as well to add JavaScript to HTML, of course. In console.log
, we can add our message within the quotation(“ “).
You can see the output in the browser, by going into inspect element>console.

Using Alert()
alert()
method is used to show the alert message in the alert box in the current window.
Syntax:
alert("your message")
In here, we just replaced the console.log with alert, using alert()
method, we can see the differences in outputs. In alert()
, we can see that alert box show up as soon as we run the program here.
<html>
<body>
<script>
alert("Hello World!!!")
</script>
</body>
</html>

Using document.write()
Okay, we have seen two methods to print hello world, but if you have noticed the output of above methods, we didn’t print the message on the main window.
document.write()
is used when you want to print the content to the HTML document, simply on the main tab.
Syntax:
document.write("Hello World!!!")
In here, document is the variable of the DOM, or HTML document, and it targets the HTML document. It is actually a pre-defined variable in JavaScript.
write()
is the method in which we have to write our message. As its name suggests that it just print the things inside of it.
<html>
<body>
<script>
document.write("Hello World!!!")
</script>
</body>
</html>
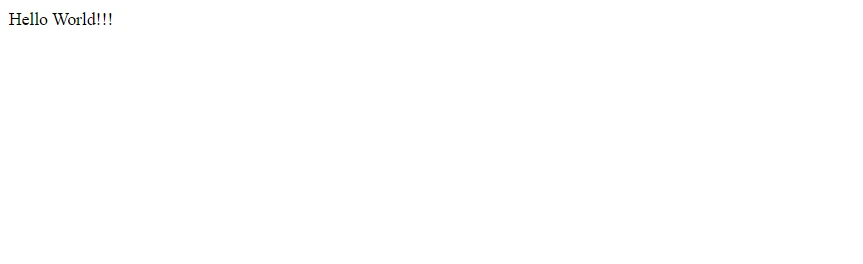