In this article, we will see how to make instagram signup page In HTML and CSS. We will just use HTML and CSS/CSS3 only to create this page. This page will have some images, instagram logo, and of course one signup form. It will be pretty good and interesting form to create, also we will use little bit of bootstrap just for some buttons, we will see about that in this article later on.
Pre-requisites to Make Instagram Signup Page In HTML and CSS
- Basic knowledge of HTML
- Basic knowledge of CSS/CSS3
Adding Phone Images
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Instagram Registration</title>
<link rel="stylesheet" href="style.css">
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://cdn.jsdelivr.net/npm/jquery@3.6.0/dist/jquery.slim.min.js"></script>
<!-- Popper JS -->
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-sm-6">
<img src="pngegg.png" class="phone1img" alt="">
<img src="pngwing.com.png" class="phone2img" alt="">
</div>
</div>
</div>
</body>
</html>
*{
margin: 0;
padding: 0;
}
.phone1img, .phone2img{
height: 650px;
padding-top: 70px;
float: right;
margin: 10px;
position: absolute;
}
.phone1img{
z-index: 1;
}
.phone2img{
left: -170px;
}
First of all, we will create images which should be in the left of the signup form. Before that, we have linked our style.css file and as we can see here we have a bunch of script files are there, these files are for bootstrap purpose, we can use classes of bootstrap to reduce our some CSS work, we are doing this project with HTML and CSS, so we won’t use it much.
Now we have added container div which have two images, you can use 1 image as well. Then we need to jump on the CSS part to set these images, Firstly, we have removed default margin and padding, then we are adjusting our images using width, float and padding, now here we have to use position to absolute, so both image come in same spot, and we have moved our second image to somewhat left, also we have applied z-index to 1 on first image, so it will come over the second image.
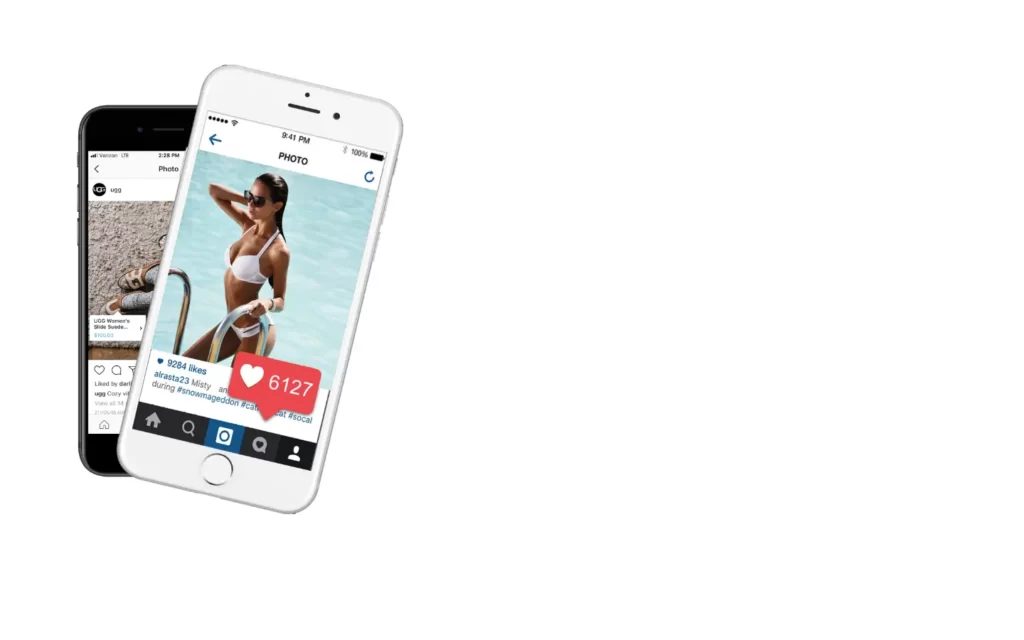
Adding Other Element For The Page
<div class="col-sm-6">
<div class="right-column text-center">
<img src="instalogo.png" class="insta-logo" alt="">
<p class="insta-info">Sign up to see photos and videos from your friends.</p>
<button type="submit" class="btn btn-primary btn-block"><img src="fb.png" class="fbimg"> Log in With Facebook</button>
<p class="or-text">OR</p>
</div>
</div>
body{
background-color: #fafafa !important;
}
.container{
margin-top: 20px;
}
.insta-logo{
width: 12rem;
}
.right-column{
background: #fff;
border: 1px solid #e6e6e6;
width: 350px;
margin: 10px;
padding: 40px;
}
.insta-info{
font-weight: 600px;
line-height: 20px;
font-size: 17px;
color: #999;
}
.btn .fbimg{
width: 16px;
}
.or-text{
font-size: 13px !important;
color: #999;
font-weight: 600;
}
.or-text::before{
content: '';
background: #efefef;
display: block;
height: 2px;
width: 110px;
position: relative;
top: 11px;
}
.or-text::after{
content: '';
background: #efefef;
display: block;
height: 2px;
width: 110px;
position: relative;
bottom: 10px;
left: 160px;
}
Now we have done with images, after that we will focus on instagram logo, sign in with Facebook button, and little information of Instagram. First of all we have added instagram logo and short information, we have just adjusted it according to actual instagram signup form. Then we have added a sign-in with Facebook button, this button is created using bootstrap class btn btn-primary btn-block
. Here btn-primary
indicates blue color, so we will get blue button with these classes.
Now for instagram info, we just give some basic style and color to adjust it, then we need a OR text to do partition our signup form and information, we have again done some basic styling but here we used :before
and :after
to give lines with the text in left and right side.
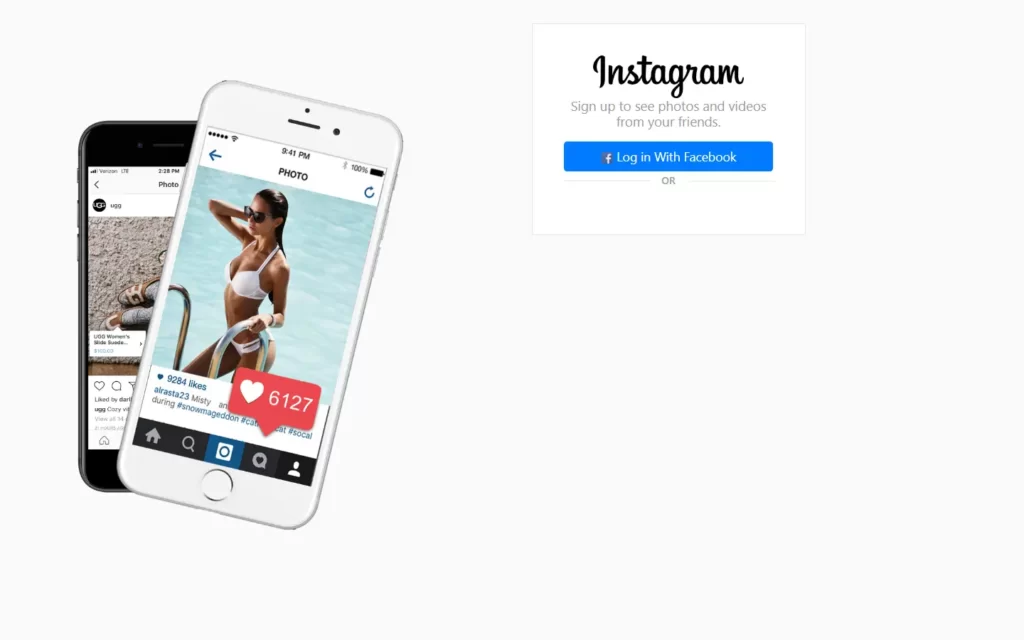
Adding Signup form
<form>
<div class="form-group">
<input type="text" class="form-control" name="" placeholder="Mobile Number Or Email">
</div>
<div class="form-group">
<input type="text" class="form-control" name="" placeholder="Full Name">
</div>
<div class="form-group">
<input type="text" class="form-control" name="" placeholder="Username">
</div>
<div class="form-group">
<input type="password" class="form-control" name="" placeholder="Password">
</div>
<button type="submit" class="btn btn-primary btn-block"> Sign up</button>
</form>
<p class="terms-text">By Signing up, you agree to our <b>Terms</b>,<b>Data Policy</b> and<b>Cookies policy</b>.</p>
</div>
<div class="login-text text-center">
<p>Have an account?<a href="https://www.youtube.com/watch?v=dQw4w9WgXcQ">Login</a></p>
</div>
</div>
.form-group{
margin-bottom: 6px !important;
}
.form-control{
background: #fafafa !important;
border: 1px solid #efefef;
font-size: 12px !important;
}
::placeholder{
color: #999 !important;
}
.btn{
margin: 12px auto;
padding: 2px !important;
font-weight: 600 !important;
}
.btn-primary{
background-color: #3897f0;
border: 1px solid #3897f0 !important;
}
.terms-text{
line-height: 18px;
font-size: 14px;
color: #999;
margin: 5px 20px;
}
.login-text{
background: #fff;
border: 1px solid #e6e6e6;
width: 350px;
margin: 20px;
}
.login-text a:hover{
text-decoration: none;
}
Now finally, we will add our signup form which have basically 4 input fields for email, name, username, and password also we have included a button to submit the form. For the styling purpose, we just have to give some color to placeholders and font-size nothing so much we have done in here.
After that, we have a little paragraph for term and conditions, which don’t have much styling again. Now lastly we have sign-in button if user already have an account.
I recommend you to run this whole project as it is and click on login button, If you like the output you can share to your friends as well.
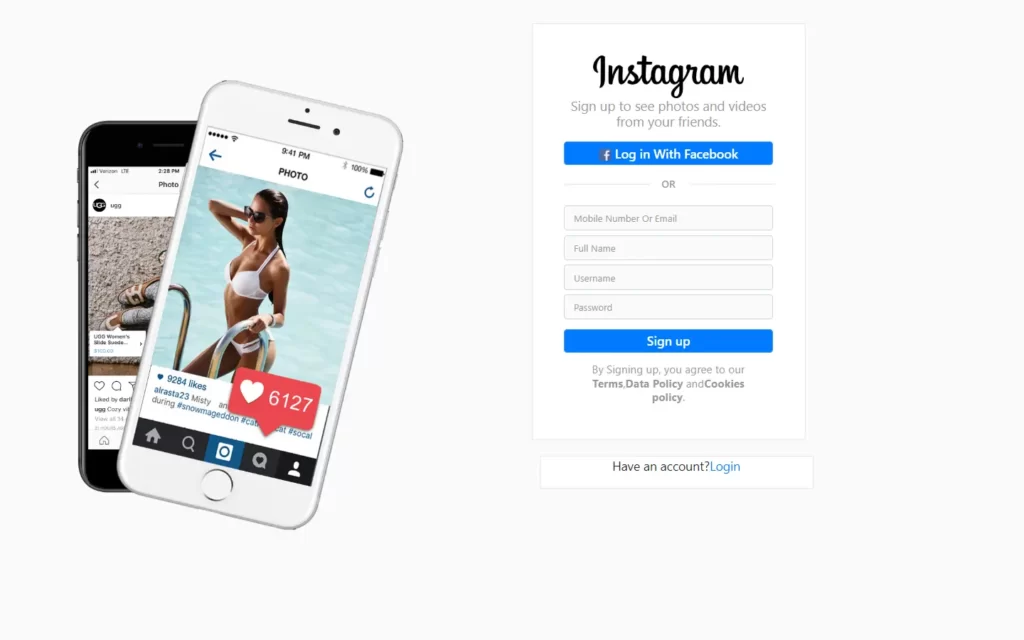
Full Source Code Of Create Instagram Signup Page Using HTML And CSS3
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Instagram Registration</title>
<link rel="stylesheet" href="style.css">
<script src="https://kit.fontawesome.com/a076d05399.js"></script>
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://cdn.jsdelivr.net/npm/jquery@3.6.0/dist/jquery.slim.min.js"></script>
<!-- Popper JS -->
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-sm-6">
<img src="pngegg.png" class="phone1img" alt="">
<img src="pngwing.com.png" class="phone2img" alt="">
</div>
<div class="col-sm-6">
<div class="right-column text-center">
<img src="instalogo.png" class="insta-logo" alt="">
<p class="insta-info">Sign up to see photos and videos from your friends.</p>
<button type="submit" class="btn btn-primary btn-block"><img src="fb.png" class="fbimg"> Log in With Facebook</button>
<p class="or-text">OR</p>
<form>
<div class="form-group">
<input type="text" class="form-control" name="" placeholder="Mobile Number Or Email">
</div>
<div class="form-group">
<input type="text" class="form-control" name="" placeholder="Full Name">
</div>
<div class="form-group">
<input type="text" class="form-control" name="" placeholder="Username">
</div>
<div class="form-group">
<input type="password" class="form-control" name="" placeholder="Password">
</div>
<button type="submit" class="btn btn-primary btn-block"> Sign up</button>
</form>
<p class="terms-text">By Signing up, you agree to our <b>Terms</b>,<b>Data Policy</b> and<b>Cookies policy</b>.</p>
</div>
<div class="login-text text-center">
<p>Have an account?<a href="https://www.youtube.com/watch?v=dQw4w9WgXcQ">Login</a></p>
</div>
</div>
</div>
</div>
</body>
</html>
style.css
*{
margin: 0;
padding: 0;
}
.phone1img, .phone2img{
height: 650px;
padding-top: 70px;
float: right;
margin: 10px;
position: absolute;
}
.phone1img{
z-index: 1;
}
.phone2img{
left: -170px;
}
body{
background-color: #fafafa !important;
}
.container{
margin-top: 20px;
}
.insta-logo{
width: 12rem;
}
.right-column{
background: #fff;
border: 1px solid #e6e6e6;
width: 350px;
margin: 10px;
padding: 40px;
}
.insta-info{
font-weight: 600px;
line-height: 20px;
font-size: 17px;
color: #999;
}
.btn .fbimg{
width: 16px;
}
.or-text{
font-size: 13px !important;
color: #999;
font-weight: 600;
}
.or-text::before{
content: '';
background: #efefef;
display: block;
height: 2px;
width: 110px;
position: relative;
top: 11px;
}
.or-text::after{
content: '';
background: #efefef;
display: block;
height: 2px;
width: 110px;
position: relative;
bottom: 10px;
left: 160px;
}
.form-group{
margin-bottom: 6px !important;
}
.form-control{
background: #fafafa !important;
border: 1px solid #efefef;
font-size: 12px !important;
}
::placeholder{
color: #999 !important;
}
.btn{
margin: 12px auto;
padding: 2px !important;
font-weight: 600 !important;
}
.btn-primary{
background-color: #3897f0;
border: 1px solid #3897f0 !important;
}
.terms-text{
line-height: 18px;
font-size: 14px;
color: #999;
margin: 5px 20px;
}
.login-text{
background: #fff;
border: 1px solid #e6e6e6;
width: 350px;
margin: 20px;
}
.login-text a:hover{
text-decoration: none;
}
Output
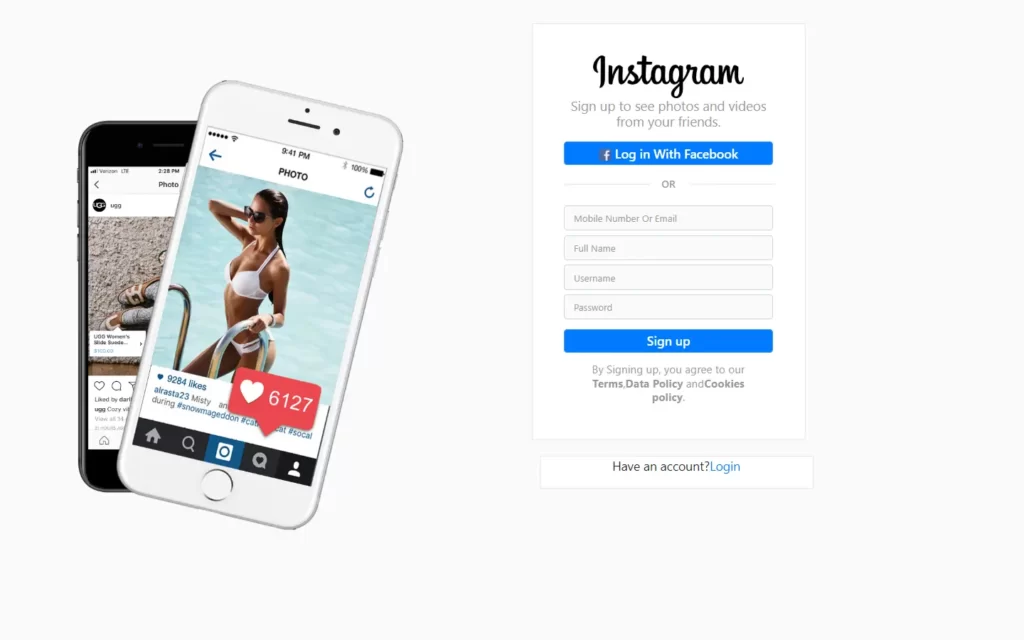