In this article, we have will learn to make Custom Warning Alert Notification using HTML, CSS & JavaScript. In this we will have a button and if we click it then we will get a pop-up message as a warning. We will also design this warning pop-up with the help of HTML and CSS. We will add JavaScript on the button. This will be a very basic and beginner-friendly, So let’s make it step by step.
Pre-requisites to Make Custom Warning Alert Notification using HTML CSS & JavaScript
- Basic knowledge of HTML.
- Basic knowledge of CSS.
- Good knowledge of JavaScript.
Creating HTML Markup and Setup Default Values
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Warning Alert Notification/title>
<link rel="stylesheet" href="style.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"/>
<script src="https://code.jquery.com/jquery-3.4.1.js"></script>
</head>
@import url('https://fonts.googleapis.com/css?family=Poppins:400,500,600,700&display=swap');
*{
margin: 0;
padding: 0;
user-select: none;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
html,body{
height: 100%;
}
body{
display: grid;
place-items: center;
overflow: hidden;
}
In the HTML Part, first of all, we will add our CSS file, then we have added a font-awesome library to get icons from this library. Then we have added a CDN link for to import the jQuery.
In CSS part, we have added Poppins fonts, which is easily available in Google. Then we have reset the default values in the CSS. And also we have provided some height to the body, also we have centered our body part using place-items
property.
Adding the Button And Message
<button>Show Alert</button>
<div class="alert hide">
<span class="fas fa-exclamation-circle"></span>
<span class="msg">Warning: This is a warning alert!</span>
<div class="close-btn">
<span class="fas fa-times"></span>
</div>
</div>
button{
padding: 8px 16px;
font-size: 25px;
font-weight: 500;
border-radius: 4px;
border: none;
outline: none;
background: #e69100;
color: white;
letter-spacing: 1px;
cursor: pointer;
}
.alert{
background: #ffdb9b;
padding: 20px 40px;
min-width: 420px;
position: absolute;
right: 0;
top: 10px;
border-radius: 4px;
border-left: 8px solid #ffa502;
overflow: hidden;
opacity: 0;
pointer-events: none;
}
.alert.showAlert{
opacity: 1;
pointer-events: auto;
}
.alert .fa-exclamation-circle{
position: absolute;
left: 20px;
top: 50%;
transform: translateY(-50%);
color: #ce8500;
font-size: 30px;
}
.alert .msg{
padding: 0 20px;
font-size: 18px;
color: #ce8500;
}
.alert .close-btn{
position: absolute;
right: 0px;
top: 50%;
transform: translateY(-50%);
background: #ffd080;
padding: 20px 18px;
cursor: pointer;
}
.alert .close-btn:hover{
background: #ffc766;
}
.alert .close-btn .fas{
color: #ce8500;
font-size: 22px;
line-height: 40px;
}
Now we need to add our button, for that we have added a button named show alert. Then we have added a div
in which we will add our message, in this we have added warning icon using fas fa-exclamation-circle
, then we have added our message as our span. Then after, we have added cancel button icon with the help of font awesome using fas fa-times
.
In CSS part, we have applied some basic CSS on the button, in this button we have added some font size
and font weight
with some basic padding and height. Now for the message we have added some color, width, and some border as well. We made overflow to hidden, and added position to absolute so that warning box will be set at the position.
Then after we have added opacity to 0, so that warning box will be hidden by default. Then we have added another class named show alert, in this we have just added opacity to 1, but we will add this class using JS. Then we have applied some styles to the button and text as well.
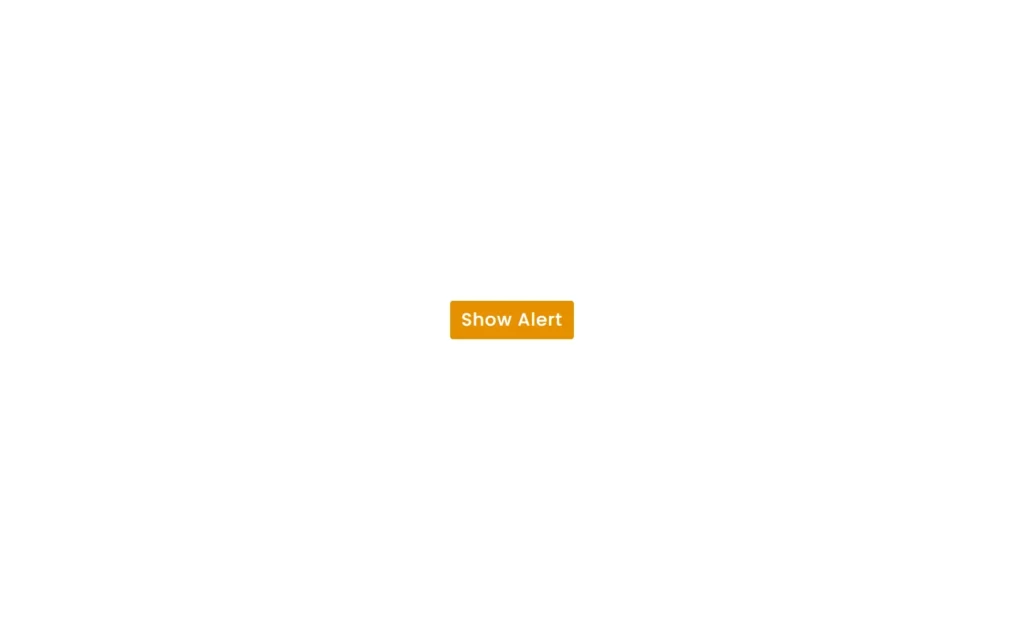
Adding Functionality to Button and Animations
<script>
$('button').click(function(){
$('.alert').addClass("show");
$('.alert').removeClass("hide");
$('.alert').addClass("showAlert");
setTimeout(function(){
$('.alert').removeClass("show");
$('.alert').addClass("hide");
},5000);
});
$('.close-btn').click(function(){
$('.alert').removeClass("show");
$('.alert').addClass("hide");
});
</script>
.alert.show{
animation: show_slide 1s ease forwards;
}
@keyframes show_slide {
0%{
transform: translateX(100%);
}
40%{
transform: translateX(-10%);
}
80%{
transform: translateX(0%);
}
100%{
transform: translateX(-10px);
}
}
.alert.hide{
animation: hide_slide 1s ease forwards;
}
@keyframes hide_slide {
0%{
transform: translateX(-10px);
}
40%{
transform: translateX(0%);
}
80%{
transform: translateX(-10%);
}
100%{
transform: translateX(100%);
}
}
In JavaScript Part, we have used jQuery so that it will be much easier. In here, we have called the button and added the function on click
event using $('button').click(function())
this line of code. In this function, we have added the show
class and showAlert
class and removed hide
class. Then after we have added some time out to this message box by 5000ms which 5 seconds. And we have called the close icon, on which we have added hide
class and removed show
class.
In CSS part, we need to add some animation on show and hide classes, in these we have added 1 second duration and ease type. In these animations, we have added 4 phases of the duration. At 0% we have translated to 100%, at 40% we have gave X to -10%, at 80% we have gave X to 0. And lastly at the 100% we have gave X to -100%, similarly in hide animation we will do the same but in reverse order. By doing this we will get some cool effect like fade-in type of effect.
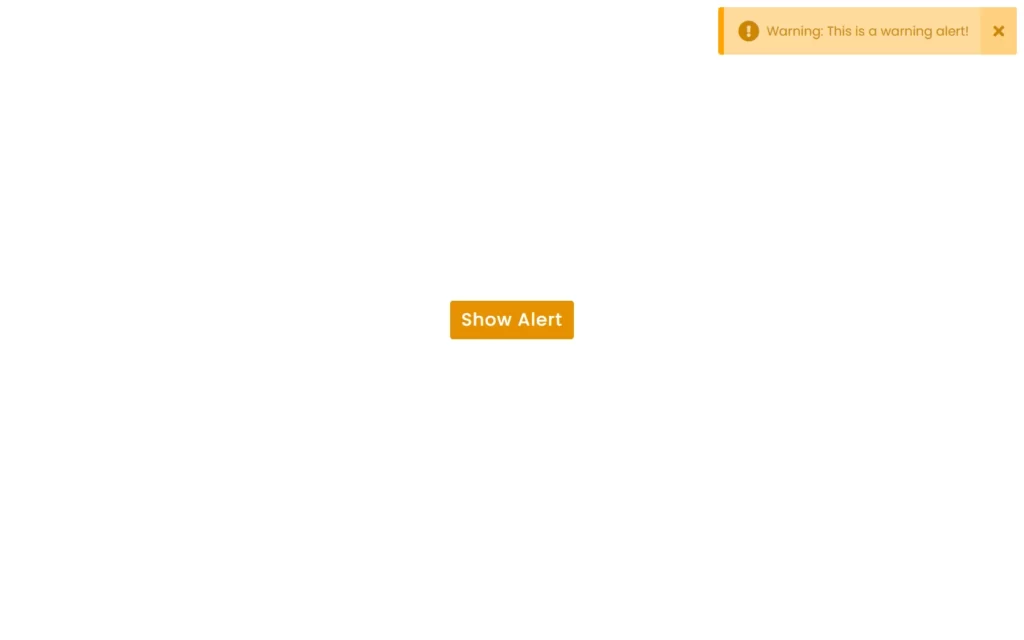
Full Source Code of Custom Warning Alert Notification using HTML, CSS & JavaScript
index.html
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Warning Alert Notification | CodingNepal</title>
<link rel="stylesheet" href="style.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"/>
<script src="https://code.jquery.com/jquery-3.4.1.js"></script>
</head>
<body>
<button>Show Alert</button>
<div class="alert hide">
<span class="fas fa-exclamation-circle"></span>
<span class="msg">Warning: This is a warning alert!</span>
<div class="close-btn">
<span class="fas fa-times"></span>
</div>
</div>
<script>
$('button').click(function(){
$('.alert').addClass("show");
$('.alert').removeClass("hide");
$('.alert').addClass("showAlert");
setTimeout(function(){
$('.alert').removeClass("show");
$('.alert').addClass("hide");
},5000);
});
$('.close-btn').click(function(){
$('.alert').removeClass("show");
$('.alert').addClass("hide");
});
</script>
</body>
</html>
style.css
@import url('https://fonts.googleapis.com/css?family=Poppins:400,500,600,700&display=swap');
*{
margin: 0;
padding: 0;
user-select: none;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
html,body{
height: 100%;
}
body{
display: grid;
place-items: center;
overflow: hidden;
}
button{
padding: 8px 16px;
font-size: 25px;
font-weight: 500;
border-radius: 4px;
border: none;
outline: none;
background: #e69100;
color: white;
letter-spacing: 1px;
cursor: pointer;
}
.alert{
background: #ffdb9b;
padding: 20px 40px;
min-width: 420px;
position: absolute;
right: 0;
top: 10px;
border-radius: 4px;
border-left: 8px solid #ffa502;
overflow: hidden;
opacity: 0;
pointer-events: none;
}
.alert.showAlert{
opacity: 1;
pointer-events: auto;
}
.alert.show{
animation: show_slide 1s ease forwards;
}
@keyframes show_slide {
0%{
transform: translateX(100%);
}
40%{
transform: translateX(-10%);
}
80%{
transform: translateX(0%);
}
100%{
transform: translateX(-10px);
}
}
.alert.hide{
animation: hide_slide 1s ease forwards;
}
@keyframes hide_slide {
0%{
transform: translateX(-10px);
}
40%{
transform: translateX(0%);
}
80%{
transform: translateX(-10%);
}
100%{
transform: translateX(100%);
}
}
.alert .fa-exclamation-circle{
position: absolute;
left: 20px;
top: 50%;
transform: translateY(-50%);
color: #ce8500;
font-size: 30px;
}
.alert .msg{
padding: 0 20px;
font-size: 18px;
color: #ce8500;
}
.alert .close-btn{
position: absolute;
right: 0px;
top: 50%;
transform: translateY(-50%);
background: #ffd080;
padding: 20px 18px;
cursor: pointer;
}
.alert .close-btn:hover{
background: #ffc766;
}
.alert .close-btn .fas{
color: #ce8500;
font-size: 22px;
line-height: 40px;
}
Output
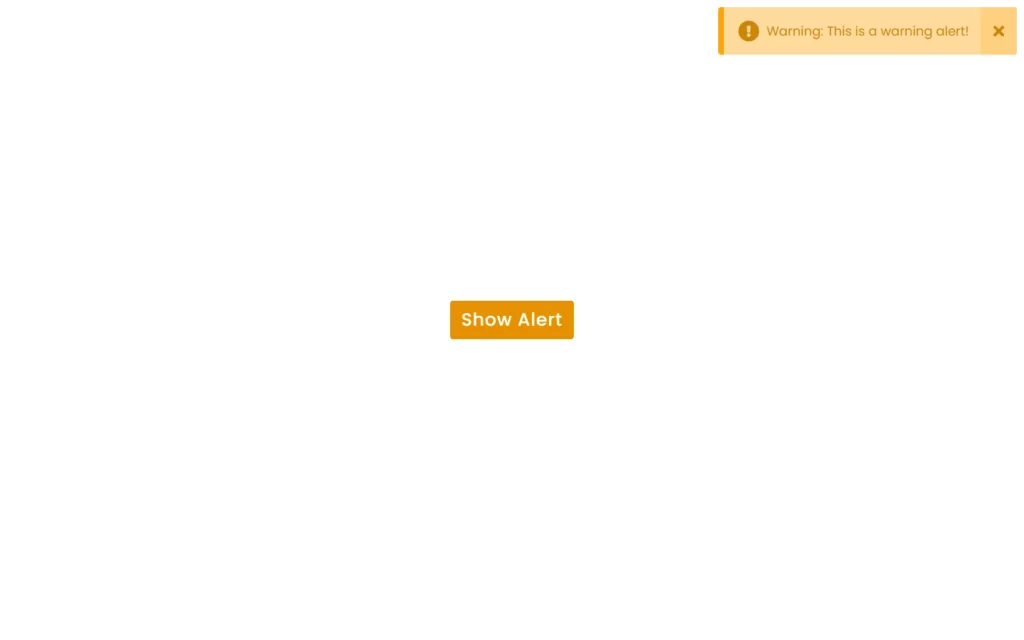
Check out video reference here: