we’re going to create a to-do list using javascript. the to-do list will have a nice UI. The user can add what to do by filling in the input and press ENTER, after which he can check what-to-do when finished, or delete it using the delete button.
The to-do list is stored in the local storage, so when he updates the page, he can always find it there on the list. There are opportunities for the user to clear the list, with the click of a clear button, in the top right corner of our app.
you see To-Do List using JavaScript demo here
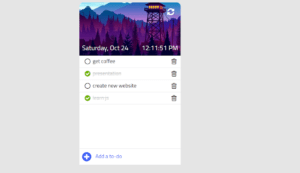
HTML
so let’s start with the HTML part. In the HTML part, we have here a container inside. There are the header and content inside. The content we have a list and then the add item div or the input inside the header. We have the Clear button to clear the local storage and also.
We have the date which will show today’s date then inside our list we have our item. Our item here has three Componate’s first is the button check. Then the text and then the trash button for our add item here. We have a plus icon then input with the text type and the ID input. And the placeholder says to add a to-do now this is the rule HTML file.
We still need to link our CSS files and the JavaScript adopt inside our head tag. We’re going to link the font from Google and the font awesome for the icons. And our own style that CSS file and at the bottom before the closing body tag. We’re going to link our script let’s now move to JavaScript but the first thing.
javascript-select element
I’m going to do is to select our elements. We’re going to need to select the clear button using a query selector. The query selector here selects the first item with this class name for us our document has only one item. With the class both clear and then we need to select the date element using the get element by ID. Which takes in the ID of the element then we need to select our last. And also the input so we can get the value the user enter and this field.
insertAdjacentHTML
Let’s talk about insert adjacent HTML as you saw before we need to add new items to our list. And keeping the old ones so we’ll need for that method called answered adjacent HTML. So what this method does let’s say this is our page. And then we have here an item with the ID element and inside.
We have some content, for example, the first child and then the last child, and inside we have other elements. This is the beginning of your element or the start this is the end of our element right now. We need first select our element using for example get element by ID. The syntax of the insert is just an HTML.
You need to say element.insert adjustment our method which takes in two arguments. The first one is the position of the thing we are adding to our element. So the position might before begin before they began or after began before and/or after the end. And the second parameter or argument is a text which could be something for example.
If we say before began the text or something will place right before. Our elements and if we say after begin. It will be right after but the beginning of our element. Which means before the first child. if we say before the end.
It will place right before the end which means after the last child in our inside or element. And if we say after the end our son will be placed outside the element. And after the end, so you may think why we just don’t use inner HTML. If we use an HTML this will overwrite all or everything inside our element.
javascript- Add a to-do-list
Now let’s talk about how to add a to-do there our list. You still remember that was select our list element using this line of code. Now we’re going to say less than answered an HTML. Which takes in the position and the text. Here is our item or to do. So we’re going to create a constantly called text equal to our item HTML code.
Here this is they’re here this is the button for the complete added an attribute called job equals complete. Then the text itself says drink coffee and last the trash button.
I added an attribute of job equals to delete and then I will put this inside a back deck or grave accent. I’m using here a template lateral. Now we need constant which is the position and I will use it here before and so every new item will be placed at the end or just after the last child. Then I will put all this code inside the function called a to-do.
Enter keycode
We are going to use or to reuse this code again and again your function will take a parameter called to-do. Which I’ll place here using a placeholder so here is our dollar sign and they call a bracket. And then our parameter knows if I say a to do and pass during coffee string to it. I will add a new item to the list successfully. Now in the user type in a to-do, he must also press ENTER to add it to the list. So how do we know that the user press the key and the keyboard?
So we will need to add an event listener to our document. Which he’s in key up so whenever the user press a key this function here will fire up this function will take an event now every key. And the keyboard has a keycode for example. The Enter key has a keycode which is 13. Now I will just say The Event that key code is 13. Then we know for sure that the user press the Enter key now. If it’s true we will get the value of the input.
So we’ll create a constant called to-do equals input.value. I remember was select our input field using this line of code. So now we need to check if the input isn’t empty if the user really felt the input or not. So we’ll say to do now if the string to do here is empty. It will return false which means it will want to run the code inside the IF statement.
Then inside our IF statement, we will call the function are to do so say had to-do. And we pass an hour to do variable or strength. The last thing we need to do is to make the end input empty. So we’ll need to say and input that value equals empty string. The problem here is that this code would only show this to the user interface.
javascript-store to-do-list
We also need to store a to-do. So a to do we will need to create a variable called List which is an array that has inside objects. For example, our first item will have ID 0. This is its name during coffee it’s ID 0 done set to false and set to false. This is another item and this is what we’ll show to the user interface. I created a new variable here which is the ID and I set it to 0. Why because every time we add a new item to the list.
We need to increment ID by one done. You can see the done here is set to false and here done set. The item here or the to-do is completed. We set this to true and if it’s not if it’s false means this one here is not completed.
The same thing for the trash property. Where a set of false which means that the item here is not remove. It’s still there now let’s update our latest code it’s looking like this. So far now or function are to do here is taken only one variable.
Which is to do now to add all the errors are all the other property. The ID and then done and trash we set them to false by default. Because when the user had a new to-do to the list by are automatic. The done and trash are set to false. Because the to-do is not complete yet and it’s not in the trash yet now.
We need to add this to our list variable and we will use for that the push method will push to our list a new object with these properties. The name is our to-do here the ID is defined here. If it’s the first time the user is adding an item to the last the ID here will be set to zero. Then done is set to false and trash is also set to false. Remember after adding an item. We need to increment the ID by one.
javascript-update to-do-list
Let’s update our function to-do or add to-do this our function is looking like. This so far now there’s other parameters. That we need to take in mind first you could see here the icon and this one is different. They are different because they have different class names this is the class name for this one and this is the class name for this one also.
This text here has not aligned through but this one does. So this text here has another class name. Which is lying through this class name. We need to create some constant for these class names so we can locate them easily. So I’m gonna create a constant call to check. Which will take in this class name one for unchecking another one for a line through. Now our function here our to-do will get as a parameter an ID.
This idea would go here so the first button here has the check button. Which has a job complete. We’ll have this ID here if it’s zero. This one will have the ID zero and also the delete button will have the same ID. The next parameter or argument is done. When done is set to false. we need to show the icon. Which means we’ll use this class name means this variable constant.
If it is set to true we’ll use this class name. if statement check if a done, is set to true or false. And then take the decision whether to use the check or the uncheck class name.
So I’m gonna say or I’m gonna create a new constant call done equal to true then. We’ll use a check class name otherwise. We’ll use the uncheck class name also I will use or I will create a new constant called line. If done is true which means it is check.
We need to add a line through the text so I will add a line through. If it’s false I will use an empty string then I will add this done and line constantly the done constantly will be here and the line will be here for the text now the last argument is trash.
So if the item is in the trash which means. It is set to true we don’t need to run this code. We will need to show anything to the user because the item is remove. So I will run returns after return inside a function no matter what code is after that return. It won’t be run if trash is set to false there is no return.
javascript-to-do-list done
now let’s talk about what will happen. We need to do is done before a todo is done our button here has this class name. If the user clicks on this button we need to remove this class name. And then add the class name for this icon also do is the possibility for the user to undone.
But to do so if the user clicks on this button which has this class name. We need to remove this class name. And then add the class name for this icon. So how do we do that using code? When the user for example clicks on this element we need to set or to select that element. And then use the class list which will return the class names of this element.
Now we need to decide if the class exists we need to remove that class otherwise. If the class does not exist in the class list we need to add that class to the class list to-do. We need to use the toggle method toggle will check if the class exists it will remove.
If it’s not it will add it to the class list now. We will create a function called complete to-do. This complete to do function will be run whenever the user clicks on these buttons. This function here would take us a parameter. The element then we’ll say element that class list that toggle check so if the check isn’t a class. We’ll remove it otherwise. We will add it to the class list so we need to remove them and check.
If it’s in the class otherwise added to the class lists. IF the check is in the class list we will remove it and add the and check. Otherwise, if a check is in the class list. We’ll remove it and add the check class to the class list. Now we need to add a line through this text. I will force the need to select this text on this element. Because this element here the one was select.
Where button to select this text who need first to go to the parent element. and then select the text element using a query selector. and then used the class less and then toggle the line through.
Now we need to update our last variable. Because three lines of code will just update the user’s interface. We also need to update our list variable before updating our list variable we must first know which item.
javascript-remove to-do-list
Let’s now talk about how to remove a to-do. For example or to-do when the user clicks on this button we should remove all this block if the user clicks on this. Once we need to select this element now the element we want to remove is not this element here we need to remove this whole element.
Which is the element that parent node and to remove this element here we need to get to its parent element. Which means we’ll say element that parent node. Then we’ll use the remove child method and a person the element.
That parent node we put this inside the function called remove to-do. Which takes in the element as a parameter remember this will remove the element just from the user interface. We also need to update our last variable. So I’m gonna say the element ID then trash this is the property equals true. So we say the trash the true which means the element ordered to-do as removed.
Target an element created dynamically
Now our elements here or our items are created dynamically. So we can’t add an event listener to these buttons. For example, the solution here is we need to select the parent element. Which is the list so we only select this list element. Then we need here to add an event listener to that element. Which will be the click event and after a click event.
We’ll run a function that will take an event as a parameter. And then we will say event dot target this will return the element.
We collect every element inside our list element. So for example, if We click the button trash button on this delete button it will turn this line of code. This trash button here has an ID which is zero the job attribute is delayed. We will set this to a variable called element soI can use this for later now when. We click on the button or do you both have the same ID.
So we need to check our need to know what is their job attribute a value so I will say the event that targets those attributes. That job that values this will turn either delayed or complete.
we will say this a constantly called element job now. I will just use an IF statement so I will say element job is complete or as delete. If it’s complete we will run the function complete to-do. Which will take in the element variable and if it’s deleted. We, Will, run the function remove to-do. Which will take in the element variable 2.
javascript-save to-do-list in local storage
Now we need to save our to-do list to the local storage, in general, to save a value to local storage. We will say local storage that said item then we give the key and value.
Here We want to store now to get the item I will say local storage that get item of the key. We will give the key the same to-do and then our last is a variable.
So I’m gonna use JSON that stringify method now the question is were to put this line of code the answer is inside our code whenever we update our must array just after it.
javascript-restore to-do-list in local storage
Now we need to restore the to-do list to restore. We will use to get item and our key which is to do with said this to a variable called data. We need to check after his data what do we mean when our user opens our to do list application for the first time. It is nothing in local storage which means the data is empty.
If data is empty we need to create a list array and set theID to 0 remember. We need to define our list and ID variables outside the blocks now. What does is data means this is not the first time our user opens the application. So we will need to get the list.
So we will say last equals JSON. That parse the data we’ll get from our local storage. And then we will need to load the last to the user interface for that. we’ll use the function would to-do and then we need to set the ID equals to list. That length why because we need to set ID. The last ID and our list variable or array.
Let’stalk about our function the load. The function will take as a parameter an array which is our list here. Then we need to loop over our array. We will use for each which will run a function that takes an item from the list or from the array list . Now We will need to show this item to the user. So I’m gonna use the RTD function. That takes in the name of the item it’s done property and the trash property.
clear storage
Now the user might want to clear the storage. So when we click on the button. Which was selected using this line of code. We will clear the local storage and refresh the page. So I will add an event listener to our Clear button. Which will fire up a function. This function will clear the storage is AS I declare the method and reload the page.
show date
Now we need to show the today’s date for that we already selected our elements. We call these constant elements. So I’m gonna date element dot innerHTML equals today. today is a variable. which is equal to a new date object.
But to show this date in format. We should use to local State string method. Which will take in two arguments the language and some options. The options here are a is an object which has some properties the weekday.
showtime
Now we need to show the current time. For that, we already selected the our elements. Here we create function showtime.inside the function we get the current time. And show the time in this format. We use the toLocaleTimeString method. And we use the set timeout method for updating the current time continuously.code is below.
//show time
function showtime(){
const currenttime=new Date();
timeElement.innerHTML=currenttime.toLocaleTimeString()
setTimeout(showtime,1000);
}
showtime()
Full source code of To-Do List using JavaScript:
https://github.com/patelrohan750/To-Do-List-in-javascript
watch the tutorial To-Do List using JavaScript