Hello, guys in this project we’re gonna build a Speed Typing Game using JavaScript application called word beater. And we’re gonna build it in pure JavaScript. No use of libraries or frameworks or anything like that and it’s pretty simple.
We just get random words and you get a certain amount of seconds to type. We have it set to 5 seconds. But this is you can change this really easily and once you type it in. If you make it in that amount of time. You’ll get a point to add to your score. And you just keep going until you fumble up and you mess up and you don’t do it in that amount of time.
And then the game’s over and then if you want to play again. You just simply type in the word that’s shown.
so you can see a demo –> Speed Typing Game using JavaScript.
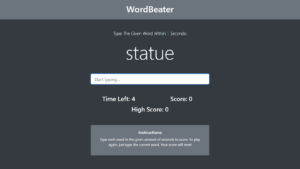
Speed Typing Game using the JavaScript project is pretty simple when you come to the page the game automatically starts. It starts the timer and it’s autofocus so it puts you right in there and then of course. If you want to play again you just put in the word and it’ll start again. So pretty simple but I think it’s a fun little project.
Skeleton of speed typing game using javascript
let’s go ahead and get started alright guys so I’m not going to type out the HTML. I’m just gonna go over real quick it’s a very simple bootstrap layout. We have an index.html with the mark-up and then a main.js file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>WordBeater</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity="sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO"
crossorigin="anonymous">
</head>
<body class="bg-dark text-white ">
<header class="bg-secondary text-center p-3 mb-5">
<h1>WordBeater</h1>
</header>
<div class="container text-center">
<!-- Word & Input -->
<div class="row">
<div class="col-md-6 mx-auto">
<p class="lead">Type The Given Word Within
<span class="text-success" id="seconds">5</span> Seconds:</p>
<h2 class="display-2 mb-5" id="current-word">hello</h2>
<input type="text" class="form-control form-control-lg" placeholder="Start typing..." id="word-input" autofocus>
<h4 class="mt-3" id="message"></h4>
<!-- Time & Score Columns -->
<div class="row mt-5">
<div class="col-md-6">
<h3>Time Left:
<span id="time">0</span>
</h3>
</div>
<div class="col-md-6">
<h3>Score:
<span id="score">0</span>
</h3>
</div>
<br><br>
<div class="col">
<h3>High Score:
<span id="highscore">0</span>
</h3>
</div>
</div>
<!-- Instructions -->
<div class="row mt-5">
<div class="col-md-12">
<div class="card card-body bg-secondary text-white">
<h5>Instructions</h5>
<p>Type each word in the given amount of seconds to score. To play again, just type the current word. Your score
will reset</p>
</div>
</div>
</div>
</div>
</div>
</div>
<script src="main.js"></script>
</body>
</html>
Main.js
So let’s jump over to our JavaScript and get started. The first thing I’m going to do is just create some global variables. So we’re gonna need a time variable. I’m gonna say let’s time and I’m using let. Because this is going to actually change.
We’re going to just set it to live for now and obviously. It’ll go you know five four three two one zero. So we want to make sure we use let because of Constis for variables. That you’re not going to reassign so let’s do that let’s also create a score variable. And the score is obviously going to be set to zero at first.
Then we also want a variable called is playing and I’m not going to set it to anything. I’m just going to initialize it and basically, this just represents if the game is going on or not so once the game is over this should be false. And as it’s going on it should be true. These are global variables that we’ll be using within functions.
// Globals
let time = currentLevel;
let score = 0;
let isPlaying;
Next thing I’m gonna do is put in your Dom element variables. Because we want to pluck stuff out from the Dom. So I’m actually gonna paste that in because it’s pretty much the same thing over and over just for different elements. So we have the word input which is the text.
We have the current word which is the word that shows here the score display and the time display which are these two numbers message. That’ll show if it’s corrector game over and then seconds is this right here this number. Because we want this to reflect. So we need to we need to grabon to those elements.
// DOM Elements
const wordInput = document.querySelector('#word-input');
const currentWord = document.querySelector('#current-word');
const scoreDisplay = document.querySelector('#score');
const timeDisplay = document.querySelector('#time');
const message = document.querySelector('#message');
const seconds = document.querySelector('#seconds');
const highscoreDisplay = document.querySelector('#highscore');
The next thing I’m Going to do is put the words in an array. We’re just using a hard-coded array of words. There’s I don’t know I think there’s like 30 or something like that. So it’s just a variable called words and set to an array and you can change the words if you want.
const words = [
'hat',
'river',
'lucky',
'statue',
'generate',
'stubborn',
'cocktail',
'runaway',
'joke',
'developer',
'establishment',
'hero',
'javascript',
'nutrition',
'revolver',
'echo',
'siblings',
'investigate',
'horrendous',
'symptom',
'laughter',
'magic',
'master',
'space',
'definition'
];
The next thing we want to do is have an init function. So basically when the window loads. I want an init function to fire off. So I’m gonna go down here and let’s say initialize game and will say function init. I said when the window loads so I’m gonna go up to the top you could do window load. I’m gonna do window dot addeventlistener and we’re gonna listen for the load event and as soon as that happens we want to fire off in it okay.
window.addEventListener('load', init);
// Initialize Game
function init() {
console.log("init call")
}
show words
Now there are a few things that are going to happen inside init. The first thing that I want to call a function to load a word from the array. Basically a random word so let’s put a comment and let’s say load word from an array. And we’re gonna call that shows word okay and then that’s gonna take in the whole array of words.
So we need to create that function let’s just say pick and show the random word and we’ll say function show. It’s going to take in words. So which will be an array and the idea that we here are that. We want to create a random index because we can access the words in the array by index.
So 0 would be the first one that would be the second third and so on. Because arrays are zero-based so let’s create a variable called R and index to create a random index. And we’re gonna use math dot floor to round down and then math dot random to generate a random number. And then we want to multiply this by however many words there are in the array.
So we can take the words array and we can get the number of words with length. The length property will give us that so that’ll give us basically a random number.
So we want to use that as an index and then we want to output the word. Now actually let me put a comment here I’ll say generate an array index. I’m gonna have a lot of comments just so people know exactly what’s going on. Then we want to output a random word so we can do that by taking the current word variable to remember that was defined up.
So it’s defined to the ID of the current word which is this h2. So we want to take that and then we want to do dot inner HTML because that will allow us to fill stuff into that element. And we want to put in word which is the array and then a random index.
function init() {
// Load word from array
showWord(words);
// Call countdown every second
setInterval(countdown, 1000);
// Check game status
setInterval(checkStatus, 50);
}
// Check game status
function checkStatus() {
if (!isPlaying && time === 0) {
message.innerHTML = 'Game Over!!!';
score = -1;
}
}
Let’s see what do we want to do next. Let’s start to work on when we actually type something in the input. So in the init I’m gonna go under show words are show word and let’s say start watching on input on word input. So every time we type whenever we type something in here we want to fire off an event.
Which will fire off a function so let’s take the word input and let’s say add an event listener. We want to listen for any input and then when that happens we want to run a function. And we’ll call it to start the match. So we’ll go right under her start match so function start match now we’re gonna have another function that is dedicated to matching to whatever the user types.
I’m gonna call that will call that match words and that’s going to either return true or false. So right under this will save it’s gonna match the current word to the word input. So let’s say function match words it’s not gonna take anything because we’re getting this stuff from these Dom elements.
We have word input and current word so let’s see alright so let’s do and if and we want to match the word input. Now, word input is a text area, not a text area but a text input so just words input will give us the element itself. We want what’s typed in here we want value so in order to get that we need to do dot value.
And we want to basically match that against the current word but the current word again is going to be the element. Which is an h2 with class and all that we just want this the word inside. So we want the inner HTML okay so we want to match those know if those match then let’s take the message and let’s do enter HTML equals and let’s say correctly. Because it’s correct and then we also want to return true else then.
We’ll say message dot inner HTML equals nothing and we’ll say return false okay so save that and now let’s go back to the match words function. So we want to take if-else that I just did and we want this in the match words. And then will give us either true or false based on if those match.
So what we want to happen now if this match is we want to set the variable is playing to true. It’s the first thing we want to do we want to set the time and we’re actually going to set the time to six. Because of the page load which takes a second. So we want to set.
We just want to set this to one above whatever the initial time is and later on, We’re going to change this up a little. Because we’re gonna make the time a little more dynamic but for now, we’re just gonna set this to six. And then we want to show a new word obviously to type.
So it’s a show word and we’ll pass in words and then we also want to clear the input. Because as you can see that the text is still in there so we want to take the word input and we want to say dot value and set it equal to nothing.
The last thing we want to do is increment the score so we’ll say score plus. Which will increment it by 1 now that’s all well and good the score will be incremented but it’s not going to reflect in the Dom. We have to do that so let’s go outside of this if and let’s say score display. Which is what we set that Dom element to and then dot in our HTML and set it to whatever the score is alright.
So we want to check the status and if the game is over we want to reset the score. Now you would think we’d set it to zero. So if we lose as soon as I start to type the score should reset.
// Initialize Game
function init() {
// Show number of seconds in UI
seconds.innerHTML = currentLevel;
// Load word from array
showWord(words);
// Start matching on word input
wordInput.addEventListener('input', startMatch);
// Call countdown every second
setInterval(countdown, 1000);
// Check game status
setInterval(checkStatus, 50);
}
// Start match
function startMatch() {
if (matchWords()) {
isPlaying = true;
time = currentLevel + 1;
showWord(words);
wordInput.value = '';
score++;
}
// Match currentWord to wordInput
function matchWords() {
if (wordInput.value === currentWord.innerHTML) {
message.innerHTML = 'Correct!!!';
return true;
} else {
message.innerHTML = '';
return false;
}
}
You can also add new features to this project(Speed Typing Game using JavaScript).
full source code Speed Typing Game using JavaScript:
https://github.com/patelrohan750/Build-a-speed-typing-game-using-javascript
watch the Build a Speed Typing Game using JavaScript