In this article, we will make popup login form using HTML, CSS & JavaScript. Here we will have a button for login, and if we click on this button then we need a form should pop up in the screen with some animations. It will be a beginner-friendly project and will be useful in many ways, so let’s make it step by step.
Pre-requisites to Make Popup Login Form using HTML, CSS & JavaScript
Creating The HTML Markup
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Popup Login Form</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="center">
<button id="show-login">Login</button>
</div>
</body>
</html>
*{
margin: 0;
padding: 0;
box-sizing: border-box;
}
body{
background: linear-gradient(to right, #3a7bd5, #3a6073);
font-family: "Raleway", sans-serif;
height: 100vh;
}
.center{
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.center button{
padding: 10px 20px;
font-size: 15px;
font-weight: 600;
color: #222;
background: #f5f5f5;
border: none;
outline: none;
cursor: pointer;
border-radius: 5px;
}
In HTML, firstly we need to link with our CSS file, so designs done in CSS reflect in the HTML. Then we need to add our button, for that, we have added a div
with class name, so that we can apply some style using that. Then we have added a button with an ID.
Now in CSS, we have firstly removed default values. Then we have added some background to our body using liner-gradient
. Also, we have added font-family of Raleway
and we added some height to the body.
We need to add the area in the center of the body, for that we have set absolute position and used top
and left
property to adjust its position. We used transform: translate(-50%, -50%);
property to center the button. And lastly, we have customized the button using some basic CSS styling. We have added some color and styled the font, also we removed the borders and outline.
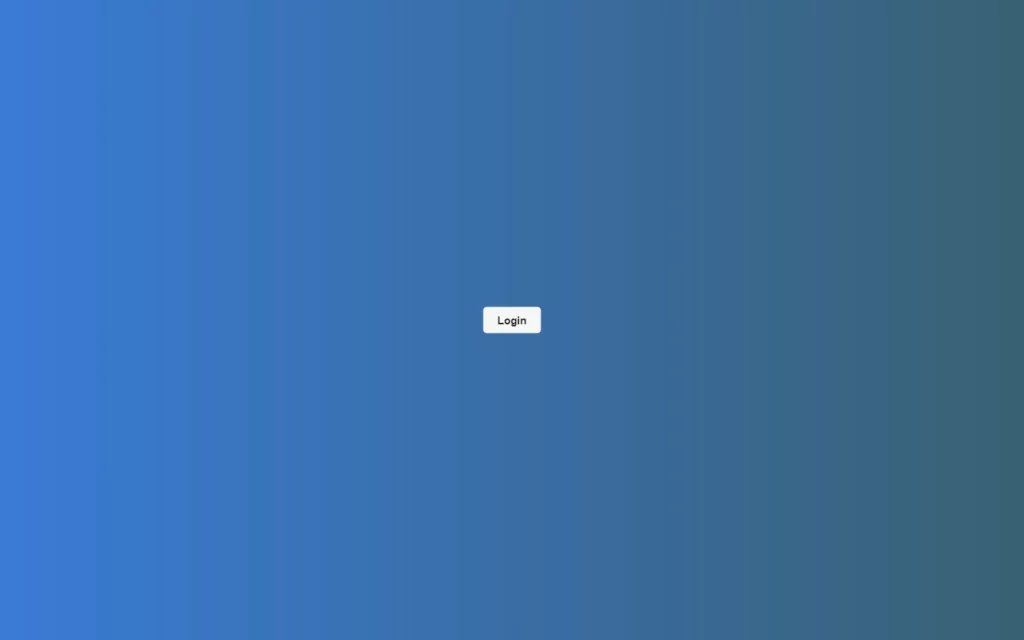
Adding Form and Customization
<div class="popup">
<div class="close-btn">×</div>
<div class="form">
<h2>Login</h2>
<div class="form-element">
<label for="email">Email</label>
<input type="text" id="email" placeholder="Enter Email">
</div>
<div class="form-element">
<label for="password">Password</label>
<input type="text" id="password" placeholder="Enter Password">
</div>
<div class="form-element">
<input type="checkbox" id="remember-me">
<label for="remember-me">Remember me</label>
</div>
<div class="form-element">
<button>Sign in</button>
</div>
<div class="form-element">
<a href="#">Forgot password</a>
</div>
</div>
</div>
.popup{
position: absolute;
top: -150%;
left: 50%;
opacity: 0;
transform: translate(-50%, -50%) scale(1.25);
width: 386px;
padding: 20px 30px;
background: #fff;
box-shadow: 2px 2px 5px 5px rgba(0,0,0,0.15);
border-radius: 10px;
transition: top 0ms ease-in-out 200ms,
opacity 200ms ease-in-out 0ms,
transform 200ms ease-in-out 0ms;
}
.popup.active{
top: 50%;
opacity: 1;
transform: translate(-50%, -50%) scale(1);
transition: top 0ms ease-in-out 0ms,
opacity 200ms ease-in-out 0ms,
transform 20ms ease-in-out 0ms;
}
.popup .close-btn{
position: absolute;
top: 10px;
right: 10px;
width: 15px;
height: 15px;
background: #000;
color: #eee;
text-align: center;
line-height: 15px;
border-radius: 15px;
cursor: pointer;
}
.popup .form h2{
text-align: center;
color: #222;
margin: 10px 0px 20px;
font-size: 25px;
}
.popup .form .form-element{
margin: 15px 0px;
}
.popup .form .form-element label{
font-size: 14px;
color: #222;
}
.popup .form .form-element input[type="text"],
.popup .form .form-element input[type="password"]{
margin-top: 5px;
display: block;
width: 100%;
padding: 10px;
outline: none;
border: 1px solid #aaa;
border-radius: 5px;
}
.popup .form .form-element input[type="checkbox"]{
margin-right: 5px;
}
.popup .form .form-element button{
width: 100%;
height: 40px;
border: none;
outline: none;
font-size: 16px;
background: #222;
color: #f5f5f5;
border-radius: 10px;
cursor: pointer;
}
.popup .form .form-element a {
display: block;
text-align: right;
font-size: 15px;
color: #1a79ca;
text-decoration: none;
font-weight: 600;
}
Now we need to add our form, for that we have took a div
, in which we have added our label for email and password. Also, we have added input fields to these two labels. Then after, we have added another label for remember me
, also we have added a checkbox field. Then we have added a button for sign in, and lastly we have added an anchor for forgot password.
In CSS, we have customized these labels and input field. We have added styles like colors, font-size, font-weight etc. Also, we have customized our sign-in button with some basic styles like color. Then after we have adjusted the checkbox and anchor tag with some basic styles.
Now we have added some transition and animation for the form while it gets popup and pop out. Also, we have added our active class for popup class, in this we have added this transition and opacity to show up the form. Lastly, we have added our close button on the form. Here we will adjust the opacity
and top
property, so that we can see the form.
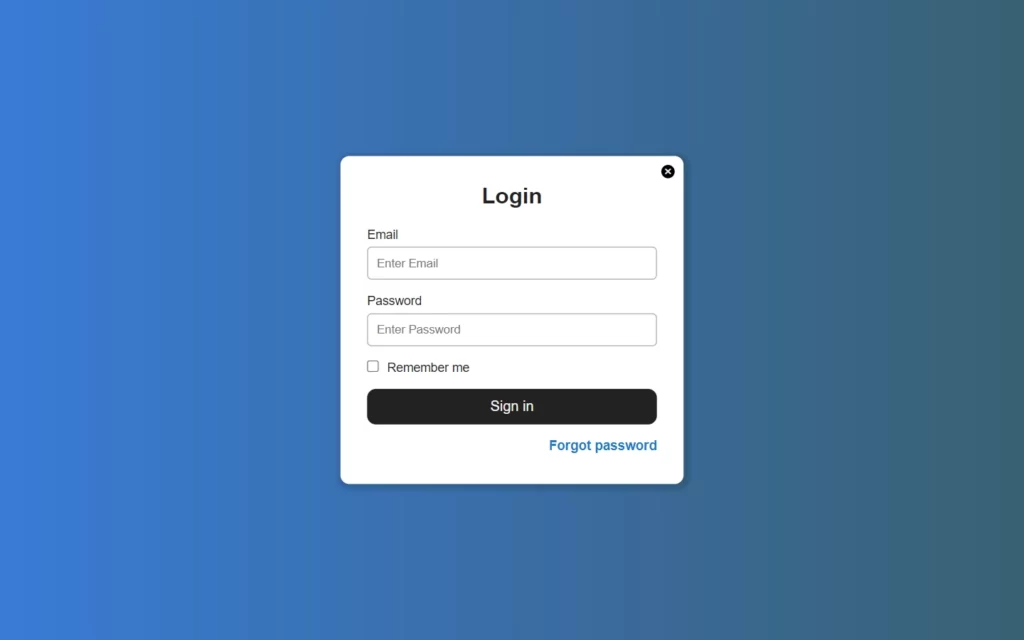
Adding Functionality to Popup and Pop out
<script>
document.querySelector("#show-login").addEventListener("click",function(){
document.querySelector(".popup").classList.add("active");
});
document.querySelector(".popup .close-btn").addEventListener("click",function(){
document.querySelector(".popup").classList.remove("active");
});
</script>
Now in JavaScript, we have selected our login button using its ID with querySelector
and we have added a click event on this button with a function. In this function we are just adding our active class in which we have adjusted opacity to 1 and also added animation.
Now for close button, we again fetched the close button using its class name, in this we have again added a click event with the function. In this function, we are just removing our active class.
Full Source Code to Make Popup Login Form using HTML, CSS & JavaScript
index.html
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Popup Login Form</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="center">
<button id="show-login">Login</button>
</div>
<div class="popup">
<div class="close-btn">×</div>
<div class="form">
<h2>Login</h2>
<div class="form-element">
<label for="email">Email</label>
<input type="text" id="email" placeholder="Enter Email">
</div>
<div class="form-element">
<label for="password">Password</label>
<input type="text" id="password" placeholder="Enter Password">
</div>
<div class="form-element">
<input type="checkbox" id="remember-me">
<label for="remember-me">Remember me</label>
</div>
<div class="form-element">
<button>Sign in</button>
</div>
<div class="form-element">
<a href="#">Forgot password</a>
</div>
</div>
</div>
<script>
document.querySelector("#show-login").addEventListener("click",function(){
document.querySelector(".popup").classList.add("active");
});
document.querySelector(".popup .close-btn").addEventListener("click",function(){
document.querySelector(".popup").classList.remove("active");
});
</script>
</body>
</html>
style.css
*{
margin: 0;
padding: 0;
box-sizing: border-box;
}
body{
background: linear-gradient(to right, #3a7bd5, #3a6073);
font-family: "Raleway", sans-serif;
height: 100vh;
}
.center{
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.center button{
padding: 10px 20px;
font-size: 15px;
font-weight: 600;
color: #222;
background: #f5f5f5;
border: none;
outline: none;
cursor: pointer;
border-radius: 5px;
opacity: 0;
}
.popup{
position: absolute;
top: 50%;
left: 50%;
opacity: 1;
transform: translate(-50%, -50%) scale(1.25);
width: 386px;
padding: 20px 30px;
background: #fff;
box-shadow: 2px 2px 5px 5px rgba(0,0,0,0.15);
border-radius: 10px;
transition: top 0ms ease-in-out 200ms,
opacity 200ms ease-in-out 0ms,
transform 200ms ease-in-out 0ms;
}
.popup.active{
top: 50%;
opacity: 1;
transform: translate(-50%, -50%) scale(1);
transition: top 0ms ease-in-out 0ms,
opacity 200ms ease-in-out 0ms,
transform 20ms ease-in-out 0ms;
}
.popup .close-btn{
position: absolute;
top: 10px;
right: 10px;
width: 15px;
height: 15px;
background: #000;
color: #eee;
text-align: center;
line-height: 15px;
border-radius: 15px;
cursor: pointer;
}
.popup .form h2{
text-align: center;
color: #222;
margin: 10px 0px 20px;
font-size: 25px;
}
.popup .form .form-element{
margin: 15px 0px;
}
.popup .form .form-element label{
font-size: 14px;
color: #222;
}
.popup .form .form-element input[type="text"],
.popup .form .form-element input[type="password"]{
margin-top: 5px;
display: block;
width: 100%;
padding: 10px;
outline: none;
border: 1px solid #aaa;
border-radius: 5px;
}
.popup .form .form-element input[type="checkbox"]{
margin-right: 5px;
}
.popup .form .form-element button{
width: 100%;
height: 40px;
border: none;
outline: none;
font-size: 16px;
background: #222;
color: #f5f5f5;
border-radius: 10px;
cursor: pointer;
}
.popup .form .form-element a {
display: block;
text-align: right;
font-size: 15px;
color: #1a79ca;
text-decoration: none;
font-weight: 600;
}
Output
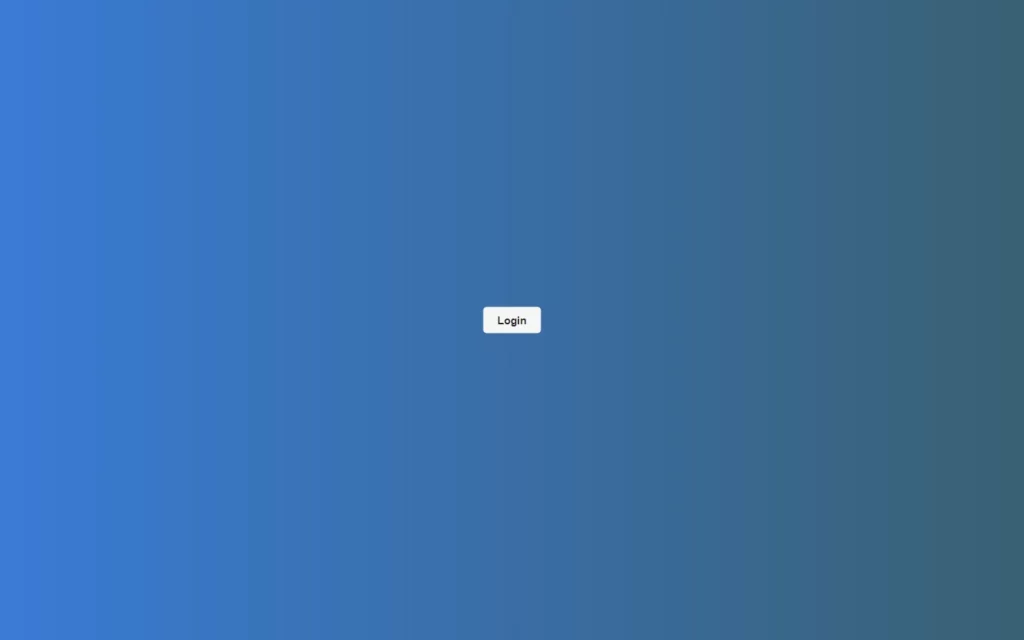
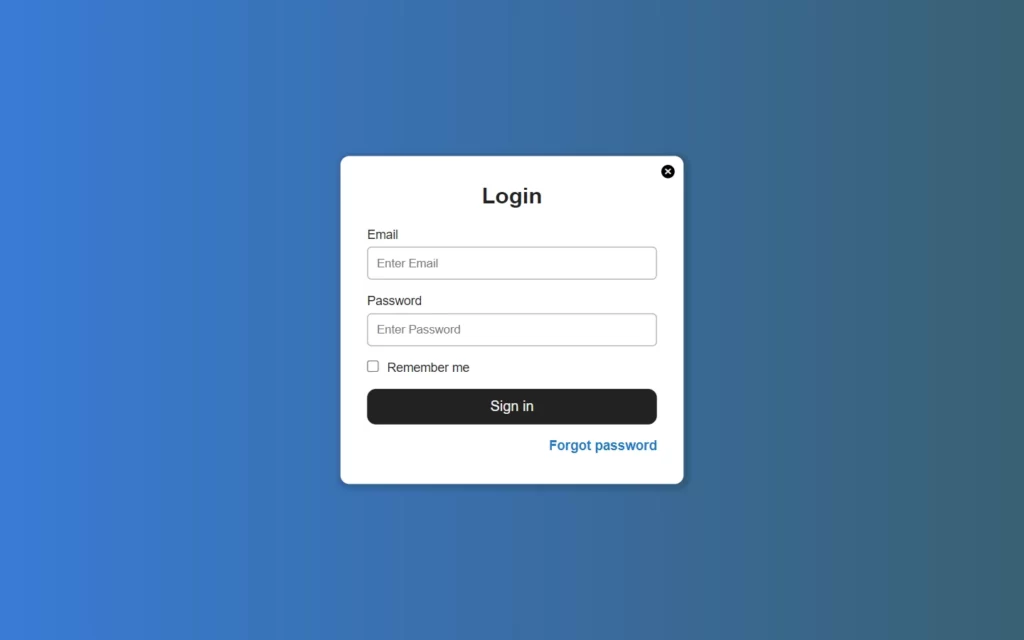
Check out the video reference here: