In this part, we are going to see that how we can compare objects in an array. So we will create some arrays, and we will compare those arrays to eliminate duplicate values. And also we will compare objects which are present in these arrays, so we can get a duplicate free array. It is a little bit hard to compare objects in JavaScript if you are a learner, so we will try to understand the problem as well as its solution.
Problem: Compare Objects in an Array
So first let’s see the problem which we are facing:
let obj1 = {name:"steven"};
let obj2 = {name:"steven"};
let arr1 = ["john", "alex", "bob", "kevin", obj1];
let arr2 = ["smith", "karl", "bob","kevin", obj2];
let newSet = new Set([...arr1,...arr2]);
let finalArr = [...newSet];
So in the above example, we have created two objects, which are actually the same, right? Then we have created two arrays with some duplicate values in these, also we have added these two objects in the array. Now we created a set which consists both arrays. So here, ideally, the set will not consist of any duplicate values, and also we are expecting it shouldn’t consist of duplicate objects as well.
But unfortunately, set is not able to determine object’s value, so obj1 and obj2 which has same values both will be in there in final set. Lastly, we have converted this set back to array. You can see the result below.
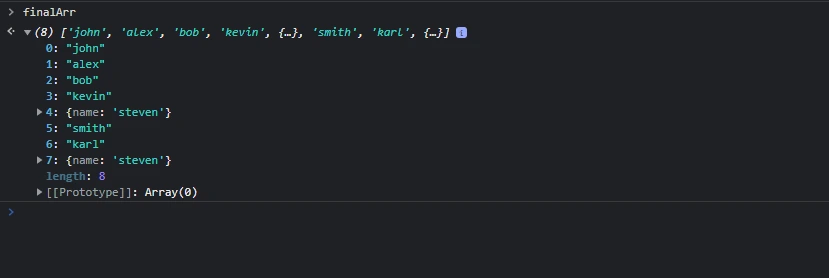
Solution: Compare Objects in an Array
As we have seen in the above condition, where set was not able to eliminate duplicate objects. So we have to make some logic with which we can easily compare object in an array.
Okay in below example, we have same things as above as arrays and objects along with same values. Now we have created a function which takes an array as parameter, here in the return statement we will apply map() method, so we can iterate through the whole array. In the map() method, we have added a callback function with parameter ele which refers to the value of the array, and it will consist each and every value present in the array in every iteration.
Now in this method, we are checking for object using if statement, also we will check where object should not be null because null also considered as an object, so it is required to eliminate it. If this condition becomes true which means we got our object then we will apply JSON.stringfy() method to get object’s values in string, so we can compare them easily.
After again we have created a set and in this set we call the arr3() function, with arrays as argument. So we can get the filtered arrays, and we just combining it in the set. Now as we know set does not contain duplicates, so here set will eliminate the duplicate objects because we have converted these objects to JSON string, so set can easily compare the objects.
let obj1 = {name:"steven"};
let obj2 = {name:"steven"};
let arr1 = ["john", "alex", "bob", "kevin", obj1];
let arr2 = ["smith", "karl", "bob","kevin", obj2];
let arr3 = function(arr){
return arr.map((ele)=>{
if (typeof(ele) === "object" && ele !== null){
return JSON.stringify(ele);
}else{
return ele;
}
})
}
let newSet = new Set([...arr3(arr1),...arr3(arr2)]);
let finalArr = [...newSet];
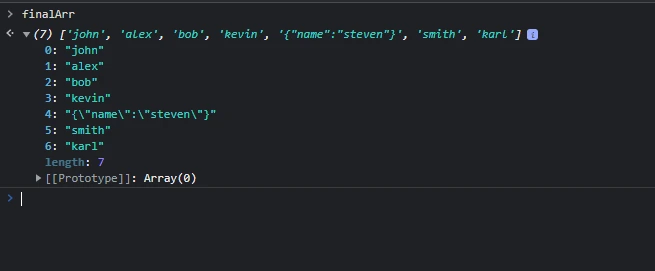