In this article, we will make Javascript convert date to long date format. For accomplishing this we will be using the toLocaleDateString()
function of JavaScript. It’s very simple to use this function but by default, this function will show the date in the format dd/m/yyyy. but we can provide optional parameter options to it which will be a map providing information about how to display various components of a date. Below is a list of the parameter names and types that are available when formatting time strings.
💡 Options key
1️⃣ day:
➜ The representation of the day.
➜ Possible values are “numeric”, “2-digit”.
2️⃣ weekday:
➜ The representation of the weekday.
➜ Possible values are “narrow”, “short”, “long”.
3️⃣ year:
➜ The representation of the year.
➜ Possible values are “numeric”, “2-digit”.
4️⃣ month:
➜ The representation of the month.
➜ Possible values are “numeric”, “2-digit”, “narrow”, “short”, “long”.
5️⃣ hour:
➜ The representation of the hour.
➜ Possible values are “numeric”, “2-digit”.
6️⃣ minute:
➜ The representation of the minute.
➜ Possible values are “numeric”, “2-digit”.
7️⃣ second:
➜ The representation of the second.
➜ Possible values are “numeric”, 2-digit”.
🌐 For different languages:
- “en-US”: For English
- “hi-IN”: For Hindi
➜ You can use more language options
var options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' };
var today = new Date();
console.log(today.toLocaleDateString("en-US"));
console.log(today.toLocaleDateString("en-US", options));
console.log(today.toLocaleDateString("hi-IN", options));
Output:
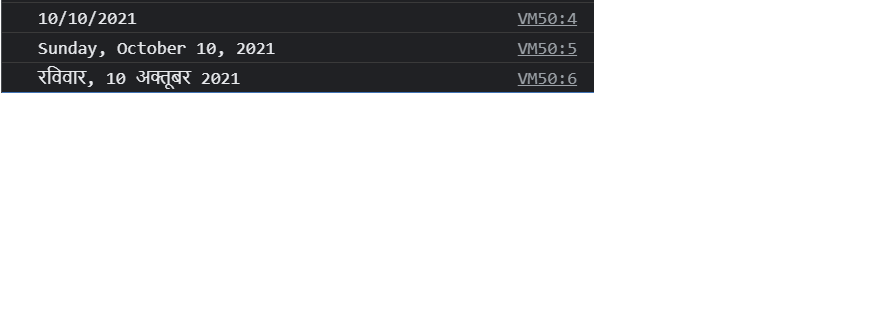
👉 Here are some JavaScript projects you should check out:
➜ Certificate Generator Website in JavaScript
➜ To-Do List using JavaScript,HTML and CSS (Beginners)
➜ Custom Pagination in JavaScript(beginners)
➜ Build A Dictionary app using JavaScript
➜ Bookmarker Application in javascript
➜ Speed Typing Game using JavaScript
➜ Simple snake game in JavaScript