➜ JavaScript is a great programming language to learn. So it is used everywhere. Web browsers like Chrome, Firefox, and Internet Explorer all use JavaScript. With the power of JavaScript, web programmers can transform web pages from simple documents into full-blown interactive applications and games. In This post, we will cover some javascript basic concepts. Every JavaScript programmer and web developer must know and master these.
Table of Contents
1. Scope
A simple definition for a scope in JavaScript.
➜ Scope determines the accessibility (visibility) of variables. In other words, Scope means variable access. In JavaScript there are two types of scope:
1) Global Scope
2) Local Scope
➜ Global Scope: A variable that is declared outside a function definition is a global variable. This means The global scope and its value are accessible and modifiable throughout your program.
➜ Local Scope: A variable this is declared inside a function definition is local. it’s far created and destroyed each time the function is executed. And It can not be accessed by using any code outside of the function.
Example 1.1: Global scope
var globalVar = 'hello Friends'; //global scope
function foo() {
console.log(globalVar); //output:hello Friends
}
foo();
console.log(globalVar); //output:hello Friends
Here globalVar variable should be global scope. It can access inside the function.
Example 1.2: Global scope- change the value
var globalVar = 'hello Friends'; //global scope
function foo() {
console.log(globalVar);
}
function foo1() {
globalVar = 'now change the value of global variable';
console.log(globalVar);
}
foo(); //hello Friends
foo1(); //now change the value of global variable
foo(); //now change the value of global variable
console.log(globalVar); //now change the value of global variable
➜ Here the first time globalVar variable value is “hello Friends”. After we create a function foo1 and inside that function, we changed the global variable value.
Example 1.3: Local scope
function foo() {
var localVar = 'hello friends';
console.log(localVar); //output:hello friends
}
console.log(localVar); //error
➜ Here localVar variable access only inside a function. We can not access outside functions. It is creating and destroying every time the function is executing.
Example 1.4: access local and global inside inner function
var globalVar = 'I am a global variable! You can access me anywhere';
function outerFunc() {
var outerVar = 'I am a variable scoped to outerFunc';
console.log(`globalVar from outerFunc: ${globalVar}`);
function innerFunc() {
var innerVar = 'I am a variable scoped to innerFunc';
console.log(`globalVar from innerFunc: ${globalVar}`);
console.log(`outerVar from innerFunc: ${outerVar}`);
}
innerFunc();
console.log(innerVar); //error
}
outerFunc();
//globalVar from outerFunc: I am a global variable! You can access me anywhere
//globalVar from innerFunc: I am a global variable! You can access me anywhere
//outerVar from innerFunc: I am a variable scoped to outerFunc
👉 more info about javascript basic concepts – Scope
2.IIFE
➜ An IIFE (Immediately Invoked Function Expression) is a JavaScript feature that runs as quickly as it is defined.
➜ It is a design pattern also known as Self Execution function that contains two main elements.
1) The first is the anonymous function with the lexical scope enclosed within the Grouping Operator ().
2) The second part creates the immediately invoked function expression () through which the JavaScript engine will directly interpret the function.
😀 Advantage Of IIFE:1)Avoid Creating Global Variable and Functions.2)As it doesn’t define variables and functions globally. So there will be no name conflicts.3)Scope is limited to that particular function.
syntax:
(function(){
//statements
})();
Example 2.1: IIFE
(function() {
var name = 'rocoderes';
console.log('Hello ' + name);
})();
//output:
//Hello rocoderes
Example 2.2: IIFE passing parameters
(function(a, b) {
console.log(a + b);
})(10, 20);
//output:
//30
Here we can pass the multiple parameters.
👉 more info about javascript basic concepts – IIFE
3.Hoisting
➜ Hoisting is a JavaScript mechanism were variables. And function declarations are move to the top of their scope before the code execution.
Example 3.1: Hoisting Function declarations
sum(10, 20);
function sum(a, b) {
console.log(a + b);
}
//this function is hoisted, so the output will be 30.
Example 3.2: Hoisting Function expressions
sum(10, 20);
var sum = function(a, b) {
console.log(a + b);
};
// Uncaught TypeError: sum is not a function
😄 What happens in the function expression scenario?
➜ The Variable declaration var expression is hoisting. So its assignment to the sum function is not hoisting. So the interpreter throws a TypeError. It sees the sum as a variable & not as a function.
Example 3.3: Hoisting Function In ES6
sum(10, 20);
const sum = (a, b) => {
console.log(a + b);
};
// Uncaught ReferenceError: Cannot access 'sum' before initialization
😄 What happens in ES6?
➜ The const bindings are not subject to Variable Hoisting. This means that const declarations do not move to the top of the current execution context. The variable is in a “temporal dead zone” from the start of the block until the initialization is processing.
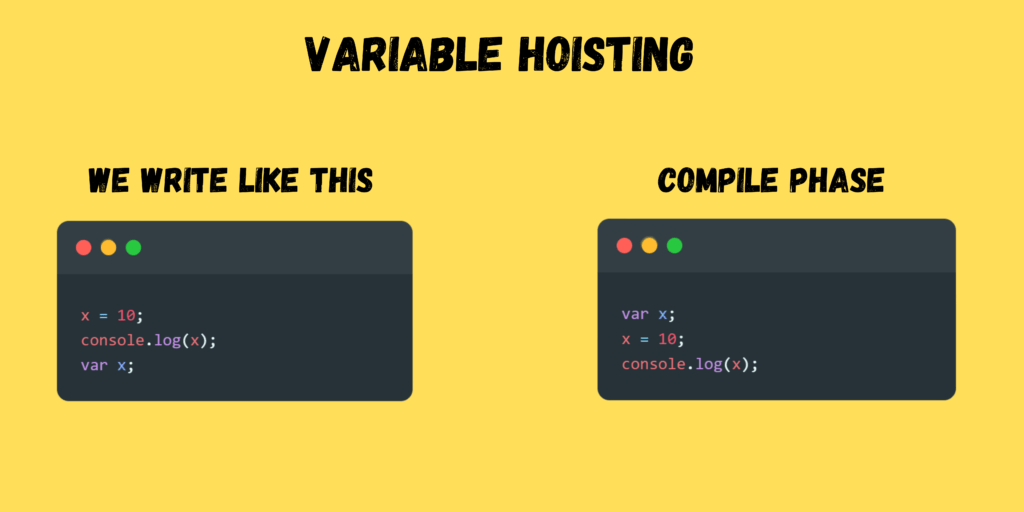
Example 3.4: Hoisting Variable
console.log(x);
var x = 1;
//Output
undefined
Example 3.5: Hoisting Variable interprets to
var x;
console.log(x);
var x = 1;
//Output
undefined;
JavaScript saves the x to the memory, console.log( x ); is call before it is define. So the result is undefined.
👉 more info about javascript basic concepts: JavaScript Visualized: Hoisting
4.Closures
➜ A closure is the combination of a function and the lexical environment within which that function is declare. Closure in action that is inner function can have access to the outer function variables/parameters as well as all the global variables.
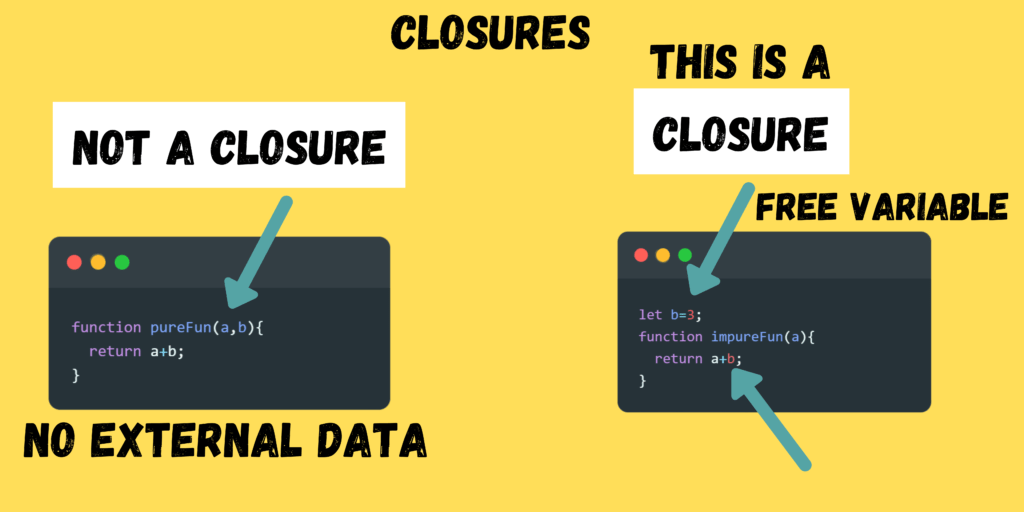
Example 4.1: OuterFunction and Inner Function
function outerFunc() {
var a = 20;
function innerFunc() {
console.log(a); // will output 20
}
innerFunc();
}
outerFunc();
➜ Here innerFunc has access to a And a is declaring of inner Func. In this example a was declaring in global parent Scope.
Example 4.2: Inner Function return
function foo() {
var x = 4; // declaration in outer scope
function bar() {
console.log(x); // outer scope is captured on declaration
}
return bar; // x goes out of scope after foo returns
}
var barWithX = foo();
barWithX(); // we can still access x
//Output:
4
➜ In the above example, when foo is call, its context is capture in the function bar. So even after it returns, a bar can still access and modify the variable x. The function foo, whose context is capture in another function, is calling to be a closure.
Example 4.3: Private Data
Lets us do some interesting things. such as defining “private” variables that are visible only to a specific function or set of functions.
function makeCounter() {
var counter = 0;
return {
value: function() {
return counter;
},
increment: function() {
counter++;
}
};
}
var a = makeCounter();
var b = makeCounter();
a.increment();
console.log(a.value());
console.log(b.value());
//Output:
1
0
➜ When makeCounter() is calling, a snapshot of the context of that function is saved. So all code inside makeCounter() will use that snapshot in their execution. Two calls of makeCounter() will thus create two different snapshots, with their own copy of the counter.
👉 more info about javascript basic concepts – JavaScript Closures
5.Callback
➜ Any Function that is passing as an argument is called a callback function. A callback is a function that is to be executing after another function has finished executing – hence the name is ‘callback’.
Note that when passing a callback function as a parameter to another function. We are only passing the callback function definition.
🤔 why callback function?
➜ JavaScript is an event-driven language. This means the instead of waiting for a response before moving on. JavaScript will keep executing while listening for other events. So callbacks are a way to make sure certain code doesn’t execute until other code has already finished execution.
Example 5.1: callback function
function talk(callback){
callback()
}
function sayHi(){
console.log("hi....");
}
talk(sayHi);
/*----------output-------------- */
hi....
Note: when you pass a function as an argument, remember not to use parenthesis.
Right: talk(sayHi)
Wrong: talk(sayHi())
Example 5.2: callback function Example 2
const personOne=(friend,callfriend)=>{
console.log(`I am Busy Right Now. I am talking to ${friend}. I will call you later `);
callfriend();
}
const personTwo=()=>{
console.log("Hey whats up ✋✋✋");
}
personOne("rocoderes",personTwo)
/*---------Output--------- */
I am Busy Right Now. I am talking to rocoderes. I will call you later
Hey whats up ✋✋✋
➜ There are two kinds of callback functions:
1) synchronous and 2) asynchronous
(1) synchronous
Example 5.3: synchronous
function show(){
console.log("i am show function");
}
function greet(callback){
callback()
}
greet(show);
console.log("End..");
/*---------Output--------- */
i am show function
End..
synchronous: It waits for each operation to complete. After that it executes the next operation.
(2) asynchronous
Example 5.4: asynchronous
setTimeout(function show(){
console.log("i am show function");
},5000)
console.log("End..");
/*---------Output--------- */
End..
i am show function
asynchronous: it never waits for each operation to complete. Rather it executes all operations in the first GO only.
🤔 why should you use Callback Functions?
➜ write more clean and readable code.
➜ DRY – Do not Repeat Yourself.
➜ Have Better maintainability.
➜ Understand the use of many JavaScript libraries that are using callbacks.
👉 more info about javascript basic concepts – JavaScript Callbacks
6)Promises
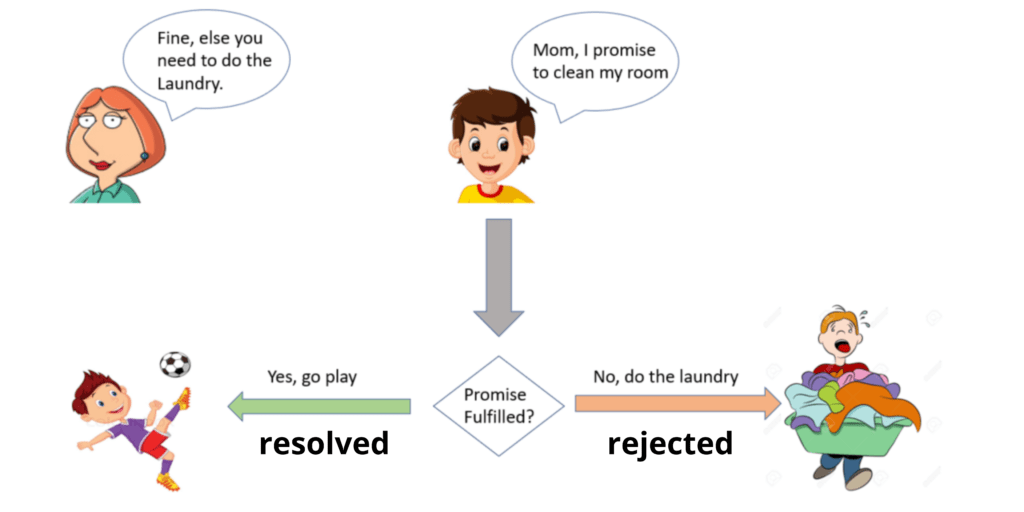
➜ A Promise is an object representing the eventual completion or failure of an asynchronous operation. A JavaScript Promise object contains both the producing code and calls to the consuming code. It is used to deal with Asynchronous operations in JavaScript.
➜ In a simple way Promise is an action that guarantees a result in the future. The result could be the expected one(positive) and if anything goes wrong. The result will be something that was not negative.
➜ Promises are used for handling asynchronous operations also called blocking code. Examples of which are DB, I/O, or API calls. Which are carring out by the executor function. Once that completes it either calls resolve on success or reject function on error.
👉 A Promise is in one of these states:
pending ➜ The operation has not yet been completing. And the promise is pending.
fulfilled ➜ The operation has finishing, and the promise is fulfilled with a value.
rejected ➜ An error has occurred during the operation. And the promise is rejecting for a reason.
🤔 How Promise works
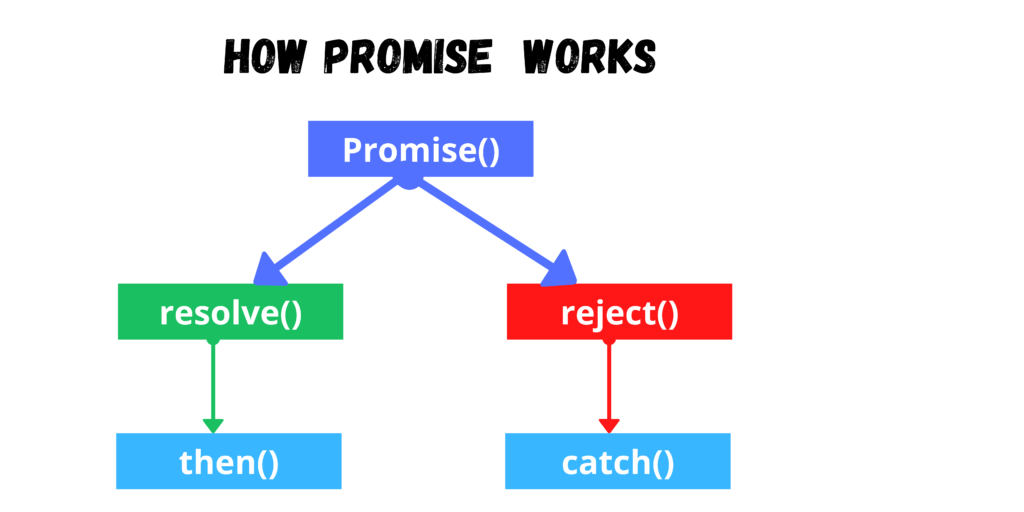
➜ A pending promise can either be resolved with a value or rejected with a reason(error).
➜ when either of these options happens, the associated handlers queued up by a promise then() method is called.
➜ A Promise is said to be settled if it’s either Resolved or Rejected, but not pending.
Example 6.1: Lets talk a look at how a student promising to return all the books he took from library looks like in JavaScript.
const promiseToReturnTheBook=new Promise((resolve,reject)=>{
const isReturned=true;
if(isReturned){
resolve("Returned All The Books")
}else{
reject("Dint Return All Books")
}
})
promiseToReturnTheBook.then((result)=>{
console.log(`Resolved : ${result}`);
}).catch((error)=>{
console.log(`Rejected : ${result}`);
})
//output
//Resolved : Returned All The Books
Therefore, our promise object here once called will resolve to return “Returned All The Books” as its response. Suppose isReturned set to false. Then the promise here will get rejecting and return “Dint Return All Books” as its response.
👉 Explanation:
➜ The Promise constructor takes in an executor function as an argument.
➜ The Executor function takes in two callback functions resolve() and reject().
➜ resolve() is called on success of the operation.
➜ reject() is called on failure of the operation.
➜ consuming a Promise.
Promise. then() only triggers if the promise is resolved(Status: Success)
Promise. catch() only triggers if the promise is rejected(Status: Failed)
😀 Benefits of promises
➜ improves Code Readability
➜ Better handling of asynchronous operations
➜ Better Flow of control definition in asynchronous logic
➜ Better Error Handling
👉 more info about javascript basic concepts – JavaScript Promises
7) Async Await
➜ Async Functions enables us to write promise-based code as if it were synchronous but without blocking the execution thread. It operates asynchronously via the event loop.
➜ Async Functions are instances of the Async Function constructor. And the await keyword is permitting within them.
🚀 async keyword
➜ putting the keyword async before a function tells the functions to return a Promise.
➜ it doesn’t matter what the function does, if it’s async it will always return a Promise.
➜ it doesn’t need to return a value. It can return void. what that happens, it’s equivalent to a Promise resolving without a value.
🚀 await keyword
➜ The await keyword can be used only inside an async function.
➜ It informs the javascript runtime environment that it should hold up program execution until the associated Promise resolves.
➜ We can use await when calling any function that returns a Promise, including web API functions.
Example 7.1: let’s talk a look at how a student promising to return all the books. He took from the library looks like in JavaScript using async-await.
const promiseToReturnTheBook=new Promise((resolve,reject)=>{
const isReturned=true;
if(isReturned){
resolve("Returned All The Books")
}else{
reject("Dint Return All Books")
}
})
async function getData(){
const response=await promiseToReturnTheBook;
console.log(response);
}
getData();
//output:
Returned All The Books
Example 7.2: try & catch block
const promiseToReturnTheBook=new Promise((resolve,reject)=>{
const isReturned=false;
if(isReturned){
resolve("Returned All The Books")
}else{
reject("Dint Return All Books")
}
})
async function getData(){
try{
const response=await promiseToReturnTheBook;
console.log(response);
}
catch(e){
console.log(e);
}
}
getData();
//output:
Dint Return All Books
Example 7.3: Fetch API Data using Async-await
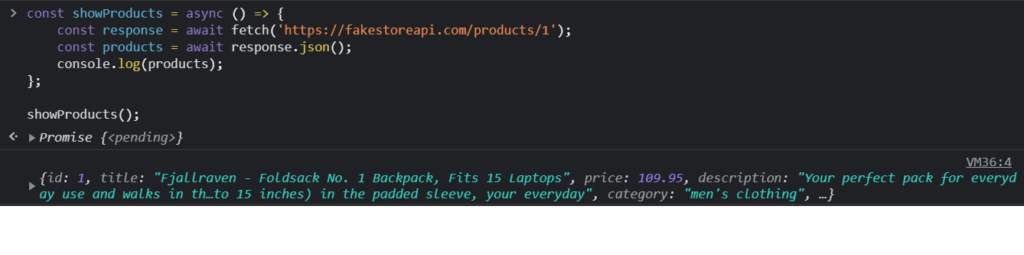
Here we create showProducts function which is fat Arrow function. And we fetch the data from API and store it in response variable.
😀 Advantages of using Async Await
➜ Error handling: we can handle both asynchronous and synchronous errors using try/catch in as an async function.
➜ Clean code: it really makes the code cleaner and easy to read
➜ Web API
👉 more info about javascript basic concepts – JavaScript Async
8) JavaScript Prototype
➜ In JavaScript, a prototype is used to add properties and methods to a constructor function. And objects inherit properties and methods from a prototype.
➜ you can create an object in JavaScript using an object constructor function.
Example 8.1: constructor function
//constructor function
function Human(){
this.name="Brock",
this.age = 23,
this.canWalk = true;
this.canSpeak = true;
}
//creating objects
const human1 = new Human();
const human2 = new Human();
➜ In the above example, the function Human() is an object constructor function. We have created two objects human1 and human2 from it.
Example 8.2: Add Properties
/ constructor function
function Human () {
this.name = 'Brock',
this.age = 23,
this.canWalk = true;
this.canSpeak = true;
}
// // creating objects
const human1 = new Human();
const human2 = new Human();
// adding property to constructor function
Human.prototype.gender = 'male';
// prototype value of Human
console.log(Human.prototype); //output: Human { gender: 'male' }
// inheriting the property from prototype
console.log(human1.gender); //output: male
console.log(human2.gender); //output: male
➜ In the above program, we have added a new property gender to the Human constructor function using:
Human.prototype.gender = ‘male’;
➜ Then object human1 and human2 inherits the property gender from the prototype property of the Human constructor function.
➜ Hence, both objects human1 and person2 can access the gender property.
Example 8.3: Add Methods
function Human() {
this.canWalk = true;
this.canSpeak = true;
}
// creating objects
const human1 = new Human();
const human2 = new Human();
// adding a method to the constructor function
Human.prototype.greet = function (name) {
if (this.canSpeak) {
console.log('Hi, I am ' + name);
} else {
console.log('Sorry i can not speak');
}
};
human1.greet("Steve") //output: Hi, I am Steve
human2.greet("Tony") //output: Hi, I am Tony
console.log(human1.__proto__); //output: Human { greet: [Function] }
➜ In the above program, a new method greet is adding to the Human constructor function using a prototype.
➜ In the above example, a human1 object is used to access the prototype property using __proto__.
👉 more info about javascript basic concepts – JavaScript Object Prototypes
9) ES6 classes
➜ A JavaScript class is a blueprint for creating objects. A class encapsulates data and functions that manipulate data. Object-Oriented Programming (OOP) can be a great way to organize your projects. Introduced with ES6, the javascript class syntax makes OOP easier.
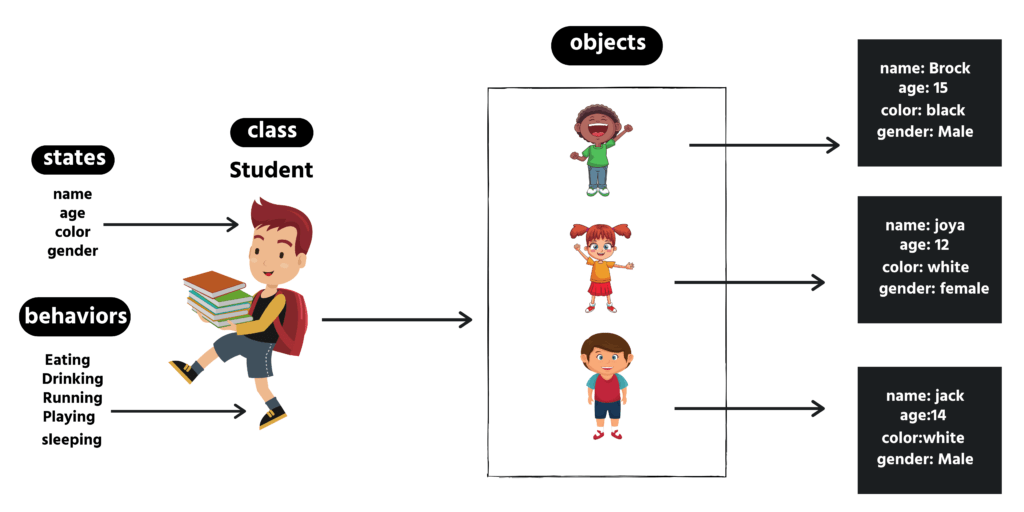
Example 9.1: Basic Es6 class syntax
class Employee {
constructor(name) {
this.name = name;
}
getName() {
return this.name;
}
}
➜ Then use the new Employee() to create a new object with all the listed methods. The constructor() method is call automatically by new. So we can initialize the object there.
Example 9.2
class Employee {
constructor(name) {
this.name = name;
}
getName() {
console.log(this.name);
}
}
const emp=new Employee('Tony')
emp.getName() //output: Tony
🚀 this Keyword
The JavaScript keyword “this” refers to the object it belongs to. When a new Employee(‘Tony’) is called:
➜ A new object is created.
➜ The constructor runs with the given argument and assigns it to this.name.
➜ Then we can call object methods, such as emp.getName().
➜ These properties and methods can be accessed using the dot( . ) operator
➜ Constructor: This is a special method for initializing an instance of that class. So what that means is that whenever we create a new instance of the class. It will invoke the constructor. This is a great place to set some properties and assign parameters.
🚀 Static Method
➜ A static method is something you can call without an instance of the class.
class Employee {
static salary=20000
constructor(name) {
this.name = name;
}
getName() {
console.log(this.name);
}
static employeeInfo(code){
console.log(`Employee Code: ${code}`);
}
}
const emp=new Employee('John')
emp.getName() //output: John
Employee.employeeInfo(120) //output: Employee Code: 120
console.log(Employee.salary); //output: 20000
➜ In the example above, the properties that use the keyword “static” belong to the class. To determine if a field or method belongs to the class or instantiated object. You simply call it using the syntax: className.memberName
👉 Class Methods: Getters and Setters
➜ get: a method used to retrieve a property’s value.
➜ set: a method used to assign a property’s value.
class Employee {
constructor(name) {
this.name = name;
}
get Name() {
console.log(this.name);
}
set Name(n){
this.name=n;
}
}
const emp=new Employee('Tony')
emp.Name //output: Tony
emp.Name="Steve"
emp.Name //output: Steve
☠️ Note: when you use getter and setter don’t use parentheses when invoked
➜ The get method is invoking with: emp.Name;
➜ The set method is invoking with: emp.Name=”Steve”;
🌠 Inheritance
➜ Inheritance is the ability to create new entities from an existing one. The class that is extended for creating newer classes is referred to as the superclass/parent class. While the newly created classes are called subclass/child class.
➜ A class can extend the properties and methods from another class using the keyword ‘extends’.
➜ The reserved ‘super’ keyword is for making super-constructor calls and allows access to parent methods.
class Employee {
name=''
constructor(id) {
this.id = id;
}
setName(firstname,lastname) {
this.name=`${firstname} ${lastname}`
}
prinDetails(){
console.log(`Name is: ${this.name}`);
console.log(`Id is: ${this.id}`);
}
}
class Manager extends Employee{
projects=[]
constructor(id){
super(id)//call the super class constructor and pass in the id parameter
}
setProject(projectname){
this.projects.push(projectname)
}
printProjects(){
console.log("projects: ",this.projects);
}
}
const manager=new Manager(120);
manager.setName('Tony','Stark');
manager.prinDetails() //output: Name is: Tony Stark Id is: 120
manager.setProject('calculator')
manager.setProject('facebook')
manager.setProject('google')
manager.printProjects() //output: projects: [ 'calculator', 'facebook', 'google' ]
➜ We have declared a class Employee. The constructor of the class contains one argument id, respectively. The keyword ‘this’ refers to the current instance of the class.
➜ By using the extends keyword, we can create a new class Manager that shares the same characteristics as its parent class Employee. So, we can see that there is an inheritance relationship between these classes.
➜ If there is a constructor present in the subclass, it needs to first call super() before using.
👉 more info about javascript basic concepts – JavaScript ES6 Classes
10) currying
➜ Currying a function is the process of taking a single function of multiple arguments. And decomposing it into a sequence of functions that each take a single argument.
😀 let us take the following simple example:
function sum(x,y,z){
return x+y+z;
}
console.log(sum(3,2,2)); //output: 7
here function sum taking in parameters x,y & z and returning the sum.
😀 By Apply Currying the same can be broken into a function return another function.
function sum(x){
return function(y){
return function(z){
return x+y+z;
}
}
}
console.log(sum(3)(2)(2));
➜ Currying is a transform that makes sum(x, y, z) callable as sum(x)(y)(z).
🤔 why is it useful ?
➜ currying helps you to avoid passing the same variable again and again.
➜ little pieces can be configured and reused with ease.
➜ It helps to create a higher-order function.
➜ It is useful in event handling.
👉 more info about javascript basic concepts – JavaScript Currying
11) apply, call, and bind methods
1) call() method
➜ The call() method calls a function with a given value and arguments provided individually.
function personInfo() {
console.log(`${this.name} ${this.age}`);
};
const person1 = {
name: 'Jon',
age: 25
};
personInfo(); // Output 1: undefined undefined
personInfo.call(person1); // output: Jon 25
personInfo.call({ name : 'Brock', age :55 }); //output: Brock 55
➜ We have a function personInfo() that will try to access this and console name and age. We have outputs:
- We didn’t use the call() method. So this by default will refer to the window object. Window object doesn’t have any properties like name or age. Hence, we get ‘undefined undefined’.
- This time we use call() and pass an object that has the required properties. This will now be the person. Hence, we get an output Jon 25.
👉 You can also pass additional arguments in call()
function personInfo(city,state) {
console.log(`${this.name} is from ${city}, ${state}`);
};
const person = {
name: 'Jon'
}
personInfo.call(person,'Los Angeles', 'California') //output: Jon is from Los Angeles, California
2) apply() method
➜ The apply() method calls a function with a given value, and arguments are provided as an array.
function sum(num1, num2) {
console.log(this + num1 + num2);
}
sum.call(2, 3, 4); // Output: 9
sum.apply(2, [3, 4]); // Output: 9
➜ It’s exactly the same as call(). The only difference is call() takes arguments individually but apply() takes them as an array. 😆 That’s it.
3) bind() method
➜ The bind() method creates a new function. That, when called, has this keyword set to the provided value. With a given sequence of arguments preceding any providing when the new function is called.
function getPerson(person) {
console.log(`${person} is from ${this.state}.`);
}
getPerson.call({ state: "California" }, "Max"); // Output 1: Max is from California.
const personFromCalifornia = getPerson.bind({ state: "California" });
personFromCalifornia("Max"); // Output 2: Max is from California.
personFromCalifornia("Ben"); // Output 3: Ben is from California.
➜ We use call() and pass first argument { state : ‘California’ } and second argument will be person.
➜ We try to get the same output as 1 using bind(). Using bind() we can bind this value to some function and get another function in return. In our case, we bind it with { state: ‘California’ } and store the returned function in personFromCalifornia. Now, when we call personFromCalifornia, we just need to pass person argument. It will already have this value.
➜ Just call the same function again with a different person.
😄 differences between call() and bind() ?
➜ call() gets invoked immediately whereas bind() returns a function that we can invoke later.
➜ call() takes additional arguments but bind() does not.
➜ call() doesn’t make a copy of the function unlike bind().
👉 more info about javascript basic concepts apply, call, and bind methods
12) map, filter and reduce method
1) map() method
➜ The map() method creates a new array with the results of calling a provided function on every element in the calling array.
➜ map calls a provided callback function once for each element in an array. in order, and returns a new array from the results.
☠️ Note: this method does not change the original array.
👉 syntax: Array.map(function (currentValue, index, arr), thisValue)
const array = [1, 4, 9, 16, 25];
let newArr = array.map((curElem, index, arr) => {
return curElem*2;
})
console.log("original array: ",array);
console.log("After apply map method: ",newArr);
/*
------output------
original array: [ 1, 4, 9, 16, 25 ]
After apply map method: [ 2, 8, 18,32, 50 ]
*/
2) filter() method
➜ This method returns a subset of the given array
➜ It executes the given function for each item in the array and depending on whether the function returns true or false it keeps that element in or filters it out.
➜ If true the element is kept in the result array.
➜ If false the element is excluded from the result array.
☠️ Note: filter() method does not change the original array.
function isGreaterThanTwo(item){
return item>2
}
const prices = [200, 300, 350, 400, 450, 500, 600];
const priceTag = prices.filter((currVal, index, array) => {
return currVal < 400
})
console.log(priceTag)//output: [ 200, 300, 350 ]
➜ in the above example callback function check if the value of the given currVal is less than 400
➜ the result is a new array with only [ 200, 300, 350 ].
3) reduce() method
➜ the reduce() method executes a reducer function on each element of the array, resulting in a single output value.
👉 syntax: Array.reduce(callback,inittialValue)
➜ The result is called an accumulator.
➜ Here initial value is optional. It’s the initial value of the accumulator. if it’s not provided it’s the default value of the array first element.
const numbers=[2,3,1,4,5,6]
const sum=numbers.reduce((accumulaotr,currentValue)=>{
return accumulaotr+currentValue
})
console.log(sum)//output: 21
👉 more info about javascript basic concepts – map(), filter() and reduce() in JavaScript
13) High Order Functions
➜ Any function which takes a function as an argument or any function which returns a function.
Example 13.1
function greet(name,greetMessage){
console.log(`Hello 😄, ${name}`);
greetMessage(name)
}
function greetMessage(name){
console.log(`${name}: How are you 😀`);
}
greet('rohan',greetMessage);
/*
---output---
Hello 😄, rohan
rohan: How are you 😀
*/
➜ in the above code, greet is high order function, as it takes greetMessage function as an argument
Example 13.2
function multiply(time){
return function(value){
return value*time
}
}
const mul=multiply(11);
console.log(mul(10)) //output: 110
➜ Here multiply is high order function, as it returns a function.
🚀 Built-in high order functions in JavaScript:
➜ map()
➜ filter()
➜ reduce()
😄 differences between callback and high order functions ?
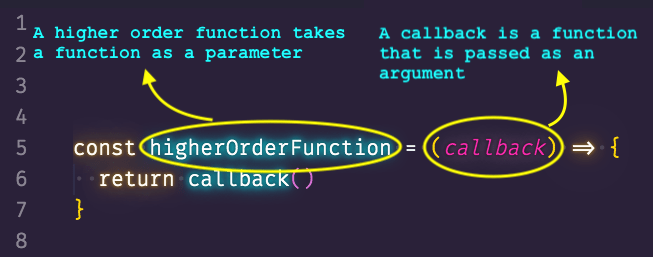
👉 more info about javascript basic concepts – Higher Order Function in Javascript
14)Destructuring and spread operator(…)
🚀 Destructuring in javascript
➜ Destructuring is a way to create variables from existing values in arrays and objects .its one of the coolest JavaScript features.
Destructuring arrays
➜ in arrays, you can have any name for variables. But the same is not true in the case of objects. In objects, you need to use variable names same as properties of the object.
const user=["Jon",22]
const [name,age]=user;
console.log(name,age) //output: jon 22
Destructuring Objects
const user={
name:"Jon",
age:22,
gender:"male"
}
const {name,age,gender}=user
console.log(name,age,gender) //output: Jon 22 male
🤔 Why Destructuring ?
➜ the first is that your code is more readable. if you destructure an object at the top of a function or code block, it is clear to the reader what variables you are going to be used.
➜ better performance
👉 more info about Destructuring
🌠 spread operator(…)
➜ The spread operator(…) can expand another item by split an iterable element like a string or an array into individual elements.
1.Inserting arrays
const fruits=['🍉','🍊','🍓']
const moreFruits=['🍋',...fruits,'🍎','🍐']
console.log(moreFruits); //output:['🍋','🍉','🍊','🍓','🍎','🍐']
2.copying array
const fruits=['🍋','🍉','🍊','🍓']
const newFruits=[...fruits]
console.log(newFruits); //output: ['🍋','🍉','🍊','🍓']
3.Concatenating arrays
const arr1=['🍉','🍊','🍓']
const arr2=['🍎','🍐','🍌']
const result=[...arr1,...arr2]
console.log(result); //output: ['🍉','🍊','🍓','🍎','🍐','🍌']
4.Spreading elements on function calls
const fruitStand=['🍎','🍐','🍌']
const sellFruit=(f1,f2,f3)=>{
console.log(`Fruits for sale: ${f1} , ${f2} , ${f3}`);
}
sellFruit(...fruitStand) //output: Fruits for sale: 🍎 , 🍐 , 🍌
5.String to array
const str='hello JavaScript'
const chars=[...str]
console.log(chars);
//output: ["h", "e", "l", "l", "o", " ", "J", "a", "v", "a", "S", "c", "r", "i", "p", "t"]
6.Combining objects
const name={
firstname:"jon",
lastname:"snow"
}
const bio={
age:26,
country:"USA"
}
const person={...name,...bio}
console.log(person);
//output: { firstname: 'jon', lastname: 'snow', age: 26, country: 'USA' }
15) JavaScript Module Pattern
➜ In a module, all the variables defined are visible only in the module. Methods in a module have scope and access to the shared private data and private methods with closure in JavaScript.
function shop(){
const fruit='mango'
const vegetable='carrot'
return {
buyFruit:()=>fruit,
buyVegetable:()=>vegetable
}
}
shop().buyFruit() //output: mango
shop().buyVegetable() //output: carrot
console.log(shop().buyFruit());
console.log(shop().buyVegetable());
➜ In the above example, shop buying groceries of fruit and vegetable should not be modifiable for any reason
➜ The function shop() returns an object that contains other functions that return a property of the function itself. from the perspective of the scope out of the shop, it can’t access any variables inside.
➜ A closure is easy to cause a memory leak if you overuse it in the wrong way, but you can encapsulate the function safely.
➜ to modify the variables, all you need to do is just add getter and setter functions in the return object.
return {
getFruit:()=> fruit,
getVegetable:()=> vegetable,
setFruit: name=> fruit= name,
setVegetable: name=> vegetable= name
}
😀 Advantages of the module pattern
➜ Namespacing
➜ maintainability
➜ reusability
➜ supports private data
➜ Enables unit testability of code
👉 Here are some JavaScript projects you should check out:
➜ Certificate Generator Website in JavaScript
➜ To-Do List using JavaScript,HTML and CSS (Beginners)
➜ Custom Pagination in JavaScript(beginners)
➜ Build A Dictionary app using JavaScript
➜ Bookmarker Application in javascript
➜ Speed Typing Game using JavaScript
➜ Simple snake game in JavaScript
I simply could not depart your web site prior to suggesting that I
really loved the usual info an individual supply
to your visitors? Is going to be again frequently in order to investigate cross-check new posts
Link exchange is nothing else however it
is only placing the other person’s website link on your page at proper place and other person will also do similar in favor of you.
This is a topic close to my heart cheers, where are your contact details though?