In this article, we will make full screen image slider in JavaScript with owl carousel. In this, we will have an image and some information in the different slides, and these slides we are going to control with the help of owl carousel and JavaScript. We will autoplay these slides on some certain amount of time, and these also, going to be controlled with mouse as well. Owl carousel will make this thing very easy. This is going to be very easy and beginner-friendly project, let’s just make this step-by-step.
Pre-requisites to Make Full Screen Image Slider in JavaScript with owl carousel
- Basic knowledge of HTML.
- Good knowledge of CSS.
- Good knowledge of JavaScript.
- Basic knowledge of jQuery and Owl Carousel.
Creating HTML Skeleton
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>two way slider with owl</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<link rel="stylesheet" href="owl.carousel.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="owl.carousel.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<br><br><br><br>
<section class="hero">
<div class="container">
<div class="row">
<div class="col-md-5">
<div class="hero-img">
<img src="huk4.jpg" alt="" class="img-responsive">
</div>
</div>
<br><br>
<div class="col-md-7">
<div class="content">
<h3><b>About Me</b></h3>
<br>
<span class="sub-title">
UI/UX Designer & Web Developer
</span>
<p class="pb-10">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Ipsa facere adipisci architecto eligendi ratione optio molestiae amet unde eius impedit?
</p>
<br>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eaque explicabo inventore eligendi earum debitis natus.
</p>
<br><br><br>
<div class="info">
<ul>
<li>
<span class="title">Name</span>
<span class="value">Jerry Kon</span>
</li>
<li>
<span class="title">Email</span>
<span class="value">whoweare@gm.com</span>
</li>
<li>
<span class="title">Cell</span>
<span class="value">9988999999</span>
</li>
<li>
<span class="title">Phone</span>
<span class="value">9988999999</span>
</li>
<li>
<span class="title">Age</span>
<span class="value">29Yrs</span>
</li>
</ul>
<div class="social-icon">
<a href="">
<span><i class="fa fa-facebook"></i></span>
</a>
<a href="">
<span><i class="fa fa-twitter"></i></span>
</a>
<a href="">
<span><i class="fa fa-linkedin"></i></span>
</a>
<a href="">
<span><i class="fa fa-behance"></i></span>
</a>
<a href="">
<span><i class="fa fa-youtube"></i></span>
</a>
</div>
</div>
</div>
<div class="content">
<h3>About My Skills</h3>
<br>
<span class="sub-title">UI/UX Designer, Photographer & Developer</span>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Hic optio, aperiam! Provident quis enim nemo earum unde ducimus culpa quidem.
</p>
<br><br>
<div class="row">
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-android"></i>
<div class="feature-content">
<h5>Graphic</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-edit"></i>
<div class="feature-content">
<h5>Web Design</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-camera-retro"></i>
<div class="feature-content">
<h5>Photography</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-code"></i>
<div class="feature-content">
<h5>Development</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
</div>
<div style="margin-top: 10px;"></div>
<div class="mt-10">
<a href="">
<span class="btn btn-danger">Contact me</span>
</a>
</div>
</div>
</div>
</div>
</div>
</div>
</section>
</body>
</html>
In the HTML, firstly, we have added required files and CDNs. We added bootstrap CDN to make our responsive, then we have added font-awesome CDN, so that we can use icons from the library. After that, we have added jQuery, which is required when we are going to use JavaScript. And lastly, we have added our owl carousel file, with which we are going to add slides.
Now let’s make our content, for this we have added a div
, in this we have an image. After that we have added a section in which we have added some headings and paragraph with some information. Then in this section, we have added some social media icons and basic information as a list.
Now we have added another div
for another section, in this we have again added some heading and information, and also we have added some icons for android, camera icon, pencil icon etc. using font-awesome library. After that, we have added some more details with these icons, and lastly, we have added a button for contact us.
Here we don’t need to resize the image, because we use bootstrap, so the image will be adjusted automatically.
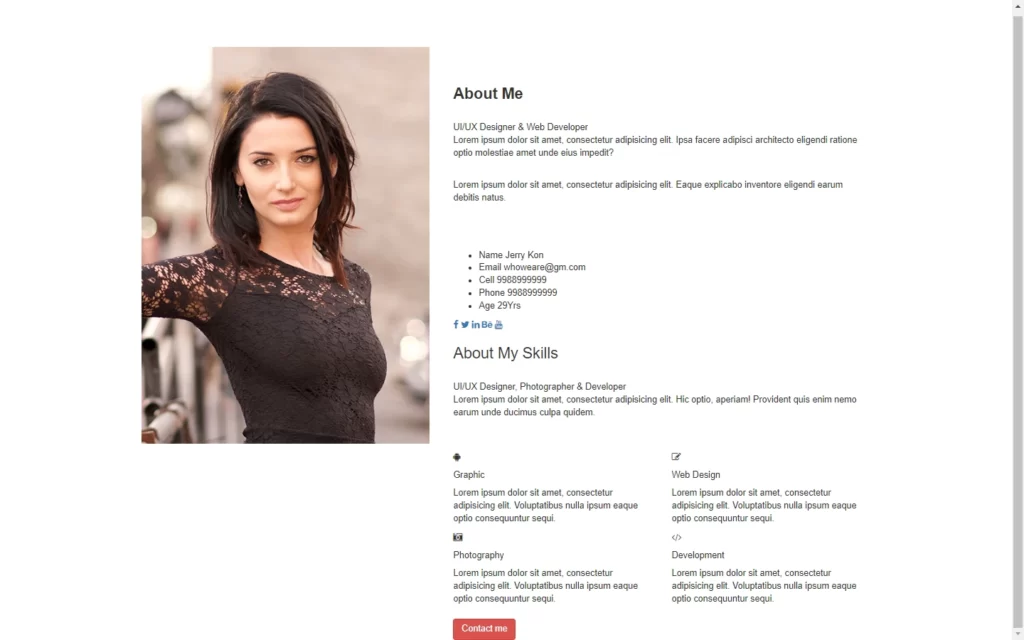
Adding Styles to All Elements
<style>
body{
font-family: tahoma;
}
.hero-img img{
border: 4px solid black;
}
.hero .content h3{
font-size: 800;
margin-bottom: 5px;
}
.hero .content .sub-title{
color: rgba(244,67,54,0.7);
letter-spacing: 2px;
font-size: 16px;
font-weight: bold;
margin-bottom: 20px;
}
.hero-content .info li{
width: 48%;
display: inline-block;
margin-bottom: 15px;
margin-left: -35px;
}
.hero .feature-box{
position: relative;
padding-left: 70px;
margin: 10px 0;
}
.hero .feature-box i{
font-size: 28px;
position: absolute;
left: 0;
width: 50px;
height: 50px;
text-align: center;
border-radius: 4px;
line-height: 50px;
color: white;
background: #343434;
}
.hero .feature-box h5{
color: #343434;
font-size: 16px;
font-weight: 600;
letter-spacing: 1px;
margin: 0 0 10px;
display: inline-block;
vertical-align: top;
width: 100%;
}
.social-icon .fa{
background: #ff004e;
color: white;
width: 40px;
height: 40px;
line-height: 40px;
text-align: center;
margin-top: 30px;
border-image: 50px;
}
.btn{
padding: 15px 50px;
border: 0;
margin-top: 40px;
background-color: #ff004e;
border: 0;
border-radius: 50px;
}
</style>
Now we need to add some basic styles to these elements. Firstly, we have added font-family
as Tahoma, then we have added a border around the image. After that, we have added some margin
and font-size
to heading. Then we have colored the subtitle and added some letter-spacing
. Then added some basic styles to font, and also we have added some basic style to the icons like color, positioning etc. Lastly, we have added some color to the button as well.
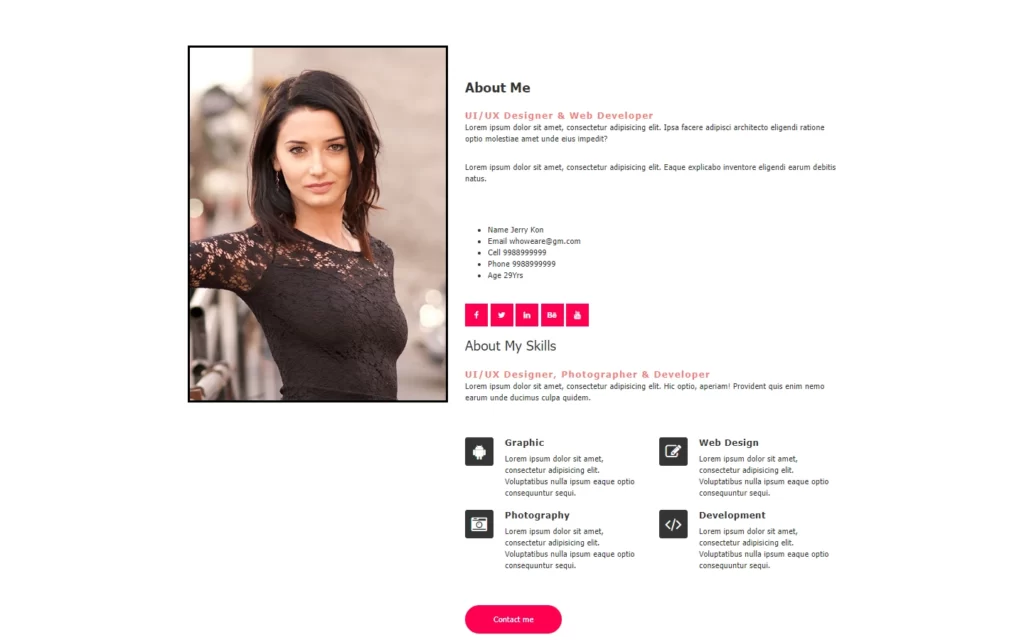
Adding Functionality to Slides
<script>
jQuery(document).ready(function(){
$('.hero .owl-carousel').owlCarousel({
items:1,
loop:true,
autoplay:true,
smartSpeed:500,
dots:false
});
});
</script>
Before jumping into the JavaScript, we need to hide our second section so that we can display these sections using JavaScript. For that, we have to add this <div class="owl-carousel owl-theme">
tag on the main section in which we have added both section. Using these classes, we will hide the content.
Now in the JavaScript part, we will use jQuery and Owl carousel for functionalities. With the help of jQuery(document).ready()
the function, we can fetch the status of the current window. If the window is ready, then we will call a function, in which we have here fetched the hero class and owl-carousel class. In this we have added items, and we have turned on loop, with autoplay on. Now we have added a duration for each section of 5 seconds.
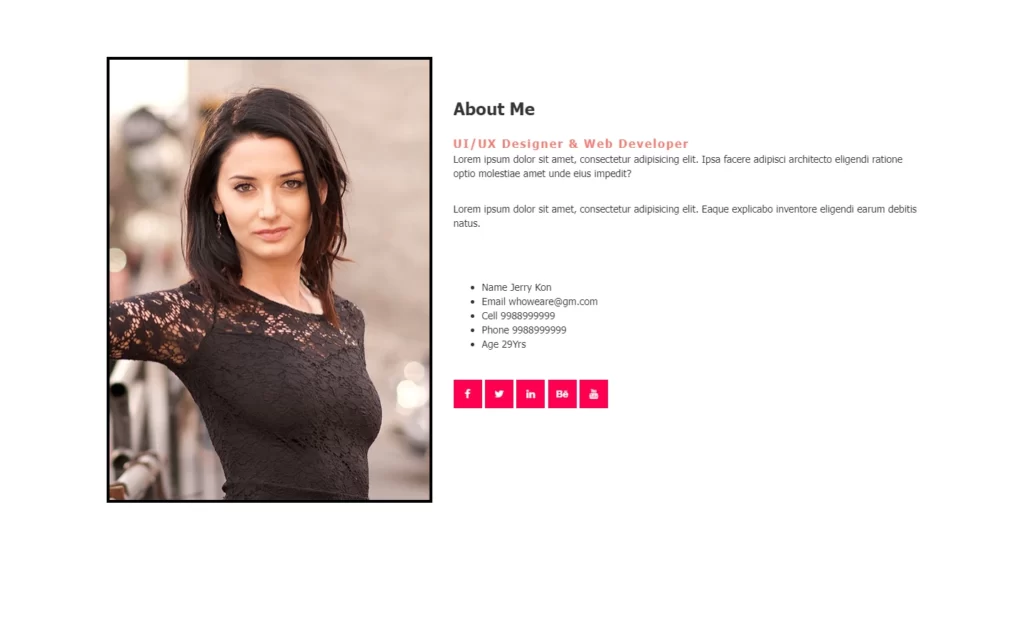
Full Source Code of Full Screen Image Slider in JavaScript with owl carousel
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>two way slider with owl</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<link rel="stylesheet" href="owl.carousel.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script src="owl.carousel.min.js"></script>
<style>
body{
font-family: tahoma;
}
.hero-img img{
border: 4px solid black;
}
.hero .content h3{
font-size: 800;
margin-bottom: 5px;
}
.hero .content .sub-title{
color: rgba(244,67,54,0.7);
letter-spacing: 2px;
font-size: 16px;
font-weight: bold;
margin-bottom: 20px;
}
.hero-content .info li{
width: 48%;
display: inline-block;
margin-bottom: 15px;
margin-left: -35px;
}
.hero .feature-box{
position: relative;
padding-left: 70px;
margin: 10px 0;
}
.hero .feature-box i{
font-size: 28px;
position: absolute;
left: 0;
width: 50px;
height: 50px;
text-align: center;
border-radius: 4px;
line-height: 50px;
color: white;
background: #343434;
}
.hero .feature-box h5{
color: #343434;
font-size: 16px;
font-weight: 600;
letter-spacing: 1px;
margin: 0 0 10px;
display: inline-block;
vertical-align: top;
width: 100%;
}
.social-icon .fa{
background: #ff004e;
color: white;
width: 40px;
height: 40px;
line-height: 40px;
text-align: center;
margin-top: 30px;
border-image: 50px;
}
.btn{
padding: 15px 50px;
border: 0;
margin-top: 40px;
background-color: #ff004e;
border: 0;
border-radius: 50px;
}
</style>
</head>
<body>
<br><br><br><br>
<section class="hero">
<div class="container">
<div class="row">
<div class="col-md-5">
<div class="hero-img">
<img src="huk4.jpg" alt="" class="img-responsive">
</div>
</div>
<br><br>
<div class="col-md-7">
<div class="owl-carousel owl-theme">
<div class="content">
<h3><b>About Me</b></h3>
<br>
<span class="sub-title">
UI/UX Designer & Web Developer
</span>
<p class="pb-10">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Ipsa facere adipisci architecto eligendi ratione optio molestiae amet unde eius impedit?
</p>
<br>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eaque explicabo inventore eligendi earum debitis natus.
</p>
<br><br><br>
<div class="info">
<ul>
<li>
<span class="title">Name</span>
<span class="value">Jerry Kon</span>
</li>
<li>
<span class="title">Email</span>
<span class="value">whoweare@gm.com</span>
</li>
<li>
<span class="title">Cell</span>
<span class="value">9988999999</span>
</li>
<li>
<span class="title">Phone</span>
<span class="value">9988999999</span>
</li>
<li>
<span class="title">Age</span>
<span class="value">29Yrs</span>
</li>
</ul>
<div class="social-icon">
<a href="">
<span><i class="fa fa-facebook"></i></span>
</a>
<a href="">
<span><i class="fa fa-twitter"></i></span>
</a>
<a href="">
<span><i class="fa fa-linkedin"></i></span>
</a>
<a href="">
<span><i class="fa fa-behance"></i></span>
</a>
<a href="">
<span><i class="fa fa-youtube"></i></span>
</a>
</div>
</div>
</div>
<div class="content">
<h3>About My Skills</h3>
<br>
<span class="sub-title">UI/UX Designer, Photographer & Developer</span>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Hic optio, aperiam! Provident quis enim nemo earum unde ducimus culpa quidem.
</p>
<br><br>
<div class="row">
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-android"></i>
<div class="feature-content">
<h5>Graphic</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-edit"></i>
<div class="feature-content">
<h5>Web Design</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-camera-retro"></i>
<div class="feature-content">
<h5>Photography</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
<div class="col-md-6">
<div class="feature-box">
<i class="fa fa-code"></i>
<div class="feature-content">
<h5>Development</h5>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus nulla ipsum eaque optio consequuntur sequi.
</p>
</div>
</div>
</div>
</div>
<div style="margin-top: 10px;"></div>
<div class="mt-10">
<a href="">
<span class="btn btn-danger">Contact me</span>
</a>
</div>
</div>
</div>
</div>
</div>
</div>
</section>
<script>
jQuery(document).ready(function(){
$('.hero .owl-carousel').owlCarousel({
items:1,
loop:true,
autoplay:true,
smartSpeed:500,
dots:false
});
});
</script>
</body>
</html>
Output
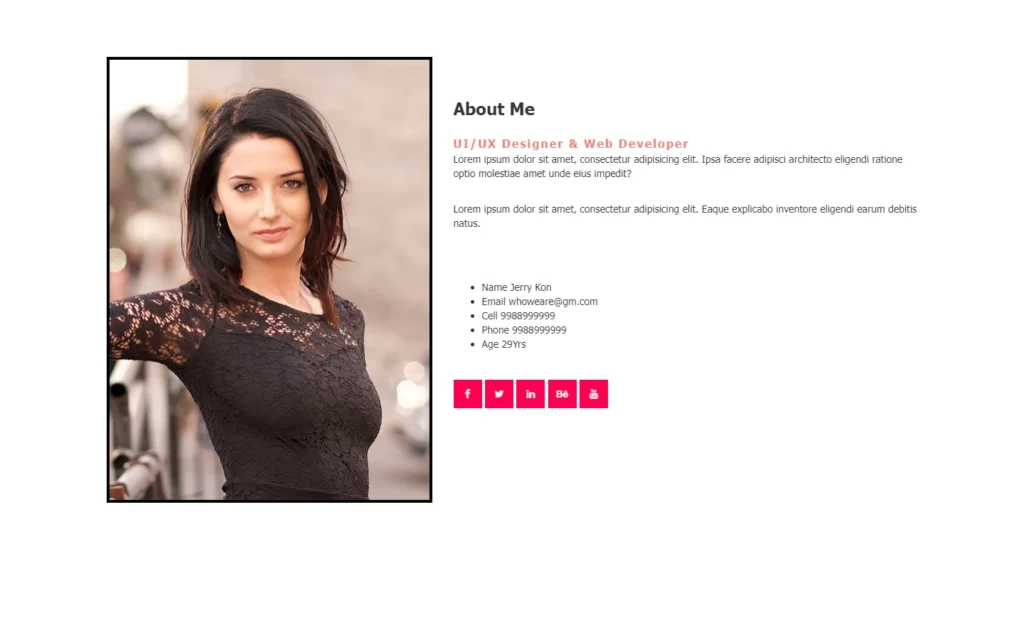
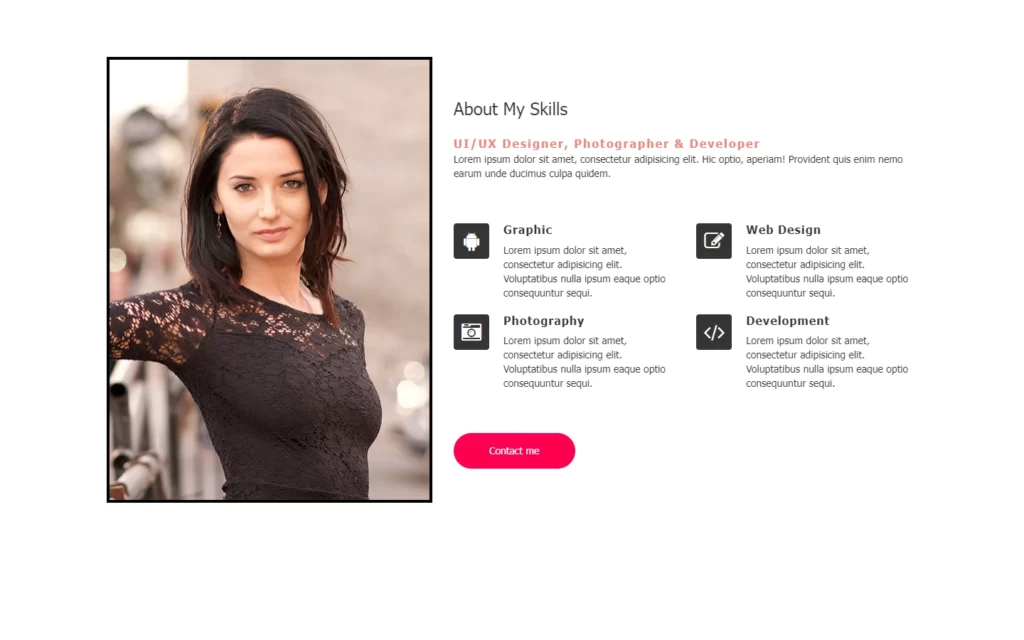