Hello, guys in this project we’re going to be building a Dictionary app using pure JavaScript. No angular, react no frameworks, not even jQuery. We’re going to be using HTML and CSS.in this project we use dictionary API.
In this project, we are going to learn new things like Fetch API, how to call API. And how to show data from the server-side in the browser. So let’s start it. Some screenshots are.
👉 In this input field, When we write an English word such as Apple and search. Then the meaning of Apple and how to speak it is its audio file.
👉 When we write the wrong word then they suggest some words.
👉So we open our editor and create index.html or style.css and index.js file. First, we design our app fastly. Our main focus will be on Javascript.
📂 index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dictonary App</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<nav>
<div class="container">
<h1>Dictionary</h1>
</div>
</nav>
<section class="input container">
<h2>Find any word exist in the world :)</h2>
<div class="form__wrap">
<div class="input__wrap">
<input type="text" placeholder="Type a word" id="input">
<button id="search">Search</button>
</div>
</div>
</section>
<section class="data container">
<span class="loading">Loading...</span>
<p class="def"></p>
<div class="audio"></div>
<div class="not__found"></div>
</section>
<script src="index.js"></script>
</body>
</html>
📂 style.css
@import url('https://fonts.googleapis.com/css2?family=Muli:wght@300;400&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
--webkit-font-smoothing: antialiased;
}
body {
font-family: 'Muli', sans-serif;
min-height: 100vh;
}
.container {
width: 1152px;
max-width: 90%;
margin: 0 auto;
}
nav {
background: #553C9A;
color: #fff;
padding: 1rem 0;
}
section.input {
padding-top: 100px;
}
h2 {
text-align: center;
font-size: 36px;
}
.form__wrap {
display: flex;
justify-content: center;
}
.input__wrap {
display: flex;
align-items: center;
border: 1px solid #ddd;
margin-top: 20px;
}
input {
border: none;
padding: 10px;
font-size: 16px;
min-width: 400px;
outline: none;
}
button{
background:#553C9A;
color: #fff;
height: 100%;
font-size: 16px;
padding: 0 20px;
cursor: pointer;
}
section.data{
max-width: 600px;
margin: 0 auto;
margin-top: 20px;
text-align: center;
}
.loading {
display: none;
}
section.data p.def {
font-size: 20px;
color: #2D3748;
line-height: 1.6;
}
.suggested {
background: #B794F4;
color: #fff;
padding: 2px 10px;
border-radius: 4px;
margin-right: 10px;
margin-top: 5px;
display: inline-block;
}
.audio {
margin-top: 20px;
}
👉 Now we write the code in index.js, We’ll need a few things first like input, search Button, API key, etc…
👉 First We need the API key. So we have to register on the Dictionary API website and get the API key.
let input = document.querySelector('#input');
let searchBtn = document.querySelector('#search');
let apiKey = 'YOUR_API_KEY';
let notFound = document.querySelector('.not__found');
let defBox = document.querySelector('.def');
let audioBox = document.querySelector('.audio');
let loading = document.querySelector('.loading');
👉 we create add event listner. when the user click on the search button.
searchBtn.addEventListener('click', function(e) {
})
👉 inside function we need to Get input data.
searchBtn.addEventListener('click', function(e) {
e.preventDefault();
// Get input data
let word = input.value;
})
👉 e.preventDefault() Meaning that when we click on the button. The page will not refresh. When our word is empty then we return something. Otherwise, we call the API.
searchBtn.addEventListener('click', function(e) {
e.preventDefault();
// Get input data
let word = input.value;
if (word === '') {
alert('Word is required');
return;
}
getData(word);
})
👉 inside getData function. The first thing we call API. We will use fetch here. fetch will return response and we store in the response variable. so we are using async-await .here response variable returns JSON data so we extract the data using the JSON method.
async function getData(word) {
// Ajax call
const response = await fetch(`https://www.dictionaryapi.com/api/v3/references/learners/json/${word}?key=${apiKey}`);
const data = await response.json();
console.log(data);
}
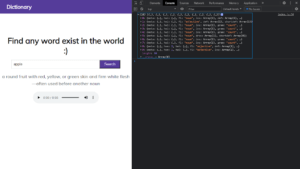
👉 here get the 10 results. but the first result is accurate. inside a first result, we need to short definition.
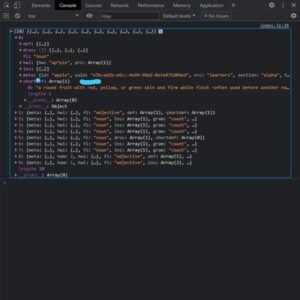
👉 if data is empty then we return a string not found. if data is present and the user enters the wrong word .first we check the data type. A data is a string then means there are suggestions.
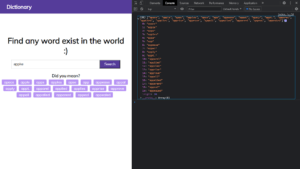
async function getData(word) {
loading.style.display = 'block';
// Ajax call
const response = await fetch(`https://www.dictionaryapi.com/api/v3/references/learners/json/${word}?key=${apiKey}`);
const data = await response.json();
console.log(data);
// if empty result
if (!data.length) {
loading.style.display = 'none';
notFound.innerText = ' No result found';
return;
}
// If result is suggetions
if (typeof data[0] === 'string') {
loading.style.display = 'none';
let heading = document.createElement('h3');
heading.innerText = 'Did you mean?';
notFound.appendChild(heading);
data.forEach((element) => {
let suggetion = document.createElement('span');
suggetion.classList.add('suggested');
suggetion.innerText = element;
notFound.appendChild(suggetion);
});
return;
}
// Result found
loading.style.display = 'none';
let defination = data[0].shortdef[0];
defBox.innerText = defination;
}
👉 if the result found we show the short definition.
async function getData(word) {
loading.style.display = 'block';
// Ajax call
const response = await fetch(`https://www.dictionaryapi.com/api/v3/references/learners/json/${word}?key=${apiKey}`);
const data = await response.json();
console.log(data);
// if empty result
if (!data.length) {
loading.style.display = 'none';
notFound.innerText = ' No result found';
return;
}
// If result is suggetions
if (typeof data[0] === 'string') {
loading.style.display = 'none';
let heading = document.createElement('h3');
heading.innerText = 'Did you mean?';
notFound.appendChild(heading);
data.forEach((element) => {
let suggetion = document.createElement('span');
suggetion.classList.add('suggested');
suggetion.innerText = element;
notFound.appendChild(suggetion);
});
return;
}
// Result found
loading.style.display = 'none';
let defination = data[0].shortdef[0];
defBox.innerText = defination;
}
👉 Now let’s work on getting audio files
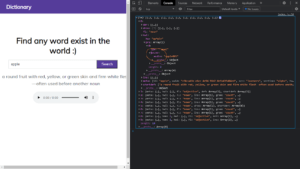
async function getData(word) {
loading.style.display = 'block';
// Ajax call
const response = await fetch(`https://www.dictionaryapi.com/api/v3/references/learners/json/${word}?key=${apiKey}`);
const data = await response.json();
console.log(data);
// if empty result
if (!data.length) {
loading.style.display = 'none';
notFound.innerText = ' No result found';
return;
}
// If result is suggetions
if (typeof data[0] === 'string') {
loading.style.display = 'none';
let heading = document.createElement('h3');
heading.innerText = 'Did you mean?';
notFound.appendChild(heading);
data.forEach((element) => {
let suggetion = document.createElement('span');
suggetion.classList.add('suggested');
suggetion.innerText = element;
notFound.appendChild(suggetion);
});
return;
}
// Result found
loading.style.display = 'none';
let defination = data[0].shortdef[0];
defBox.innerText = defination;
// Sound
const soundName = data[0].hwi.prs[0].sound.audio;
if (soundName) {
renderSound(soundName);
}
console.log(data);
}
function renderSound(soundName) {
// https://media.merriam-webster.com/soundc11
let subfolder = soundName.charAt(0);
let soundSrc = `https://media.merriam-webster.com/soundc11/${subfolder}/${soundName}.wav?key=${apiKey}`;
let aud = document.createElement('audio');
aud.src = soundSrc;
aud.controls = true;
audioBox.appendChild(aud);