In this article, we will make animated nike shoes landing page with only HTML, CSS & JavaScript. This page will contain a navigation bar, slides for shoes, add to cart option and some more basic things as a simple webpage has. Also, we will animate these slides and webpage.
Pre-requisites To Make Animated Nike Shoes Landing Page With Only HTML, CSS & JavaScript
- Good knowledge of HTML.
- Good knowledge of CSS and CSS animations.
- Good knowledge of JavaScript.
Building Common Layout And Styling
<body>
<div id="slider" class="slider">
<div class="row fullheight">
<div class="col-6 fullheight bg-red"></div>
<div class="col-6 fullheight bg-blue"></div>
</div>
</div>
</body>
:root {
--red: #ff3838;
--white: #ffffff;
--black: #000000;
--red-one: #CB356B;
--red-two: #BD3F32;
--green-one: #56ab2f;
--green-two: #94d049;
--black-one: #232526;
--black-two: #70767b;
--blue-one: #021B79;
--blue-two: #0575E6;
--silver: #bdc3c7;
}
@import url('https://fonts.googleapis.com/css2?family=Lato:wght@400;700;900&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Lato', sans-serif;
}
.bg-red {
background-color: var(--red);
}
.bg-white {
background-color: var(--white);
}
.bg-black {
background-color: var(--black);
}
.bg-blue {
background-color: var(--blue-one);
}
.bg-green {
background-color: var(--green-one);
}
body, html {
margin: 0;
padding: 0;
height: 100vh;
overflow: hidden;
}
.row {
display: flex;
flex-wrap: wrap;
flex-direction: row;
}
.row:after, .row:before {
box-sizing: border-box;
}
.col-6 {
width: 50%;
position: relative;
}
.slider {
overflow: hidden;
height: 500%;
}
.fullheight {
height: 100vh;
}
First of all In HTML, we have just partitioned our layout into 2 different parts. And for styling, we have assigned colors into the classes, if we use these classes then that class will be colored. Also, we have adjusted our content area with some basic CSS.
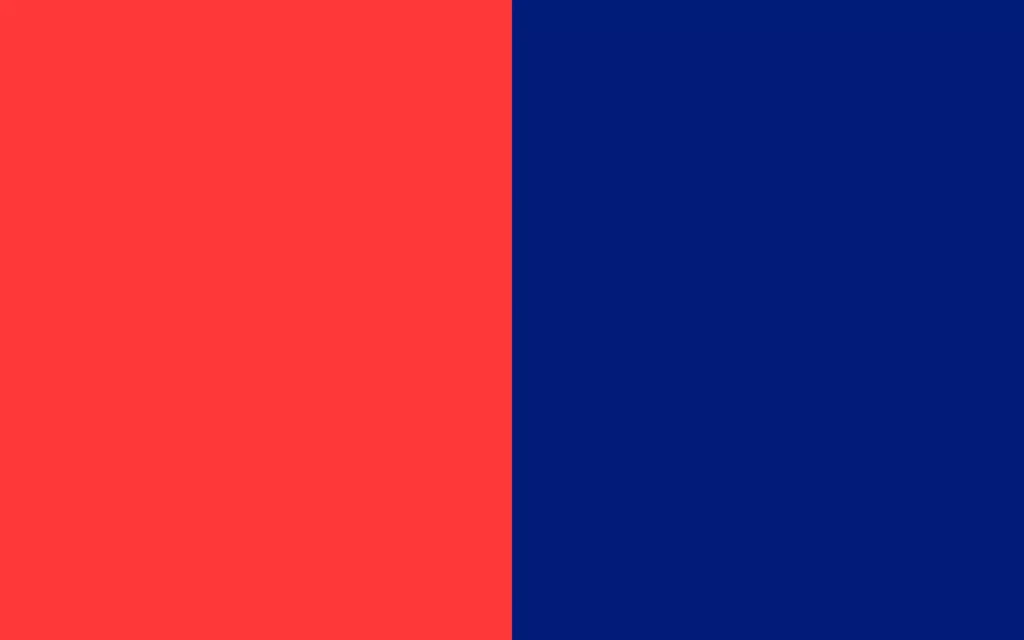
Adding Product Image
<div class="col-6 fullheight bg-blue">
<div class="product-img">
<div class="img-wrapper">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG.png" alt="placeholder+image">
</div>
</div>
</div>
.product-img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100%;
}
.img-wrapper img {
height: auto;
width: 80%;
transform: rotate(-35deg);
}
Now let’s put a product image, So we have simply fetched our product image from assets folder, in this folder we have all product images. Then after in CSS part, we have adjusted image position, and we have rotated it to 35%, so image looks better now.
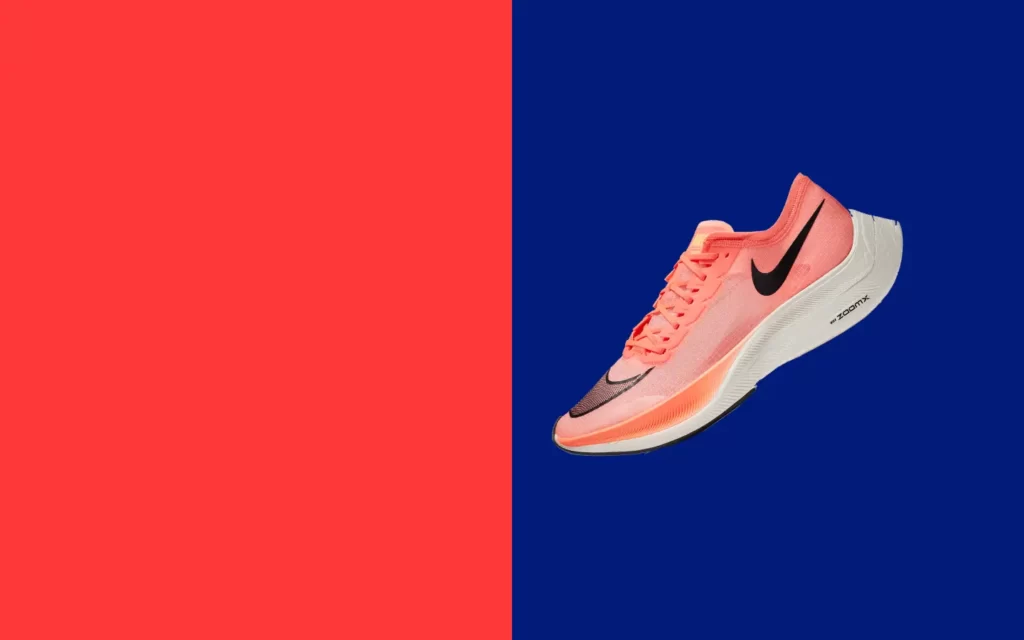
Adding More Product Images
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG-1.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG (1).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG (3).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG (4).jpg" alt="placeholder+image">
</div>
</div>
.more-images {
position: absolute;
right: 90px;
top: 50%;
}
.more-images-item {
height: fit-content;
border-radius: 15px;
overflow: hidden;
margin: 5px 0;
}
.more-images-item img {
height: auto;
width: 80px;
border-radius: 15px;
transition: 1s;
}
.more-images-item:hover img {
transform: scale(1.5);
}
.more-images-item:hover {
cursor: pointer;
}
Now, we need to add some more images of our product, like all views of our product. For that, we have just added those image in HTML part. In CSS part, we have put those image in small-image area, and we added some animation(Zoom-in effect) on these images, also we have given some transition, so animation would be smooth. To add zoom-in effect, we have just added scale to 1.5.
Adding Product Information
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike ZoomX Vaporfly NEXT%
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
.product-info {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100%;
padding: 0 10%;
color: var(--silver);
}
.info-wrapper {
margin: auto;
position: relative;
text-align: right;
}
.product-name h2 {
font-weight: 900;
font-size: xxx-large;
color: var(--black);
}
.product-price {
font-weight: 900;
font-size: xx-large;
color: var(--black);
}
.product-price span {
font-size: large;
color: var(--black-two);
}
.product-size {
margin: 20px 0;
}
.size-wrapper {
display: flex;
flex-flow: row-reverse;
padding: 5px;
}
.size-wrapper div {
font-weight: 900;
margin: 5px;
width: 50px;
height: 50px;
border-radius: 50%;
padding: 13px;
border: 2px solid var(--silver);
color: var(--silver);
}
.size-wrapper div.active {
border: 2px solid var(--black);
color: var(--black);
}
.size-wrapper div:hover {
border: 2px solid var(--black);
color: var(--black);
cursor: pointer;
}
.color-wrapper {
display: flex;
flex-flow: row-reverse;
padding: 5px;
}
.color-wrapper > div {
margin: 5px;
width: 50px;
height: 50px;
border-radius: 50%;
padding: 3px;
border: 2px solid var(--silver);
background-color: var(--silver);
}
.color-wrapper > div.active {
border: 2px solid var(--black);
}
.color-wrapper > div:hover {
cursor: pointer;
border: 2px solid var(--black);
}
.color-wrapper > div >div {
width: 40px;
height: 40px;
border-radius: 50%;
}
.product-description {
margin: 60px 0;
text-align: justify;
font-weight: 600;
}
.button > button {
font-weight: 900;
font-size: x-large;
padding: 15px 60px;
border-radius: 30px;
border: 2px solid var(--black);
background-color: var(--white);
color: var(--black);
transition: .5s;
}
.button > button:hover {
cursor: pointer;
background-color: var(--black);
color: var(--white);
}
After that, we need to add product information like name, size, price, color etc. Before adding the product information, we will remove background color which we have gave in HTML at partition time. Then after, we just added product name, price, color, size, add to cart button and description of the product. Now in CSS part, we have applied basic CSS designing to create modern information. Also, we have animated these effects little bit smoother with transition property.
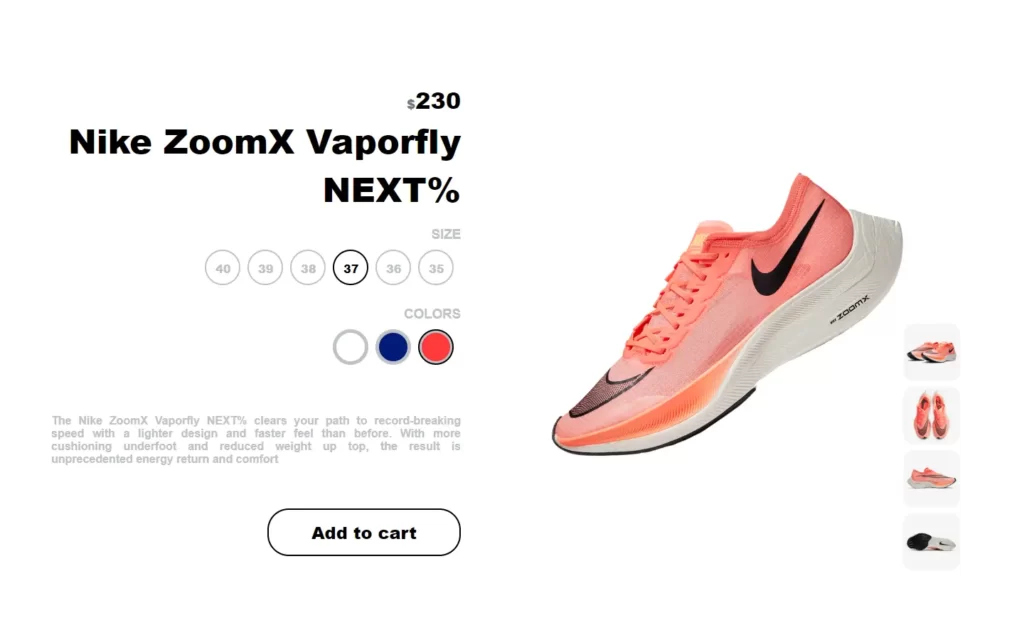
Making Slider For Multiple Products
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike Zoom Fly 3
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #6b6d68, #629f2a)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH.png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH (1).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH (2).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH (3).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
<!-- END SLIDE 2 -->
<!-- SLIDE 3 -->
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike Air Max Alpha TR 3
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #cdcbd3, #171617)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7.png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7 (1).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7 (2).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7 (3).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
<!-- END SLIDE 3 -->
<!-- SLIDE 4 -->
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike Air Zoom SuperRep
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #32519a, #4967af)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (1).png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (4).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (3).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (2).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
let slideIndex = 3;
let slider = document.getElementById('slider')
let slides = slider.getElementsByClassName('slide')
slider.style.marginTop = '-' + slideIndex + '00vh'
Now we will added some more other products, but before that, we need to add some designing variation around the product for that we just have added color same as product color and we have provided some border radius at top- left so it will for somewhat circular form. Then after, In HTML part, we have just copied above code for the 3 more times and we have just changed it color and product images.
In JS part, we are just getting the slider and slide. Then after we have styled our slider class with marginTop, with this we can get our image on our screen as per index number given. We will handle this thing with sliders menu.
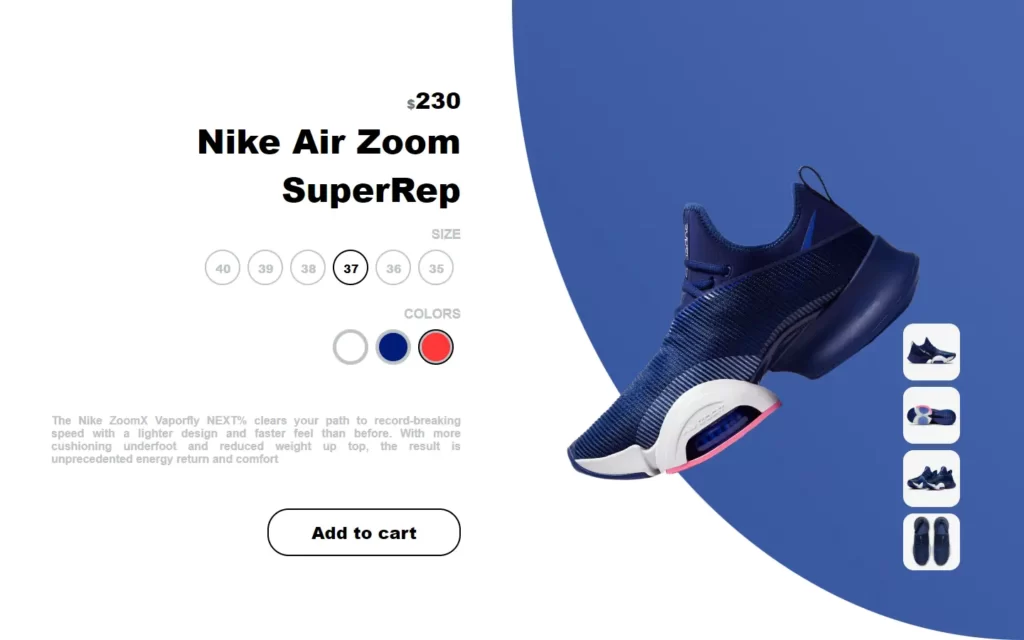
Adding Slide Control
<div id="slide-control" class="slide-control">
<div class="slide-control-item">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG.png" alt="placeholder+image">
</div>
<div class="slide-control-item">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH.png" alt="placeholder+image">
</div>
<div class="slide-control-item">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7.png" alt="placeholder+image">
</div>
<div class="slide-control-item">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (1).png" alt="placeholder+image">
</div>
</div>
.slide-control {
display: flex;
padding: 5px;
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
}
.slide-control div {
height: 50px;
width: 50px;
margin: 10px;
transition: .5s;
}
.slide-control div:hover {
cursor: pointer;
transform: translateY(-30px);
border-bottom: 2px solid var(--black);
}
.slide-control div img {
height: auto;
width: 100%;
filter: grayscale(100%);
transform: rotate(-45deg);
}
.slide-control div:hover img {
filter: unset;
}
.slide-control div.active img {
filter: unset;
}
.slide-control div.active {
border-bottom: 2px solid var(--black);
}
let slideControl = document.getElementById('slide-control')
let slideControlItems = slideControl.getElementsByClassName('slide-control-item')
slider.style.marginTop = '-' + slideIndex + '00vh'
setTimeout(() => {
slideControlItems[slideIndex].classList.add('active')
slides[slideIndex].classList.add('active')
}, 500)
chooseProduct = (index) => {
if (index === slideIndex) return
slideIndex = index
let currSlideControl = slideControl.querySelector('.slide-control-item.active')
currSlideControl.classList.remove('active')
let currSlide = slider.querySelector('.slide.active')
currSlide.classList.remove('active')
setTimeout(() => {
slider.style.marginTop = '-' + slideIndex + '00vh'
slideControlItems[slideIndex].classList.add('active')
slides[slideIndex].classList.add('active')
}, 1500)
}
Array.from(slideControlItems).forEach((el, index) => {
el.onclick = function() {
chooseProduct(index)
}
})
Now we need to add all product into slider so for that we have added all product images in the HTML, and in CSS we have just made images too small, and also we have done some animation on hover and on active events. Again, we have rotated all the images by 45%, so it looks somewhat similar to the main image. Also, we have used filter
property to unset, so this means we don’t want any kind of color filter or blur something.
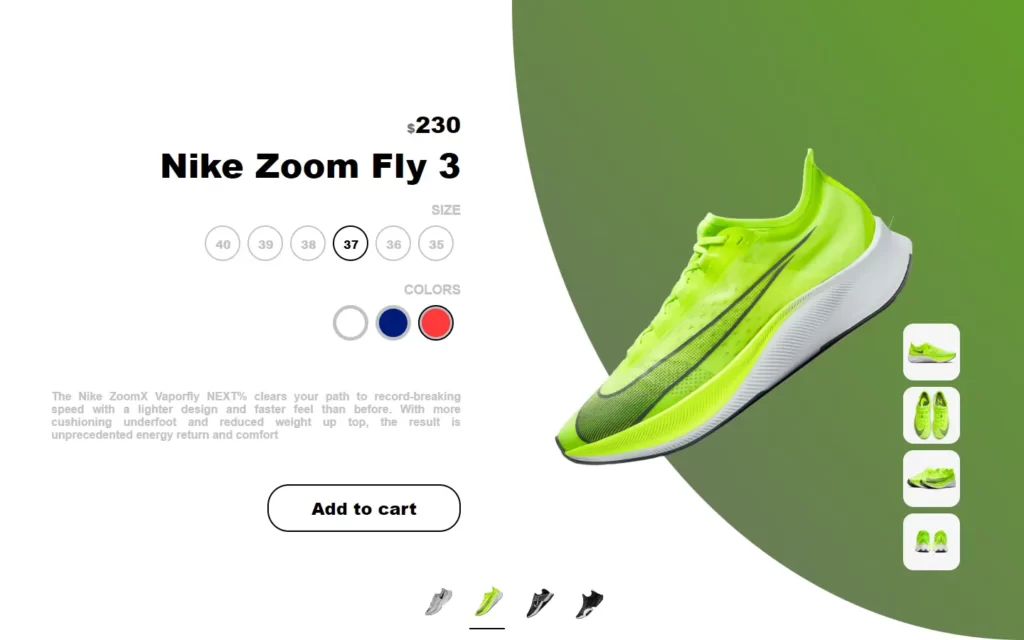
Adding Animations To Products Images and Information
We just added some delay on each element for smoother animations:
.right-to-left {
transition: 1s;
transform: translateX(100%);
}
.active .right-to-left {
transform: translateX(0);
}
.bottom-up {
transition: 1s;
transform: translateY(100vh);
}
.active .bottom-up {
transform: translateY(0);
}
.left-to-right {
transition: 1s;
transform: translateX(-150%);
}
.active .left-to-right {
transition: 1s;
transform: translateX(0);
}
.more-images-item:nth-child(1) {
transition-delay: .2s;
}
.more-images-item:nth-child(2) {
transition-delay: .4s;
}
.more-images-item:nth-child(3) {
transition-delay: .6s;
}
.more-images-item:nth-child(4) {
transition-delay: .8s;
}
.info-wrapper > div:nth-child(1) {
transition-delay: .2s;
}
.info-wrapper > div:nth-child(2) {
transition-delay: .4s;
}
.info-wrapper > div:nth-child(3) {
transition-delay: .6s;
}
.info-wrapper > div:nth-child(4) {
transition-delay: .8s;
}
.info-wrapper > div:nth-child(5) {
transition-delay: 1s;
}
.info-wrapper > div:nth-child(6) {
transition-delay: 1.2s;
}
Adding Model for Product view
<div id="modal" class="modal">
<span id="modal-close" class="close">×</span>
<img id="modal-content" class="modal-content">
<div class="more-images">
<div class="more-images-item">
<img class="img-preview">
</div>
<div class="more-images-item">
<img class="img-preview">
</div>
<div class="more-images-item">
<img class="img-preview">
</div>
<div class="more-images-item">
<img class="img-preview">
</div>
</div>
</div>
.modal {
display: none;
position: fixed;
z-index: 100;
padding-top: 100px;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgb(0,0,0);
background-color: rgba(0,0,0,0.9);
}
.modal-content {
margin: auto;
display: block;
width: 80%;
max-width: 700px;
}
.modal-content {
animation-name: zoom;
animation-duration: .6s;
}
@keyframes zoom {
from {
transform: scale(0);
} to {
transform: scale(1);
}
}
.close {
position: absolute;
top: 15px;
right: 35px;
color: var(--white);
font-size: 40px;
font-weight: 900;
transition: .3s;
}
.close:hover, .close:focus {
color: var(--silver);
text-decoration: none;
cursor: pointer;
}
let modal = document.getElementById('modal')
let closeBtn = document.getElementById('modal-close')
closeBtn.onclick = () => {
modal.style.display = 'none'
}
let moreImages = document.getElementsByClassName('more-images-item')
let previewImages = document.getElementsByClassName('img-preview')
Array.from(moreImages).forEach((el) => {
el.onclick = () => {
let imgItems = el.parentNode.getElementsByTagName('img')
Array.from(imgItems).forEach((item, index) => {
previewImages[index].src = item.src
})
let img = el.querySelector('img')
modal.style.display = 'block'
let modalContent = modal.querySelector('.modal-content')
modalContent.src = img.src
let temp = modalContent.cloneNode(true)
modalContent.parentNode.replaceChild(temp, modalContent)
}
})
Now we will add model for preview images, for that, we have added images again as multiple images for the product. Now, in CSS part, we need our these images to zoom in when we click on the images. We have added z-index
to 100, so zoomed image will cover whole webpage, also we have used black background color with 0.9 opacity so rest of the webpage content cover behind the color. It will be previewing the image like as we do in Amazon or Flipkart.
Then after in JavaScript part, we will just add onClick
event listener on each of the images, and we will add the main image to preview with the help of modalContent.parentNode.replaceChild(temp, modalContent)
code.
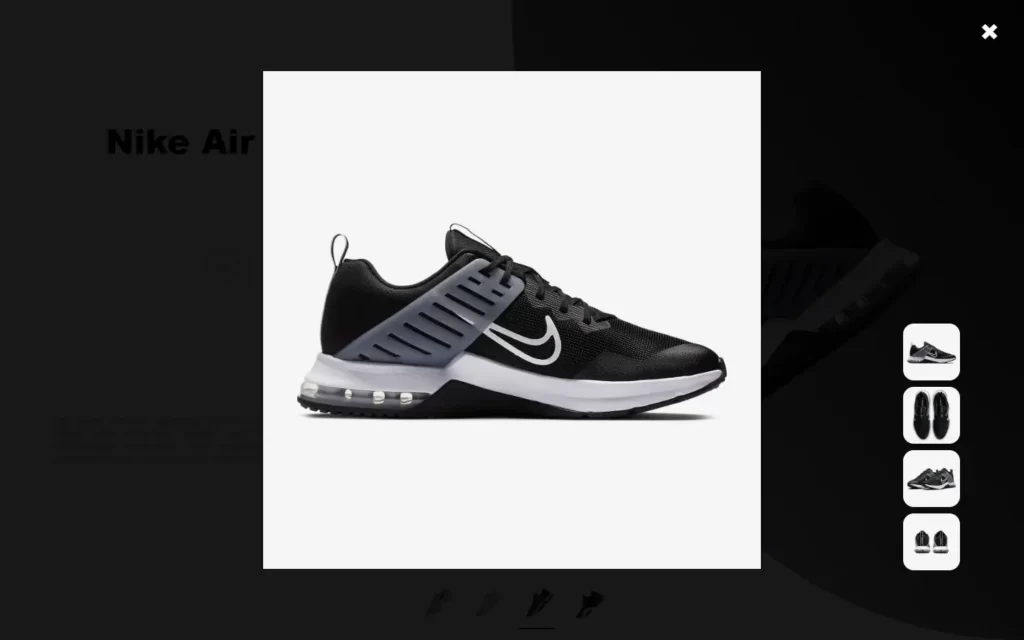
Adding Rest of The Elements
<div class="navbar">
<a href="#" class="logo">
Logo
</a>
<div class="navbar-right menu">
<a href="#" class="active">New releases</a>
<a href="#">Men</a>
<a href="#">Women</a>
<a href="#">Kids</a>
<a href="#">About</a>
<a href="#">Contacts</a>
</div>
<div class="navbar-right">
<a href="#" class="cart">
<i class="bx bx-cart-alt"></i>
<span class="badge">2</span>
</a>
</div>
</div>
.navbar {
background-color: transparent;
padding: 10px 90px;
position: fixed;
top: 0;
left: 0;
right: 0;
z-index: 99;
display: flex;
}
.navbar a {
float: left;
color: var(--white);
text-decoration: none;
font-size: 14px;
font-weight: 900;
padding:12px;
text-transform: uppercase;
}
.navbar .logo {
font-size: 30px;
flex: 1;
color: var(--black);
}
.navbar-right a:hover {
color: var(--black);
border-bottom: 2px solid var(--black);
}
.navbar-right a.active {
color: var(--black);
border-bottom: 2px solid var(--black);
}
.navbar-right a {
transition: .5s;
}
.cart {
position: relative;
}
.cart i {
font-size: x-large;
}
.badge {
position: absolute;
top: 0;
right: 1px;
font-size: 12px;
background-color: var(--red);
width: 20px;
height: 20px;
padding: 2px;
text-align: center;
justify-content: center;
border-radius: 50%;
}
Okay, we have done all the work related to our project, now we need to give last finishing touch here. We will add a navbar, logo and cart which don’t do anything right now, but you can do customization form JavaScript to add functionalities. Basically, we are not adding any functionalities with these elements, we will just add them as showcase.
So here, we have added a logo which have no linkage to any page, we have navbar here with some random categories, and finally we have cart icon. Now in css, we have just did some adjustment and styling to set them on there location and also we gave some animation to them for smoother look.
Full Source Code of Make Animated Nike Shoes Landing Page With Only HTML, CSS & JavaScript
index.html
<!DOCTYPE html>
<html>
<head>
<title>NIKE PRODUCTS PAGE</title>
<meta charset="utf-8">
<link href='https://unpkg.com/boxicons@2.0.7/css/boxicons.min.css' rel='stylesheet'>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<!-- TOP NAV -->
<div class="navbar">
<a href="#" class="logo">
Logo
</a>
<div class="navbar-right menu">
<a href="#" class="active">New releases</a>
<a href="#">Men</a>
<a href="#">Women</a>
<a href="#">Kids</a>
<a href="#">About</a>
<a href="#">Contacts</a>
</div>
<div class="navbar-right">
<a href="#" class="cart">
<i class="bx bx-cart-alt"></i>
<span class="badge">2</span>
</a>
</div>
</div>
<!-- END TOP NAV -->
<!-- MAIN -->
<div id="slider" class="slider">
<!-- SLIDE 1 -->
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike ZoomX Vaporfly NEXT%
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #e19e95, #fd835c)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG.png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG-1.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG (1).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG (3).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG (4).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
<!-- END SLIDE 1 -->
<!-- SLIDE 2 -->
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike Zoom Fly 3
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #6b6d68, #629f2a)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH.png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH (1).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH (2).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH (3).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
<!-- END SLIDE 2 -->
<!-- SLIDE 3 -->
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike Air Max Alpha TR 3
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #cdcbd3, #171617)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7.png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7 (1).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7 (2).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7 (3).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
<!-- END SLIDE 3 -->
<!-- SLIDE 4 -->
<div class="row fullheight slide">
<div class="col-6 fullheight">
<!-- PRODUCT INFO -->
<div class="product-info">
<div class="info-wrapper">
<div class="product-price left-to-right">
<span>$</span>230
</div>
<div class="product-name left-to-right">
<h2>
Nike Air Zoom SuperRep
</h2>
</div>
<div class="product-size left-to-right">
<h3>SIZE</h3>
<div class="size-wrapper">
<div>35</div>
<div>36</div>
<div class="active">37</div>
<div>38</div>
<div>39</div>
<div>40</div>
</div>
</div>
<div class="product-color left-to-right">
<h3>COLORS</h3>
<div class="color-wrapper">
<div class="active">
<div class="bg-red"></div>
</div>
<div class="">
<div class="bg-blue"></div>
</div>
<div class="">
<div class="bg-white"></div>
</div>
</div>
</div>
<div class="product-description left-to-right">
<p>
The Nike ZoomX Vaporfly NEXT% clears your path to record-breaking speed with a lighter design and faster feel than before. With more cushioning underfoot and reduced weight up top, the result is unprecedented energy return and comfort
</p>
</div>
<div class="button left-to-right">
<button>
Add to cart
</button>
</div>
</div>
</div>
<!-- END PRODUCT INFO -->
</div>
<div class="col-6 fullheight img-col" style="background-image: linear-gradient(to top right, #32519a, #4967af)">
<!-- PRODUCT IMAGE -->
<div class="product-img">
<div class="img-wrapper right-to-left">
<div class="bounce">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (1).png" alt="placeholder+image">
</div>
</div>
</div>
<!-- END PRODUCT IMAGE -->
<!-- PRODUCT MORE IMAGES -->
<div class="more-images">
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW.jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (4).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (3).jpg" alt="placeholder+image">
</div>
<div class="more-images-item bottom-up">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (2).jpg" alt="placeholder+image">
</div>
</div>
<!-- ENDPRODUCT MORE IMAGES -->
</div>
</div>
<!-- END SLIDE 4 -->
<!-- SLIDE CONTROL -->
<div id="slide-control" class="slide-control">
<div class="slide-control-item">
<img src="assets/zoomx-vaporfly-next-running-shoe-4Q5jfG.png" alt="placeholder+image">
</div>
<div class="slide-control-item">
<img src="assets/zoom-fly-3-mens-running-shoe-XhzpPH.png" alt="placeholder+image">
</div>
<div class="slide-control-item">
<img src="assets/air-max-alpha-tr-3-mens-training-shoe-0C1CV7.png" alt="placeholder+image">
</div>
<div class="slide-control-item">
<img src="assets/air-zoom-superrep-mens-hiit-class-shoe-ZWLnJW (1).png" alt="placeholder+image">
</div>
</div>
<!-- END SLIDE CONTROL -->
</div>
<!-- END MAIN -->
<!-- MODAL -->
<div id="modal" class="modal">
<span id="modal-close" class="close">×</span>
<img id="modal-content" class="modal-content">
<div class="more-images">
<div class="more-images-item">
<img class="img-preview">
</div>
<div class="more-images-item">
<img class="img-preview">
</div>
<div class="more-images-item">
<img class="img-preview">
</div>
<div class="more-images-item">
<img class="img-preview">
</div>
</div>
</div>
<!-- END MODAL -->
<script type="text/javascript" src="index.js"></script>
</body>
</html>
style.css
:root {
--red: #ff3838;
--white: #ffffff;
--black: #000000;
--red-one: #CB356B;
--red-two: #BD3F32;
--green-one: #56ab2f;
--green-two: #94d049;
--black-one: #232526;
--black-two: #70767b;
--blue-one: #021B79;
--blue-two: #0575E6;
--silver: #bdc3c7;
}
@import url('https://fonts.googleapis.com/css2?family=Lato:wght@400;700;900&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Lato', sans-serif;
}
.bg-red {
background-color: var(--red);
}
.bg-white {
background-color: var(--white);
}
.bg-black {
background-color: var(--black);
}
.bg-blue {
background-color: var(--blue-one);
}
.bg-green {
background-color: var(--green-one);
}
body, html {
margin: 0;
padding: 0;
height: 100vh;
overflow: hidden;
}
.row {
display: flex;
flex-wrap: wrap;
flex-direction: row;
}
.row:after, .row:before {
box-sizing: border-box;
}
.col-6 {
width: 50%;
position: relative;
}
.slider {
overflow: hidden;
height: 500%;
}
.fullheight {
height: 100vh;
}
.product-img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100%;
}
.img-wrapper img {
height: auto;
width: 80%;
transform: rotate(-35deg);
}
.more-images {
position: absolute;
right: 90px;
top: 50%;
}
.more-images-item {
height: fit-content;
border-radius: 15px;
overflow: hidden;
margin: 5px 0;
}
.more-images-item img {
height: auto;
width: 80px;
border-radius: 15px;
transition: 1s;
}
.more-images-item:hover img {
transform: scale(1.5);
}
.more-images-item:hover {
cursor: pointer;
}
.product-info {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100%;
padding: 0 10%;
color: var(--silver);
}
.info-wrapper {
margin: auto;
position: relative;
text-align: right;
}
.product-name h2 {
font-weight: 900;
font-size: xxx-large;
color: var(--black);
}
.product-price {
font-weight: 900;
font-size: xx-large;
color: var(--black);
}
.product-price span {
font-size: large;
color: var(--black-two);
}
.product-size {
margin: 20px 0;
}
.size-wrapper {
display: flex;
flex-flow: row-reverse;
padding: 5px;
}
.size-wrapper div {
font-weight: 900;
margin: 5px;
width: 50px;
height: 50px;
border-radius: 50%;
padding: 13px;
border: 2px solid var(--silver);
color: var(--silver);
}
.size-wrapper div.active {
border: 2px solid var(--black);
color: var(--black);
}
.size-wrapper div:hover {
border: 2px solid var(--black);
color: var(--black);
cursor: pointer;
}
.color-wrapper {
display: flex;
flex-flow: row-reverse;
padding: 5px;
}
.color-wrapper > div {
margin: 5px;
width: 50px;
height: 50px;
border-radius: 50%;
padding: 3px;
border: 2px solid var(--silver);
background-color: var(--silver);
}
.color-wrapper > div.active {
border: 2px solid var(--black);
}
.color-wrapper > div:hover {
cursor: pointer;
border: 2px solid var(--black);
}
.color-wrapper > div >div {
width: 40px;
height: 40px;
border-radius: 50%;
}
.product-description {
margin: 60px 0;
text-align: justify;
font-weight: 600;
}
.button > button {
font-weight: 900;
font-size: x-large;
padding: 15px 60px;
border-radius: 30px;
border: 2px solid var(--black);
background-color: var(--white);
color: var(--black);
transition: .5s;
}
.button > button:hover {
cursor: pointer;
background-color: var(--black);
color: var(--white);
}
.img-col {
border-bottom-left-radius: 100%;
}
.slide-control {
display: flex;
padding: 5px;
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
}
.slide-control div {
height: 50px;
width: 50px;
margin: 10px;
transition: .5s;
}
.slide-control div:hover {
cursor: pointer;
transform: translateY(-30px);
border-bottom: 2px solid var(--black);
}
.slide-control div img {
height: auto;
width: 100%;
filter: grayscale(100%);
transform: rotate(-45deg);
}
.slide-control div:hover img {
filter: unset;
}
.slide-control div.active img {
filter: unset;
}
.slide-control div.active {
border-bottom: 2px solid var(--black);
}
.modal {
display: none;
position: fixed;
z-index: 100;
padding-top: 100px;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgb(0,0,0);
background-color: rgba(0,0,0,0.9);
}
.modal-content {
margin: auto;
display: block;
width: 80%;
max-width: 700px;
}
.modal-content {
animation-name: zoom;
animation-duration: .6s;
}
@keyframes zoom {
from {
transform: scale(0);
} to {
transform: scale(1);
}
}
.close {
position: absolute;
top: 15px;
right: 35px;
color: var(--white);
font-size: 40px;
font-weight: 900;
transition: .3s;
}
.close:hover, .close:focus {
color: var(--silver);
text-decoration: none;
cursor: pointer;
}
.navbar {
background-color: transparent;
padding: 10px 90px;
position: fixed;
top: 0;
left: 0;
right: 0;
z-index: 99;
display: flex;
}
.navbar a {
float: left;
color: var(--white);
text-decoration: none;
font-size: 14px;
font-weight: 900;
padding:12px;
text-transform: uppercase;
}
.navbar .logo {
font-size: 30px;
flex: 1;
color: var(--black);
}
.navbar-right a:hover {
color: var(--black);
border-bottom: 2px solid var(--black);
}
.navbar-right a.active {
color: var(--black);
border-bottom: 2px solid var(--black);
}
.navbar-right a {
transition: .5s;
}
.cart {
position: relative;
}
.cart i {
font-size: x-large;
}
.badge {
position: absolute;
top: 0;
right: 1px;
font-size: 12px;
background-color: var(--red);
width: 20px;
height: 20px;
padding: 2px;
text-align: center;
justify-content: center;
border-radius: 50%;
}
/* ANIMATION */
.right-to-left {
transition: 1s;
transform: translateX(100%);
}
.active .right-to-left {
transform: translateX(0);
}
.bottom-up {
transition: 1s;
transform: translateY(100vh);
}
.active .bottom-up {
transform: translateY(0);
}
.left-to-right {
transition: 1s;
transform: translateX(-150%);
}
.active .left-to-right {
transition: 1s;
transform: translateX(0);
}
.more-images-item:nth-child(1) {
transition-delay: .2s;
}
.more-images-item:nth-child(2) {
transition-delay: .4s;
}
.more-images-item:nth-child(3) {
transition-delay: .6s;
}
.more-images-item:nth-child(4) {
transition-delay: .8s;
}
.info-wrapper > div:nth-child(1) {
transition-delay: .2s;
}
.info-wrapper > div:nth-child(2) {
transition-delay: .4s;
}
.info-wrapper > div:nth-child(3) {
transition-delay: .6s;
}
.info-wrapper > div:nth-child(4) {
transition-delay: .8s;
}
.info-wrapper > div:nth-child(5) {
transition-delay: 1s;
}
.info-wrapper > div:nth-child(6) {
transition-delay: 1.2s;
}
.bounce {
animation-name: bounce;
animation-timing-function: ease;
animation-iteration-count: infinite;
animation-duration: 3s;
}
@keyframes bounce {
0% {
transform: translateY(0);
} 50% {
transform: translateY(-50px);
} 100% {
transform: translateY(0);
}
}
index.js
let slideIndex = 0;
let slider = document.getElementById('slider')
let slides = slider.getElementsByClassName('slide')
let slideControl = document.getElementById('slide-control')
let slideControlItems = slideControl.getElementsByClassName('slide-control-item')
slider.style.marginTop = '-' + slideIndex + '00vh'
setTimeout(() => {
slideControlItems[slideIndex].classList.add('active')
slides[slideIndex].classList.add('active')
}, 500)
chooseProduct = (index) => {
if (index === slideIndex) return
slideIndex = index
let currSlideControl = slideControl.querySelector('.slide-control-item.active')
currSlideControl.classList.remove('active')
let currSlide = slider.querySelector('.slide.active')
currSlide.classList.remove('active')
setTimeout(() => {
slider.style.marginTop = '-' + slideIndex + '00vh'
slideControlItems[slideIndex].classList.add('active')
slides[slideIndex].classList.add('active')
}, 1500)
}
Array.from(slideControlItems).forEach((el, index) => {
el.onclick = function() {
chooseProduct(index)
}
})
let modal = document.getElementById('modal')
let closeBtn = document.getElementById('modal-close')
closeBtn.onclick = () => {
modal.style.display = 'none'
}
let moreImages = document.getElementsByClassName('more-images-item')
let previewImages = document.getElementsByClassName('img-preview')
Array.from(moreImages).forEach((el) => {
el.onclick = () => {
let imgItems = el.parentNode.getElementsByTagName('img')
Array.from(imgItems).forEach((item, index) => {
previewImages[index].src = item.src
})
let img = el.querySelector('img')
modal.style.display = 'block'
let modalContent = modal.querySelector('.modal-content')
modalContent.src = img.src
let temp = modalContent.cloneNode(true)
modalContent.parentNode.replaceChild(temp, modalContent)
}
})
Output
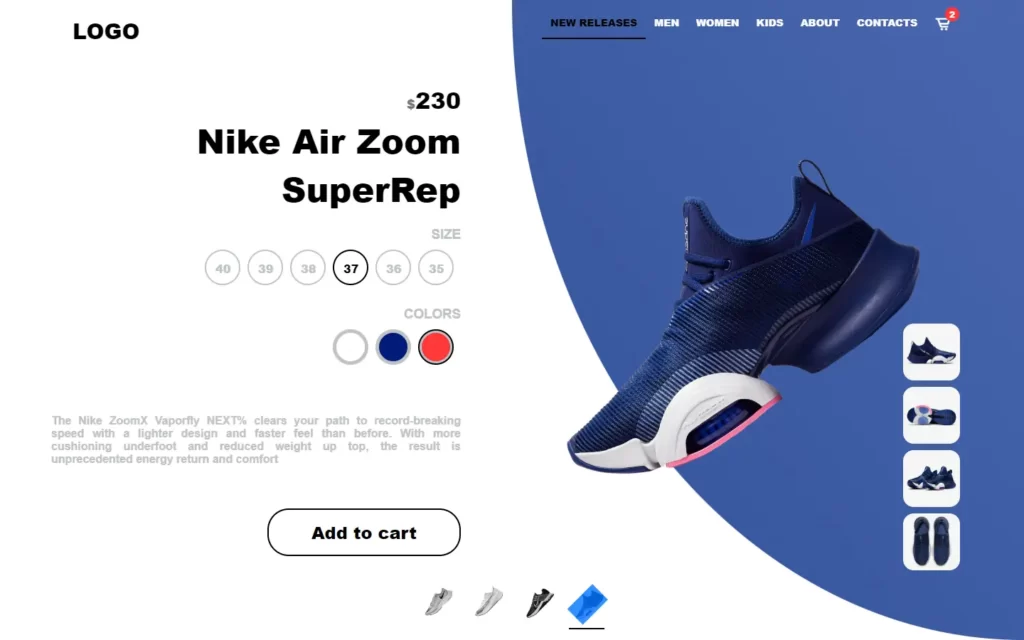