In this article, we are going to talk about how we can perform searching an array for a value. Searching an array is a common task. In the past, we have relied on indexOf() and lastIndexOf(). As of ES6, there are two new array methods: findIndex() and find(). This tutorial covers indexOf(), lastIndexOf(), findIndex() and find().
So as we know, we can do searching an array operation for a value using indexOf() and lastIndexOf() methods which returns index of given value. But it becomes hard, when we try to find a number of elements from a given array on basis of certain conditions, then it becomes necessary to loop or another array to do this kind of operation. But today, we will another ways to do this same operation.
Searching an Array For a Value: indexOf() and lastIndexOf()
Let’s see, first, how we can use indexOf() and lastIndexOf() methods. Here’s a basic example for this, in here we have defined an array with some value, and also we added a duplicate value. Now we can use indexOf() method to find value 38 and this method will return the very first index of this value. And we will use lastIndexOf() method to find the same value, but this method will return the very last index of the value.
>let arr = [1, 20, 38, 7, 27 ,38]
>undefined
>arr.indexOf(38)
>2
>arr.lastIndexOf(38)
>5
Searching an Array For a Value: findIndex()
In findIndex() method, we have to pass a function where we add some logic to get some filtered values from the array. In this function we have passed three parameters, element, index and new array. In element, the value of the array will be assigned every time, index will be the index number of that element and new array will target the given array, when we will call this function inside findIndex() method to apply on array.
Now in this function we have added a logic to get values until value is less than 30, and we are just displaying those elements. Here we will also get the index of last element.
let arr = [1, 27, 6, 30 , 56, 2, 30];
let over30 = function(ele, index, theArr){
console.log(ele + "-" + index + "-" + theArr);
return ele > 30;
}
console.log(arr.findIndex(over30));
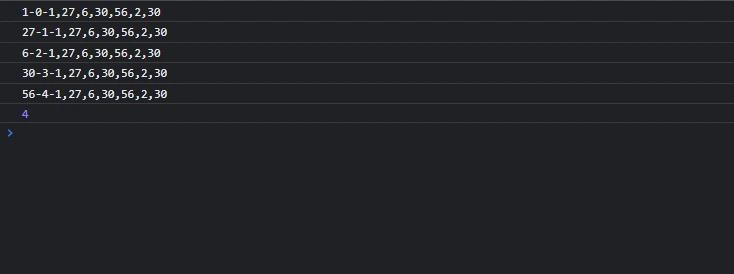
Searching an Array For a Value: find()
The find() method is most of similar to findIndex() method, which again takes a function as a parameter and this function contains similar three parameters. But here is the only difference between these two method is findIndex() returns index of last value inputted in the array, where find() method will return value of last element added to array.
let arr = [1, 27, 6, 30 , 56, 2, 30];
let over30 = function(ele, index, theArr){
console.log(ele + "-" + index + "-" + theArr);
return ele > 30;
}
console.log(arr.find(over30));
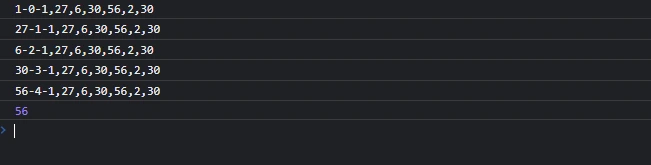