In this article, we are going to make random joke generator using JavaScript Fetch API, so in this we are having a joke here, and also we have the button whenever we will click this button we will generate a new joke for this we are going to see how we can fetch an API and get the data, and we can use it.
Pre-requisites To Make Random Joke Generator Using JavaScript Fetch API
- Basic knowledge of HTML.
- Good knowledge of CSS & CSS3.
- Good knowledge of JavaScript.
Adjusting Default Values
<body>
<section>
<h1 class="title">Random Dad Jokes Generator</h1>
<div class="container">
<div class="joke">
<p></p>
</div>
<button>Get a Dad Joke</button>
</div>
</section>
<script src ="main.js"></script>
</body>
So first of all we need to create the layout, so here let’s say that in the body we will have a section and in the section first of all let’s say that we will have div with a class of container so now and in the container we will have a div with a class of joke, and also we will have a button so which will basically generate the jokes, and let’s actually also link our style sheet so link CSS and also link the script tag here.
@import url("<https://fonts.googleapis.com/css2?family=Montserrat:wght@300;700&display=swap>");
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
html {
font-family: "Montserrat";
font-size: 10px;
}
body {
background-color: rgb(37, 38, 39);
color: white;
}
section {
min-height: 100vh;
width: 100%;
padding: 100px 0;
display: flex;
align-items: center;
justify-content: center;
}
In our style.css, we need the font, so we already selected the font we are going to use Montserrat and also 300 and 700 you can get the font from the Google fonts.com, after that lets get the basic style which is margin 0 and padding 0 and also say that box sizing will be the border box and after that lets come to the HTML and say that font size will be 10 pixels and also said that font family I want is the Montserrat.
Let’s come to the body and give the background color it will be a dark color after that let’s say that the color we want is the white, after that lets come to the section the min height will be 100vh and also say that width will be 100% and padding will be 100 pixels and 0 also let’s make a display flex and align item center and justify content also center.
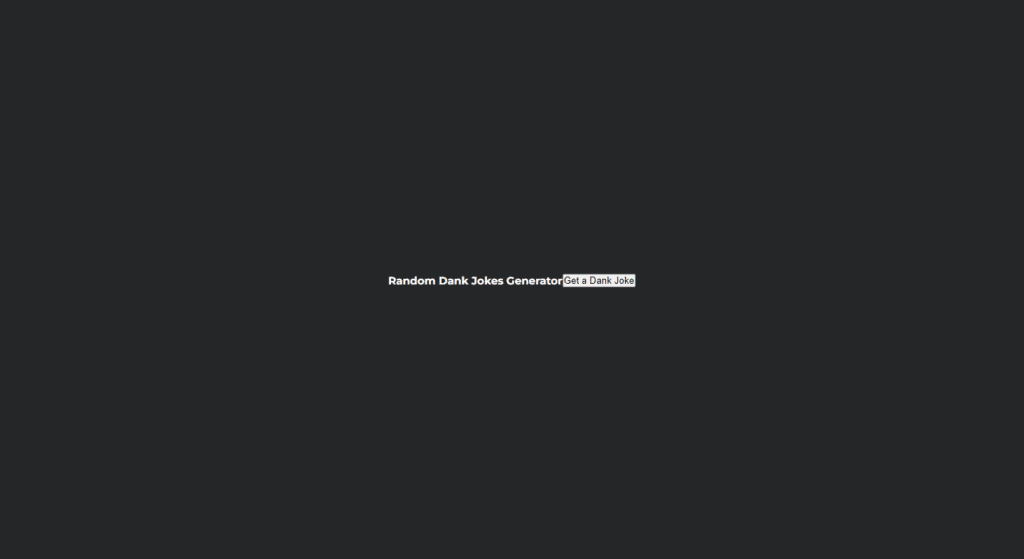
Customizing The Joke Container
.title {
position: absolute;
top: 50px;
font-size: 3rem;
font-weight: 700;
text-align: center;
}
.container {
width: 90%;
max-width: 500px;
margin: 0 auto;
background-color: rgb(51, 55, 59);
border-radius: 8px;
padding: 3rem;
display: flex;
flex-direction: column;
}
.container p {
font-size: 2rem;
font-weight: 300;
line-height: 3rem;
text-align: justify;
color: aqua;
}
After that lets come to the container, let’s say that width will be 90% and also say that max width will be something like 500 pixels now we need the margin 0 and auto, let’s have a background color like rgb (51, 55, 59) after that let’s have a border radius to 8 pixels also let’s have a padding of 3rem and display flex with flex direction to column.
Let’s style the paragraph element, let’s say that the font size will be something like 2 rem and font weight 300 and also say that line height is 3rem after that lets have a text alignment to justify with color aqua, so it will look good.
Now for title, let’s set position to absolute with top 50 pixels now let’s add font size 3rem and font weight to 700 also text alignment to center.
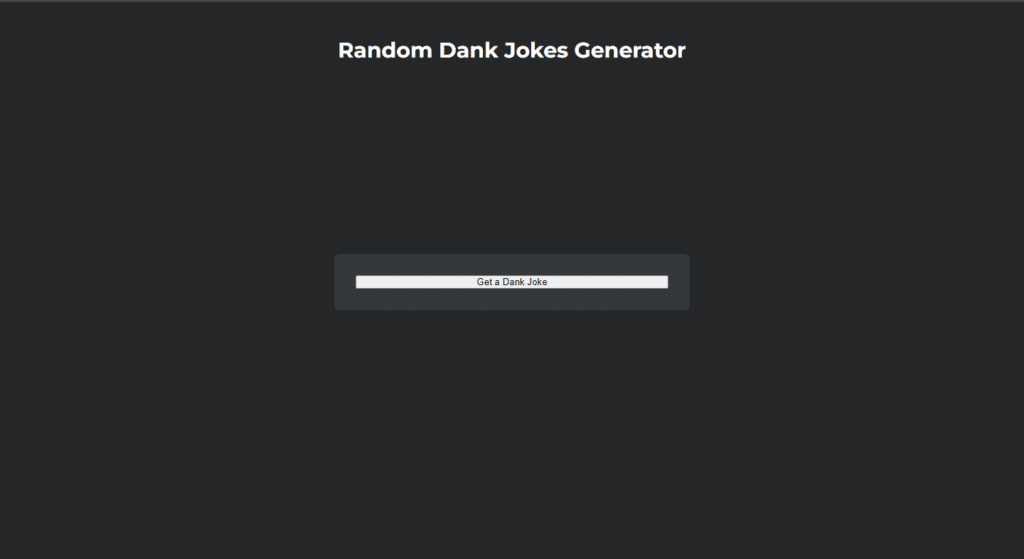
Customizing The Button
button {
margin-top: 40px;
width: fit-content;
padding: 2rem;
font-size: 2rem;
background-color: rgb(25, 28, 29);
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
align-self: flex-end;
}
Let’s extend the button here, so button will be emerging top will be something like 40px, and width will be fit content and also say that padding is 2rem now let’s increase the font size, so font size will be 2rem and also background color we want a dark color also let us make the white color for the text looking good and let’s have border none and also border radius the border area should be 4 pixels, and cursor to pointer now align-self will be the flex end.
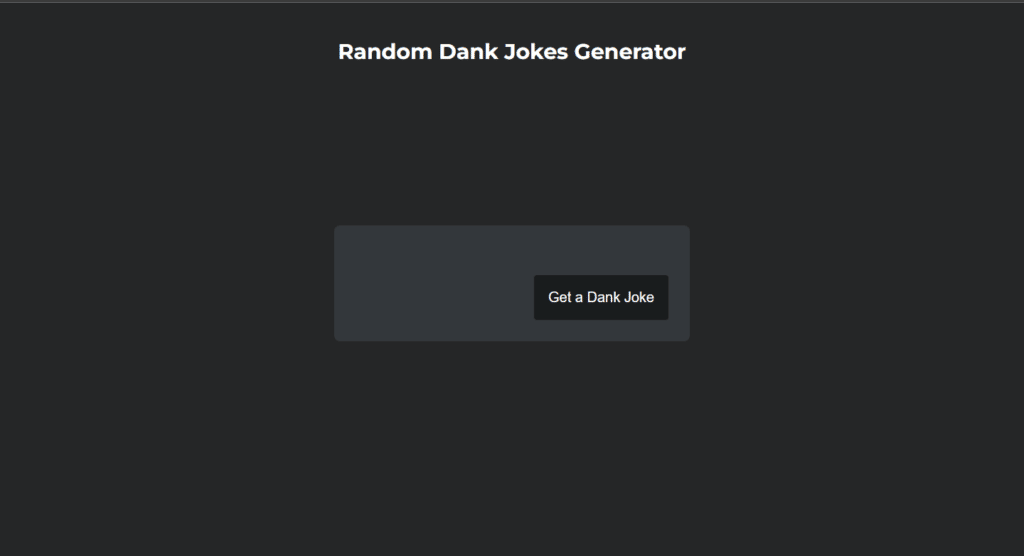
Adding Functionality To Button & API
const button = document.querySelector(".container button");
const jokeDiv = document.querySelector(".container .joke p");
document.addEventListener("DOMContentLoaded", getJock);
button.addEventListener("click", getJock);
async function getJock() {
const jokeData = await fetch("<https://icanhazdadjoke.com/>", {
headers: {
Accept: "application/json"
}
});
const jokeObj = await jokeData.json();
jokeDiv.innerHTML = jokeObj.joke;
console.log(jokeData);
}
Let’s fetch the API so in our main.js, we need to grab the button here so button to the document.queryselector(”.container button”) and also let’s say that constant joke div to document.queryselector(”.container .joke p”) now let’s add event listener with DOM content loaded with this if we load the project then getJock function will be called, after that we will add event listener on button so if we click on button then getJock function will be called again, and we’ll get new joke.
Now let’s define the function getJock, here let’s fetch API here we have used this https://icanhazdadjoke.com/ with header accept we will use here application/JSON format.
Now let’s const jokeObj we will fetch jokeData in JSON format, after that lets do innerHTML to jokeDiv.
Full Source Code Of Random Joke Generator Using JavaScript Fetch API
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dank Jokes</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<section>
<h1 class="title">Random Dank Jokes Generator</h1>
<div class="container">
<div class="joke">
<p></p>
</div>
<button>Get a Dank Joke</button>
</div>
</section>
<script src="main.js"></script>
</body>
</html>
style.css
@import url("<https://fonts.googleapis.com/css2?family=Montserrat:wght@300;700&display=swap>");
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
html {
font-family: "Montserrat";
font-size: 10px;
}
body {
background-color: rgb(37, 38, 39);
color: white;
}
section {
min-height: 100vh;
width: 100%;
padding: 100px 0;
display: flex;
align-items: center;
justify-content: center;
}
.title {
position: absolute;
top: 50px;
font-size: 3rem;
font-weight: 700;
text-align: center;
}
.container {
width: 90%;
max-width: 500px;
margin: 0 auto;
background-color: rgb(51, 55, 59);
border-radius: 8px;
padding: 3rem;
display: flex;
flex-direction: column;
}
.container p {
font-size: 2rem;
font-weight: 300;
line-height: 3rem;
text-align: justify;
color: aqua;
}
button {
margin-top: 40px;
width: fit-content;
padding: 2rem;
font-size: 2rem;
background-color: rgb(25, 28, 29);
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
align-self: flex-end;
}
main.js
const button = document.querySelector(".container button");
const jokeDiv = document.querySelector(".container .joke p");
document.addEventListener("DOMContentLoaded", getJock);
button.addEventListener("click", getJock);
async function getJock() {
const jokeData = await fetch("<https://icanhazdadjoke.com/>", {
headers: {
Accept: "application/json"
}
});
const jokeObj = await jokeData.json();
jokeDiv.innerHTML = jokeObj.joke;
console.log(jokeData);
}
Output
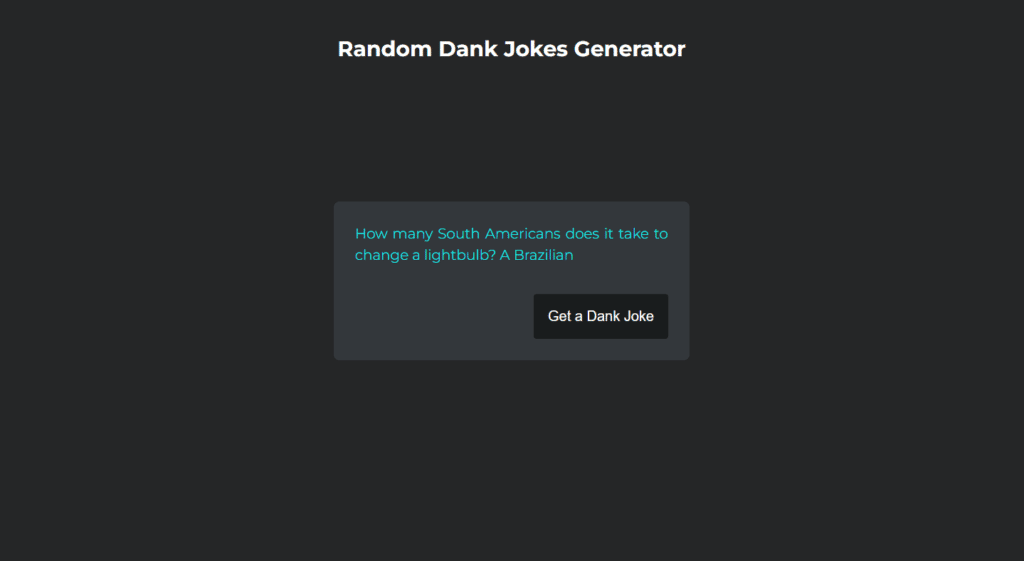