Looking forward to associating an exciting event, it helps to know how long you have to wait. In this project, In Countdown Calendar using python tkinter.you will use Python’s Tkinter module for straightforward build A program that counts the large day.
What happens In Countdown Calendar using Python Tkinter
Displays the incoming list when running the program until it tells you how many days there are until each. Run it again and the next day you will see One day subtracted from each “Until the days” figures. Fill in your dates Try it in the future and you will never miss it An important day – or homework deadline – too!
you can see the demo here
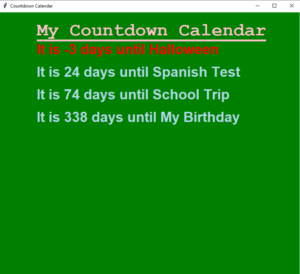
How Countdown Calendar works
The program learns about the important events by reading information from a text file—this is called “file input”. The text file contains the name and date of each event. The code calculates the number of days from today until each event using Python’s datetime module. It displays the results in a window created by Python’s Tkinter module.
Making and reading the text file
All the information for your Countdown Calendar must be stored in a text file.
Create a new file
Open a new IDLE file, then type in a few upcoming events that are important to you. Put each event on a separate line and type a comma between the event and its date. Make sure there is no space between the comma and the event date.
Halloween,4/11/20
Spanish Test,01/12/20
School Trip,20/01/21
My Birthday,11/10/21
Save it as a text file
Next, save the file as a text file. file save as “events.txt”. Now you’re ready to start coding the Python program.
Open a new Python file
Now you need to create a new file for the code. Save this as “countdown_calendar.py”
Set up the modules
This project needs two modules: Tkinter and datetime. Tkinter will be used to build a simple GUI, while datetime will make it easy to do calculations using dates. Import them by typing these two lines at the top of your new program.
from tkinter import Tk, Canvas
from datetime import date, datetime
Create the canvas
Now set the window that will display your important events And the number of days to each one. Put this code below . This creates a window containing one “Canvas” – a blank rectangle to which you can add text and graphics.
root = Tk()
c = Canvas(root, width=800, height=800, bg='black')
c.pack()
c.create_text(100, 50, anchor='w', fill='orange',
font='Arial 28 bold underline', text='My Countdown Calendar')
Read the text file
Next create a function to read and save all events from the text file. create new a function called get_events. Inside the function, an empty list that will keep events there file read.
from datetime import date, datetime
def get_events():
list_events = []
root = Tk()
Open the text file
This next bit of code will open the file called events.txt so the program can read it.
def get_events():
list_events = []
with open('events.txt') as file:
Start a loop
Now add a for loop to bring information from the text file into your program. The loop will be run for every line in the events.txt file.
def get_events():
list_events = []
with open('events.txt') as file:
for line in file:
Remove the invisible character
When typing details in a text file. Press enter/return key at the end of each line. This added an invisible The letter “newline” at the end of the whole line. So you can’t see this character. Python can Enter this line of code, which tells Python ignoring these invisible characters there reads a text file.
with open('events.txt') as file:
for line in file:
line = line.rstrip('n')
Store the event details
At this point, a dynamic line called the line holds the details for each event as a character unit, similar to that like Halloween, 31/10/2017. Use split () the command to chop this string into two parts. The parts before and after the comma will be separate from the items that you can save in a list current_event
for line in file:
line = line.rstrip('n')
current_event = line.split(',')
Using datetime
The Halloween event is kept in current_event as a list contents two items: “Halloween” and “31/10/2017”. Use the datetime module to convert the second item in the list (instead of 1) from a string into to form that Python can understand as date.
current_event = line.split(',')
event_date = datetime.strptime(current_event[1], '%d/%m/%y').date()
current_event[1] = event_date
Add the event to the list
Now the current_event list holds two things: the name of the event (as a string) and the date of the event. Add current_event to the list of events. Here’s the whole code for the get_events() function.
def get_events():
list_events = []
with open('events.txt') as file:
for line in file:
line = line.rstrip('n')
current_event = line.split(',')
event_date = datetime.strptime(current_event[1], '%d/%m/%y').date()
current_event[1] = event_date
list_events.append(current_event)
return list_events
Count the days
Create a function to count the a number of days between two dates. The datetime module makes this easy. Because it can add dates together or subtract one from another. Type the code shown here below your get_events() function. It will store the number of days as a string in the variable time_between.
def days_between_dates(date1, date2):
time_between = str(date1—date2)
Split the string
If Halloween is 27 days away, the string stored in time_between would look like this: ’27 days, 0:00:00′ (the zeros refer to hours, minutes, and seconds). Only the number at the beginning of the string is important. So you can use the split() command again to get to the part you need. Type the code highlighted below after the code in Step 14. It turns the string into a list of three items: ’27’, ‘days’, ‘0:00:00’. The list is stored in number_of_days.
def days_between_dates(date1, date2):
time_between = str(date1—date2)
number_of_days = time_between.split(' ')
Return the number of days
To finish off this function, you just need to return the value stored in position 0 of the list. In the case of Halloween, that’s 27. Add this line of code to the end of the function.
def days_between_dates(date1, date2):
time_between = str(date1—date2)
number_of_days = time_between.split(' ')
return number_of_days[0]
Get the events
Now that you’ve written all the functions, you can use them to write the main part of the program. Put these two lines at the bottom of your file. The first line calls (runs) the get_events() function and stores the list of calendar events in a variable called events. The second line uses the datetime module to get today’s date and stores it in a variable called today.
c.create_text(100, 50, anchor='w', fill='orange',
font='Arial 28 bold underline', text='My Countdown Calendar')
events = get_events()
today = date.today()
Display the results
Next calculate the number of days until each event and display the results on the screen. You need to do this for every event in the list, so use a for loop. For each event in the list, call the days_between_dates() function and store the result in a variable called days_until. Then use the Tkinter create_text() function to display the result on the screen.
for event in events:
event_name = event[0]
days_until = days_between_dates(event[1], today)
display = 'It is %s days until %s' % (days_until, event_name)
c.create_text(100, 100, anchor='w', fill='lightblue',
font='Arial 28 bold', text=display
Spread it out
The problem is that all the text is displayed at the same location (100, 100). If we create a variable called vertical_space and increase its value every time the program goes through the for loop, it will increase the value of the y coordinate and space out the text further down the screen. That’ll solve it!
vertical_space = 100
for event in events:
event_name = event[0]
days_until = days_between_dates(event[1], today)
display = 'It is %s days until %s' % (days_until, event_name)
c.create_text(100, vertical_space, anchor='w', fill='lightblue',
font='Arial 28 bold', text=display)
vertical_space = vertical_space + 30
full source code Countdown Calendar using python tkinter:
https://github.com/patelrohan750/Countdown-Calendar-using-Python-Tkinter-