A linear search in python is a very simple search algorithm. In this type of search. You search sequentially for each item. Everything in check. And when a match founded. The item returns. Otherwise, the search continues until the end of data collection. A linear search is easy to implement in python or other languages.
In Linear search in python. Compares the search item with all items available in the array and. When the item is successfully matching. It returns the index of the element in the array. Otherwise returns the element not found.
Note: Linear Search applies to unsorted or unordered lists.When there are fewer elements in a list.
How Linear search Works?
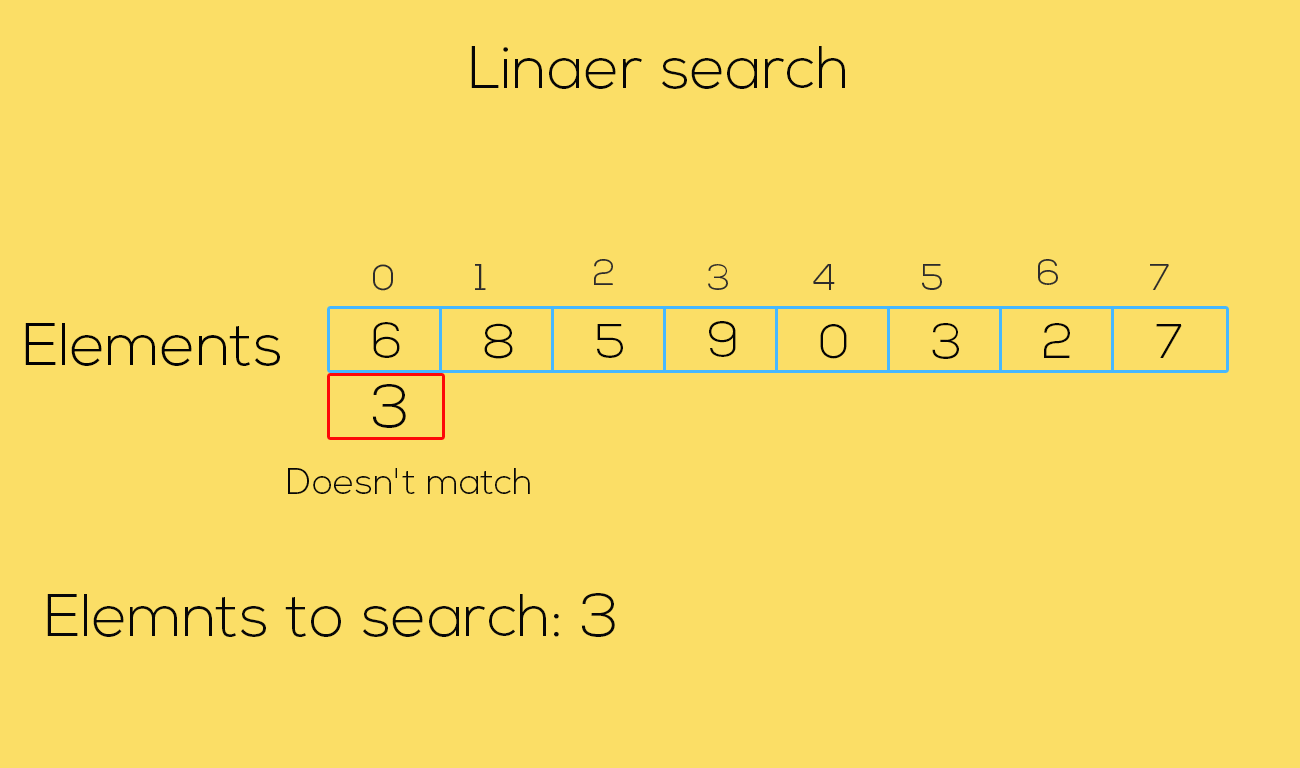
If you want to determine the positions of the occurrence of the number 3 in this array. To determine the positions, every element in the array from start to end. Example from index 0 to index 7 will compare with the number 3. To check which element matches the number 3.
Features of Linear Search
1.It is use for an unsorted and unordered small list of elements.
2.Linear search time complexity in best case O(1), in average case O(n), and in worst case O(n)
3.its Space Complexity is O(1)
4.it has a very simple implementation.
Implementing Linear Search in python
Following are the steps of implementation that we will be following:
1.Traverse the array using a for loop.
2.In every step, compare the target value with the current value of the array.
- If the values are the same, return the current index of the array.
- If values do not match, move on to the next array item.
3.If no match is found, return element not found.
def linearSearch(arr,item):
arr1=[]
flag=False
for i in range(len(arr)):
if arr[i]==item:
flag=True
arr1.append(i)
if flag==True:
for i in arr1:
print(f"{item} Found in Index {i}")
else:
print("element not found")
arr=[6,8,5,5,0,3,2,7]
print(arr)
item=int(input("Enter Element You Want To Search: "))
linearSearch(arr,item)


We know you like Linear search. Because it is so damn simple to implement. but it is not used practically because binary search is a lot faster than linear search.
Full source code:
https://github.com/patelrohan750/Algorithms-in-python/tree/main/linear%20search%20in%20python