Variable means anything that can vary. In JavaScript, variables hold the data value and it can be changed anytime.
JavaScript uses reserved keyword var to declare a variable. A variable must have a unique name. You can assign a value to a variable using equal to (=) operator when you declare it or before using it.
Syntax :
var variable-name;
var variable-name = value;
Example: Variable Declaration
var myName="patel"; //variable stores string value
var myAge=22; // variable stores number value
var city; //declared a variable without assigning a value
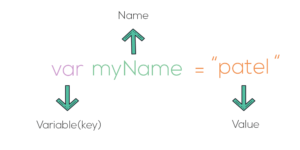
Naming Variables Rules:
1)The first character must be a letter or an underscore(_) or a dollar($). You can’t use a number as the first character
Example:
var myName="patel"; //valid
var _myName="rocoderes"; //valid
var $myAge=21; //valid
var 1myname=”Beast”; //not valid
2)The rest of the variable name can include any letter, any number, or the underscore. can’t use any other character, including spaces.
Example:
var _myAge1=22; //valid
var my Age=23; //not valid
3)Variable names are case sensitive
Example:
var myAge=22;
console.log(myage); //its show ReferenceError: myage is not defined
4)no limit to the length of the variable name.
5)you can’t use one of the javascript reserved words as a variable name.
Example:
var var=22; //not valid
Challenge Time
var _myName="patel";
var _1my__name="ro";
var 1myname="coderes";
var $myName="Rocoderes";
try it yourself whether it is valid or not