In this article, we are going to look at passing a JavaScript value between HTML pages. So basically we will have two HTML pages, and we will move some or information from one page to another using some basic methods. So this thing will be very much helpful when you try to create websites where you need to pass the data from one page to another. We will see this with very basic example and explanation.
Techniques For Passing a JavaScript Value Between HTML Pages
Okay, first, let’s see some different techniques for passing a JavaScript value between HTML pages:
- Local Storage
- URL query string
- IndexedDB
- Single page application(SPA)
So these are basic methods which are helpful in doing this specific task. We are going to look at two of these methods: local storage and URL query string. Because these techniques are pretty good and straight forward, also IndexedDB is pretty similar to local storage, but it is complicated to deal with. Single page applications are none other than AngularJS and ReactJS which makes this task a lot simple than other techniques, and also it is not that topic which we can cover in this single article.
Passing a JavaScript Value Between HTML Pages: Local Storage
The first basic technique is local storage, which is actually one of the basic way to do. Local storage method is already given by JavaScript itself. Now let’s see a basic example to understand in deep, here we will try to pass time to second page from first page.
So here we create two HTML pages and two JS files associated to them. In the first HTML file, we just added a heading and a button. Then in app.js file, we have created an object for Date(), and then we added a click event on the button where we have accessed localStorage() method.
So that we can use it and store date in that using localStorage.setItem()
. Then we used this window.document.location = './index2.html';
to set a targeted location where we need to access this data. Lastly, we have to run this function when this page gets loaded using document.addEventListener('DOMContentLoaded', function(){ init();})
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div class="content">
<h1>Click The Button To Go On Next Page</h1>
<button id="item1">Click</button>
</div>
<script src="app.js"></script>
</body>
</html>
const init = (e) =>{
let btn = document.querySelector("#item1");
let startTime = new Date();
btn.addEventListener('click', function(){
localStorage.setItem('start-time', startTime.getTime());
window.document.location = './index2.html';
});
};
document.addEventListener('DOMContentLoaded', function(){
init();
});
Now we have added some basic headings, and also we will add a span with id so that we can put the data in it using its ID. Then in app2.js script, we will access that data using this localStorage.getItem('start-time')
method. Now we have created another object of Date() and we are just subtracting the both time values. And we have added a function call when our second page gets loaded.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div class="content">
<h1>Next Page</h1>
<h2>Here's The Amount of Time Passed: <span id="time">TIME</span></h2>
</div>
<script src="app2.js"></script>
</body>
</html>
const init = (e) =>{
let spn = document.querySelector("#time");
let endTime = new Date();
let elapsedTime = endTime.getTime()- localStorage.getItem('start-time');
spn.innerHTML = elapsedTime;
}
document.addEventListener("DOMContentLoaded", function(){
init();
})
Passing a JavaScript Value Between HTML Pages: URL Query String
Now let’s see another technique to do this same task is by using URL Query String. In app.js file, we have again created an object of Date(), and we added the same click event on this button. In this, we have used window.document.location
, where we specified a targeted HTML file where we will access this data. Also, we have added '?startTime=' +startTime.getTime()
so that this string will be passed as URL along with time.
const init = (e) =>{
let btn = document.querySelector("#item1");
let startTime = new Date();
btn.addEventListener('click', function(){
window.document.location = './index2.html' + '?startTime=' + startTime.getTime();
});
};
document.addEventListener('DOMContentLoaded', function(){
init();
});
Now in app2.js file we have created another date object from which we will subtract startTime
. Then we will fetch the time from the URL string using window.location.search()
method. And from this we just need to get the time or simply data, for that, we will apply to replace method on this URL string. And we have added a regular expression, so that we can get URL string except data, and we have replaced it with blank string.
Finally, we will just subtract data from the current time. Okay, so this method should be used to pass little amount of data because the data we are passing will be reflected in to the URL.
"use strict"
const init = (e) =>{
let spn = document.querySelector("#time");
let endTime = new Date();
console.log(endTime);
let startTime = window.location.search.replace(/^.*?\=/,'');
spn.innerHTML = endTime.getTime() - startTime;
}
document.addEventListener("DOMContentLoaded", function(){
init();
})
Output
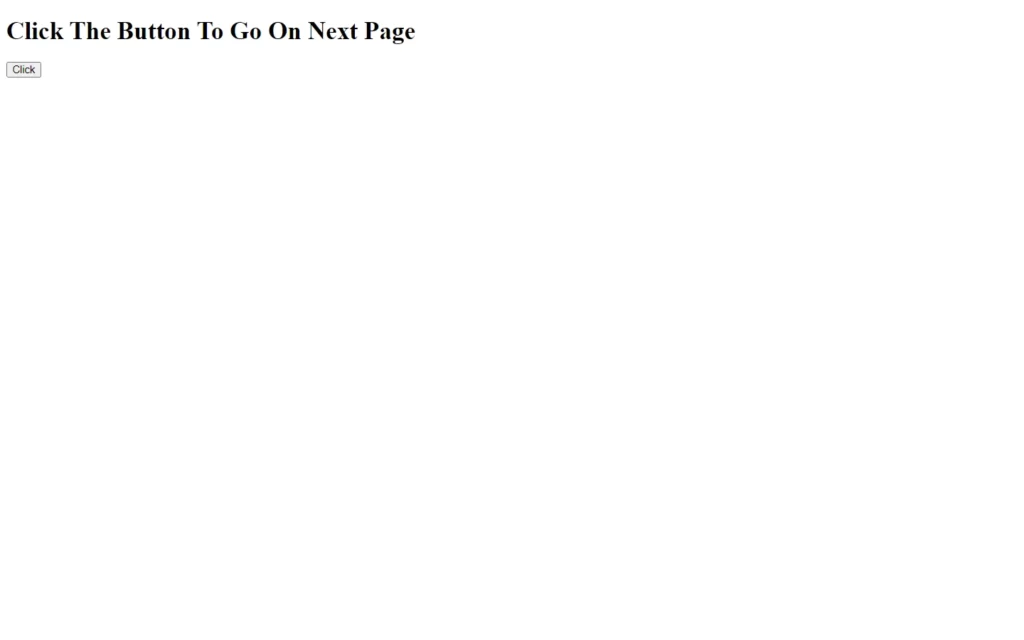
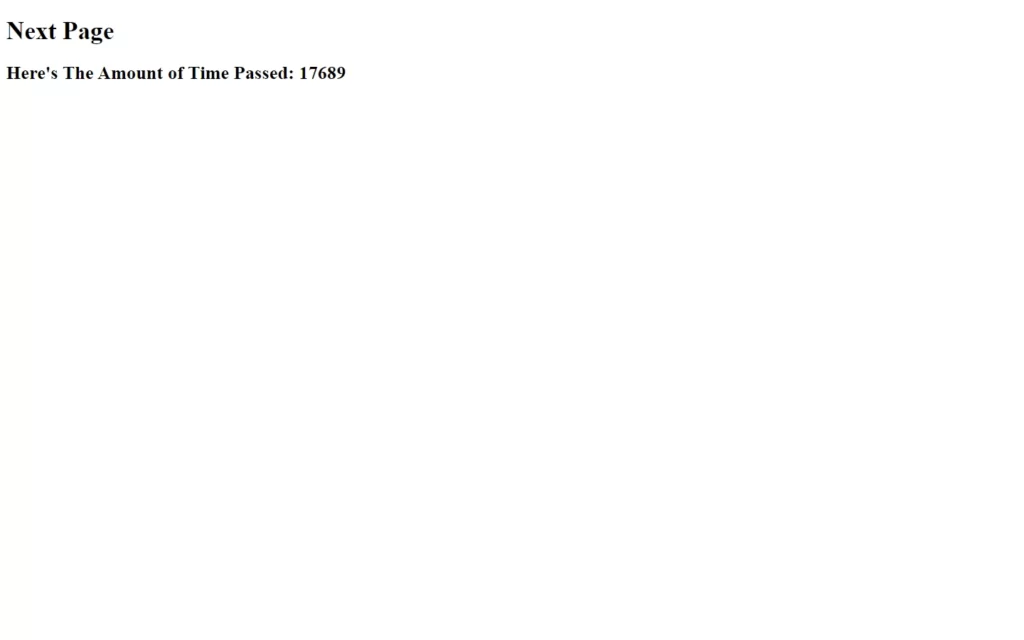