In this article, we are going to see the Extracting Numbers from a String in JavaScript. As we know, extracting a number from the given string will be easier when we apply some methods like parseInt() and parseFloat() methods, right? But it also has some limitation which we are going to see in here, and also we will see some different ways to extract numbers from a string.
Extracting Numbers From a String Using Traditional Methods
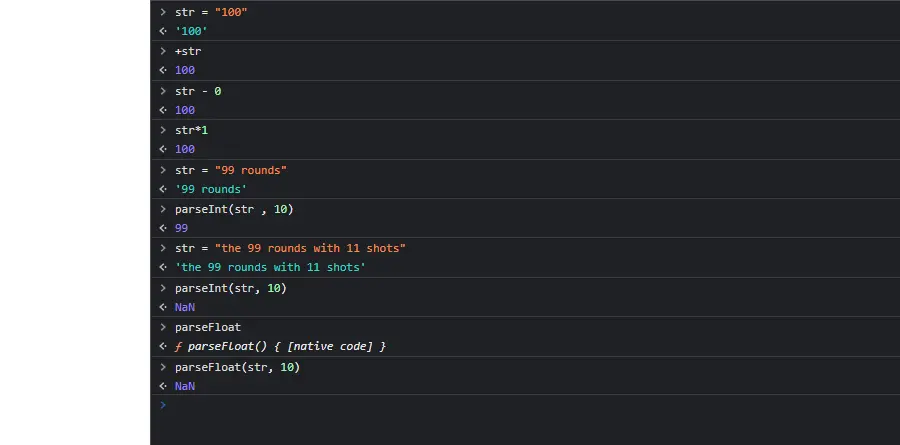
As we can see, in the above simple example, first we have added a number as a string which can be extracted in multiple ways like using mathematical operations, or we can simply use parseInt() and parseFloat() methods. Which is actually simple and straight-forward when string is only a number.
Next, we have added a number with a string behind it, this number can also get extracted with the help of parseInt() and parseFloat() method since string is “99 rounds”. Here, number is in starting of the string, so extraction of number will be performed with these two methods.
Now we have a problematic string which is numbers within strings, here we have “the 99 rounds with 11 shots”, so extracting numbers from this string won’t work. So basically, parseInt() and parseFloat() methods are not able to extract the number from the string.
So we are going to solve this type of problem where numbers are given between strings.
Extracting Numbers From a String: Solution
Let’s have a basic solution to extract number is to use isNaN() method which returns a boolean result to check where a string is number or not. Here we have a string “the 99 rounds with 11 shots”, firstly we have split the string with a space(“ “), so that array will be created like [“the”, “99”, “rounds”, “with”, “11”, “shots”].
Now we have applied filter() method on this array, in which are just returning those strings which are numbers, to do this we have a method !isNaN() which checks where the element is number or not. After this, we have another array will be build named nums who has [“99”, “11”].
As we can see, the resultant array has numbers, but they are in string form, so we need to convert these strings into the number. For that, we have applied map() method on the array, and we applied parseInt() method to convert string to number.
var str = "the 99 rounds with 11 shots";
var arr = str.split(" ");
let nums = arr.filter((elem)=>{
return !isNaN(elem);
})
let final_arr = nums.map((ele)=>{
return parseInt(ele);
})
console.log(final_arr);
console.log(typeof(final_arr[0]));
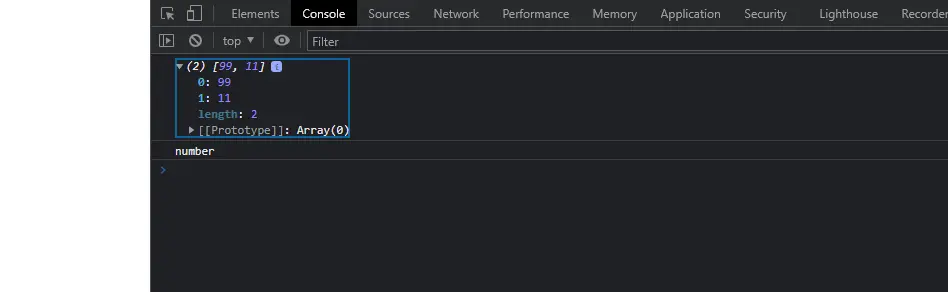
Extracting Numbers From a String: Solution (Alternatives)
As we know, JavaScript is the flexible language, so that we can have multiple solutions for the one problem. So here are some alternatives to Extracting Numbers From a String:
Alternative 1:
let str = "the 99 rounds with 11 shots";
const result = str.split(' ').reduce((acc, cur) => {
if (!isNaN(cur)) acc.push(+cur);
return acc;
}, []);
console.log(result);
Alternative 2:
let str = "the 99 rounds with 11 shots";
const result = str.match(/\d+/g).map(Number);
console.log(result)
Alternative 3:
var str = "the 99 rounds with 11 shots";
let final = str.split(' ').filter(function(n) {
return !isNaN(n)
}).map(Number)
console.log(final);