Introduction to JavaScript array
In javascript array, the term “array” refers to an array of items or elements. They store data as elements and can be retrieved when you require them. In JavaScript, the term “array” refers to an ordered listing of values. Each value is known as an element that is specified through an index.
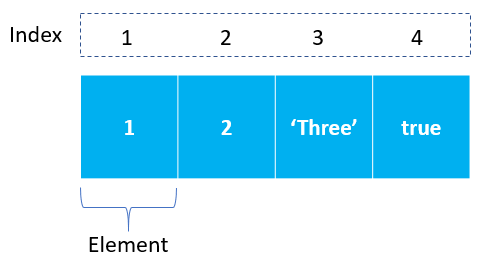
The square bracket [] represents an array using JavaScript. The elements of the array are separated by commas(,).
In JavaScript, arrays can be a collection of elements of all types. This means you can make an array using elements of type String Boolean Number, Objects, as well as other arrays.
Here’s an example of an array that has the following elements: Type Number, Boolean, String, and Object.
const mixedTypedArray = [200, true, 'javascript', {}];
The position of an element in an array is identified as its index. The array index begins with zero in JavaScript and then increases by one for every element.
So, for example, in the above array, the element 200 is at index 0, true is at index 1, ‘javascript’ is at index 2, and so on.
In other words, the number of elements contained in the array determines the array’s length. For instance, this array’s length is 4.
Incredibly, JavaScript arrays do not have an exact length. They can be changed in length by assigning an appropriate numeric value. We’ll get more information about that later.
Creating JavaScript arrays
JavaScript provides you with two ways to create an array. The first one is to use the Array constructor as follows:
For example, the following creates the scores
array that has five elements (or numbers):
const scores = new Array(9,10,8,7,6);
Note that if you use the array () constructor to create an array and pass a number into it, you create an array with an initial size.
A second way to create an array is by assigning an array value to a variable.
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
Accessing JavaScript array elements
You can retrieve and access the elements of an array by using its index. It is necessary to use the square bracket syntax for accessing array elements.
const element = array[index];
You can access array elements one by one or in a loop based on your needs.
When you’re accessing the elements using an index like this:
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
shopping[0] //bread
shopping[1] //milk
shopping[2] //cheese
shopping[3] //hummus
shopping[4] //noodles
You can use the length of an array to traverse backward and access elements.
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
const len = shopping.length;
shopping[len - 1]; // 'noodles'
shopping[len - 4]; // 'milk'
You can also loop through the array using a regular for
or forEach
loop, or any other loop.
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
for(let i=0; i<shopping.length; i++) {
console.log(`Element at index ${i} is ${shopping[i]}`);
}
And here’s the output:
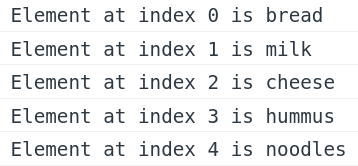
JavaScript Array Basic operations
How to Add Elements to the end of an array
Use the push() method to add an element to an array. The push() method adds an element at the end of the array.
const shopping = ['bread', 'milk', 'cheese', 'hummus'];
shopping.push("noodles");
console.log(shopping);
Now The shopping array is:
['bread', 'milk', 'cheese', 'hummus', 'noodles'];
Note that the push() method adds an element to the end of the array.
How to Add Elements to the beginning of an array
If You want to add an element to the beginning of an array, you use the unshift() method:
const shopping = ['bread', 'milk', 'cheese', 'hummus'];
shopping.unshift("noodles");
console.log(shopping);
Now The shopping array is:
['noodles','bread', 'milk', 'cheese', 'hummus'];
How to Remove Elements from the end of an array
The easiest way to remove a single element from an array is using the pop() method. Every time you call the pop() method, it removes an element from the end of the array. Then it returns the removed element and changes the original array. To save that item in a new variable, you could do this:
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
let removeElement = shopping.pop();
console.log(removeElement) // 'noodles'
console.log(shopping) // ['bread', 'milk', 'cheese', 'hummus']
How to Remove Elements from the beginning of an array
Use the shift() method to remove an element from the beginning of an array. shift() returns the removed element and changes the original array and you can also save that item in new variable.
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
let removeElement = shopping.shift();
console.log(removeElement) // 'bread'
console.log(shopping) // ['milk', 'cheese', 'hummus', 'noodles']
How to Copy and Clone an Array
The slice() method returns a shallow copy of a portion of an array into a new array object. Note that the slice() method doesn’t change the original array.
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
const copyArray = shopping.slice();
console.log(copyArray) // ['bread', 'milk', 'cheese', 'hummus', 'noodles']
slice() Parameters
The slice(start,end) method takes in:
- start (optional) – Starting index of the selection. If not provided, the selection starts at start 0.
- end (optional) – Ending index of the selection (exclusive). If not provided, the selection ends at the index of the last element.
let languages = ["JavaScript", "Python", "C", "C++", "Java"];
// slicing the array (from start to end)
let new_arr = languages.slice();
console.log(new_arr); // [ 'JavaScript', 'Python', 'C', 'C++', 'Java' ]
// slicing from the third element
let new_arr1 = languages.slice(2);
console.log(new_arr1); // [ 'C', 'C++', 'Java' ]
// slicing from the second element to fourth element
let new_arr2 = languages.slice(1, 4);
console.log(new_arr2); // [ 'Python', 'C', 'C++' ]
Output:
[ 'JavaScript', 'Python', 'C', 'C++', 'Java' ]
[ 'C', 'C++', 'Java' ]
[ 'Python', 'C', 'C++' ]
How to check if an value is an array
To check if a value is an array, you use array.isArray() method. The method returns true if the passed value is an array.
const shopping = ['bread', 'milk', 'cheese', 'hummus', 'noodles'];
console.log(Array.isArray(shopping)); // true
console.log(Array.isArray('Mango')); // false
console.log(Array.isArray([])); // true
Array Destructuring in JavaScript
Destructuring means breaking down a complex structure into simpler parts. With destructuring syntax, you can extract smaller fragments from objects and arrays. It can be used for assignments and declaration of a variable.
let [tomato, mushroom, carrot] = ['🍅', '🍄', '🥕'];
console.log(tomato, mushroom, carrot); // Output:, 🍅 🍄 🥕
So, the destructuring syntax saves you from writing lots of code. This gives you a massive boost in productivity.
How to Use the Rest Parameter in JS
JavaScript Rest Parameters provide us with a way to declare a function that can take an indefinite number of arguments available as an array. In simple language, we can define the JavaScript function with Rest Parameters, which doesn’t have a fixed number of parameters and can take any number of arguments when called.
For example, if I want to write a generic sum function, which can be used to add any number of values, which means it should work for sum(1, 2) function call, sum(1,2,3) function call, sum(1,2,3,4) function calls, etc.
The rest operator converts the arguments to an array so that you can iterate through them easily. This is evident from the following example.
function sum(...args){
console.log(args);
}
sum(1, 2); //[1, 2]
sum(4, 5, 6); //[4, 5, 6]
You can see that the arguments 1 and 2 are transformed into an array. Similarly, 4, 5 and 6 are also transformed into the array.
The rest parameter must be the last parameter of the function. If you don’t follow this, you will get SyntaxError.
The following code will give SyntaxError: The rest parameter must be the last formal parameter.
function display(arg1, ...args, arg3){
//function body
}
The correct way of writing the code is
function display(arg1, arg2, ...args){
console.log(arg1);
console.log(arg2);
console.log(args);
}
display(2, 3, 4, 5, 6);
Output:
2
3
[4, 5, 6]
4, 5 and 6 has become array elements because of the rest parameter args.
How to Use the Spread Operator in JS
The spread operator spreads out an iterable such as an array, string, map, or set into individual elements. The spread operator consists of three dots(…) followed by an iterable.
It looks like has the same as the Rest parameter operator.In fact, it perform almost the opposite function to Rest operator. Spread operator turns an array to comma-separated values.
Below example spread array1 to a comma-separated list of values into the array2
var array1 = [2, 3];
var array2 = [1, ...array1, 4, 5]; // spread
// array2 = [1, 2, 3, 4, 5]
Concat array Using Spread Operator
const arr1 = ['coffee', 'tea', 'milk']
const arr2 = ['juice', 'smoothie']
// Without spread
var beverages = arr1.concat(arr2)
// With spread
var beverages = [...arr1, ...arr2]
// result
// ['coffee', 'tea', 'milk', 'juice', 'smoothie']
Make copy of array Using spread Operator
const arr1 = ['coffee', 'tea', 'milk']
// With spread
const arr1Copy = [...arr1]
How to convert a string to an array of individual characters?
When you use a spread operator with a string, it converts that string into an array of characters. The spread operator does not modify the original string.
let str = "JavaScript";
let arr = [...str];
console.log(arr); //["J", "a", "v", "a", "S", "c", "r", "i", "p", "t"]
JavaScript Array Methods
So far, we have seen a few array properties and methods. Let’s do a quick recap of the ones we’ve looked at:
push()
– Insert an element at the end of the array.unshift()
– Insert an element at the beginning of the array.pop()
– Remove an element from the end of the array.shift()
– Remove an element from the beginning of the array.slice()
– Create a shallow copy of an array.Array.isArray()
– Determine if a value is an array.length
– Determine the size of an array.
Now we’ll learn about other important JS array methods with examples.
The concat() array method
If you want to merge two or more arrays into a single array, use the JavaScript array concat() method.
The concat() method does not modify the existing arrays and returns the new array, which is a merger of two or more arrays.
You can pass one or more arrays to the concat() method.
const first = [1, 2, 3];
const second = [4, 5, 6];
const merged = first.concat(second);
console.log(merged); // [1, 2, 3, 4, 5, 6]
console.log(first); // [1, 2, 3]
console.log(second); // [4, 5, 6]
The join() array method
The join() method returns a new string by concatenating all elements in an array, separated by a specified separator.
let message = ["JavaScript", "is", "fun."];
// join all elements of array using space
let joinedMessage = message.join(" ");
console.log(joinedMessage);
// Output: JavaScript is fun.
The fill() array method
The fill() method fills an array with a static value. You can change all the elements to a static value or change a few selected items. Note that the fill() method changes the original array.
const colors = ['red', 'blue', 'green'];
colors.fill('pink');
console.log(colors); // ["pink", "pink", "pink"]
Here is an example where we are changing only the last two elements of the array using the fill() method:
const colors = ['red', 'blue', 'green'];
colors.fill('pink', 1,3); // ["red", "pink", "pink"]
In this case, the first argument of the fill() method is the value we change with. The second argument is the start index to change. It starts with 0. The last argument is to determine where to stop filling. The max value of it could be colors.length.
The includes() array method
The includes() method checks if an array contains a specified element or not. If the element is found, the method returns true and false otherwise.
// defining an array
let languages = ["JavaScript", "Java", "C"];
// checking whether the array contains 'Java'
let check = languages.includes("Java");
console.log(check);
// Output: true
The indexOf() array method
You may want to know the index position of an element in an array. You can use the indexOf() method to get that. It returns the index of the first occurrence of an element in the array. If an element is not found, the indexOf() method returns -1.
const fruits = ['apple', 'orange', 'banana', 'apple', 'pear'];
console.log(fruits.indexOf('apple'));
// output: 0
The JavaScript array lastIndexOf() method is used to search the position of a particular element in a given array. It behaves similar to indexOf() method with a difference that it start searching an element from the last position of an array. The lastIndexOf() method is case-sensitive.
const fruits = ['apple', 'orange', 'banana', 'apple', 'pear'];
console.log(fruits.lastIndexOf('apple'));
//output:4
The reverse() array method
As the name suggests, this method reverses the order of array elements by modifying the existing array.
const names = ['tom', 'alex', 'bob'];
names.reverse(); // returns ["bob", "alex", "tom"]
The sort() array method
The sort() method sorts the items of an array in a specific order (ascending or descending).
let city = ["California", "Barcelona", "Paris", "Kathmandu"];
// sort the city array in ascending order
let sortedArray = city.sort();
console.log(sortedArray);
// Output: [ 'Barcelona', 'California', 'Kathmandu', 'Paris' ]
The splice() array method
The JavaScript splice() method changes the elements of the array by replacing or removing the elements.
Syntax of splice() method
- Removed: The array that stores all the removed elements that the splice() method returns
- Array: The array on which the splice() method is being applied
- Splice: Function call to the method
- Index: Starting index for the splice() method
- Count: Specifies the number of items in the array to replace/remove from the starting index
- Items: Items that replace the array elements from the starting index
In the example below, we add an element zack at index 1 without deleting any elements.
const names = ['tom', 'alex', 'bob'];
names.splice(1, 0, 'zack');
console.log(names); // ["tom", "zack", "alex", "bob"]
Have a look at the following example. Here we remove one element from index 2 (the 3rd element) and add a new element, zack. The splice() method returns an array with the deleted element, bob.
const names = ['tom', 'alex', 'bob'];
const deleted = names.splice(2, 1, 'zack');
console.log(deleted); // ["bob"]
console.log(names); // ["tom", "alex", "zack"]
The find() array method
The find() method returns the first matched element from the array that satisfies the condition in the function.
syntax is: array.find(function(element, index, array),thisValue)
const prices = [200, 300, 350, 400, 450, 500, 600];
const findElem = prices.find((element, index, array) => {
return element < 400
})
console.log(findElem) // 200
The map() array method
The map() method creates a new array with the results of calling a provided function on every element in the calling array.
map calls a provided callback function once for each element in an array. in order, and returns a new array from the results.
Note: this method does not change the original array.
syntax: Array.map(function (currentValue, index, arr), thisValue)
const array1 = [1, 4, 9, 16, 25];
array1.map((curElem, index, arr) => {
console.log(`element ${curElem} at ${index} index`);
})
//output:
// element 1 at 0 index
// element 4 at 1 index
// element 9 at 2 index
// element 16 at 3 index
// element 25 at 4 index
Example: 2
const array = [1, 4, 9, 16, 25];
let newArr = array.map((curElem, index, arr) => {
return curElem*2;
})
console.log("original array: ",array);
console.log("After apply map method: ",newArr);
/*
------output------
original array: [ 1, 4, 9, 16, 25 ]
After apply map method: [ 2, 8, 18,32, 50 ]
*/
The reduce() array method
The reduce() method executes a reducer function (that you provide) on each element of the array, resulting in a single output value.
The result is called an accumulator.
Here initial value is optional. It’s the initial value of the accumulator. if it’s not provided it’s the default value of the array first element.
const numbers=[2,3,1,4,5,6]
const sum=numbers.reduce((accumulaotr,currentValue)=>{
return accumulaotr+currentValue
})
console.log(sum)//output: 21
The filter() array method
This method returns a subset of the given array. It executes the given function for each item in the array. Whether the function returns true or false, it keeps that element in or filters it out. If true, the element is kept in the result array. If false, the element is excluded from the result array.
Note: filter() method does not change the original array.
function isGreaterThanTwo(item){
return item>2
}
const prices = [200, 300, 350, 400, 450, 500, 600];
const priceTag = prices.filter((currVal, index, array) => {
return currVal < 400
})
console.log(priceTag)//output: [ 200, 300, 350 ]
In the above example callback function check if the value of the given currVal is less than 400. the result is a new array with only [ 200, 300, 350 ].
👉 more info about javascript basic concepts – map(), filter() and reduce() in JavaScript
👉 If You want to learn more about javascript array then watch this video